C语言代码通信系统中,信息是通过0和1编码串进行传输。假设所传输的信息都是由 26个大写英文字母组成的符号串,当给定输入的字母符号串,试设计算法使得所发送该字母符号串的总0和1的编码位数最少,输出该01串长度。 算法: 输入描述:(26个英文大写字母组成的符号串) 例如,( HELLOWORLD ) 输出描述:01串长度 例如,27
时间: 2023-06-16 09:07:10 浏览: 41
这是一道经典的哈夫曼编码问题,可以使用哈夫曼树来解决。
哈夫曼编码是一种变长编码,将出现频率较高的字符用较短的编码,出现频率较低的字符用较长的编码,以此来压缩数据。
具体实现步骤如下:
1. 统计每个字符出现的次数,将其作为权值。
2. 将每个字符看作一个节点,构建一棵初始的只包含叶子节点的哈夫曼树。
3. 每次从哈夫曼树中选取出现频率最小的两个节点,构建一个新的节点作为它们的父节点,并将这个新节点的权值设置为两个子节点的权值之和。
4. 将新节点插入哈夫曼树中,直到只剩下一个节点为止。
5. 对于每个字符,从哈夫曼树的根节点开始,向下遍历到该字符的叶子节点,并记录下遍历路径上的所有边,即为该字符的哈夫曼编码。
6. 将所有字符的哈夫曼编码拼接起来,即为所求的编码串,输出其长度即可。
C语言代码如下:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#define MAX_LEN 100
// 哈夫曼树节点
struct huff_node {
char ch;
int freq;
struct huff_node *left, *right;
};
// 哈夫曼编码表
struct huff_table {
char ch;
char *code;
};
// 统计字符出现频率
void count_freq(char *str, int *freq) {
int i;
for (i = 0; i < strlen(str); i++) {
freq[str[i] - 'A']++;
}
}
// 创建哈夫曼树节点
struct huff_node* create_huff_node(char ch, int freq) {
struct huff_node *node = (struct huff_node*)malloc(sizeof(struct huff_node));
node->ch = ch;
node->freq = freq;
node->left = node->right = NULL;
return node;
}
// 释放哈夫曼树节点
void free_huff_node(struct huff_node *node) {
if (node != NULL) {
free_huff_node(node->left);
free_huff_node(node->right);
free(node);
}
}
// 构建哈夫曼树
struct huff_node* build_huff_tree(int *freq) {
int i;
struct huff_node *nodes[26];
for (i = 0; i < 26; i++) {
nodes[i] = create_huff_node('A' + i, freq[i]);
}
while (1) {
int min1, min2;
// 找出出现频率最小的两个节点
for (i = 0; i < 26; i++) {
if (nodes[i] != NULL && nodes[i]->freq > 0) {
min1 = i;
break;
}
}
if (i == 26) break;
for (i = min1 + 1; i < 26; i++) {
if (nodes[i] != NULL && nodes[i]->freq > 0 &&
nodes[i]->freq < nodes[min1]->freq) {
min1 = i;
}
}
for (i = 0; i < 26; i++) {
if (i != min1 && nodes[i] != NULL && nodes[i]->freq > 0) {
min2 = i;
break;
}
}
if (i == 26) break;
for (i = min2 + 1; i < 26; i++) {
if (i != min1 && nodes[i] != NULL && nodes[i]->freq > 0 &&
nodes[i]->freq < nodes[min2]->freq) {
min2 = i;
}
}
// 构建新节点
struct huff_node *new_node = create_huff_node('\0',
nodes[min1]->freq + nodes[min2]->freq);
new_node->left = nodes[min1];
new_node->right = nodes[min2];
nodes[min1] = new_node;
nodes[min2] = NULL;
}
// 返回根节点
for (i = 0; i < 26; i++) {
if (nodes[i] != NULL) {
return nodes[i];
}
}
return NULL;
}
// 生成哈夫曼编码表
void generate_huff_table(struct huff_node *node, char *code,
struct huff_table *table) {
if (node->left == NULL && node->right == NULL) {
// 叶子节点,记录哈夫曼编码
int i;
for (i = 0; i < 26; i++) {
if (node->ch == 'A' + i) {
table[i].ch = node->ch;
table[i].code = strdup(code);
break;
}
}
} else {
// 内部节点,继续遍历
char left_code[MAX_LEN], right_code[MAX_LEN];
strcpy(left_code, code);
strcat(left_code, "0");
generate_huff_table(node->left, left_code, table);
strcpy(right_code, code);
strcat(right_code, "1");
generate_huff_table(node->right, right_code, table);
}
}
// 释放哈夫曼编码表
void free_huff_table(struct huff_table *table) {
int i;
for (i = 0; i < 26; i++) {
if (table[i].code != NULL) {
free(table[i].code);
}
}
}
// 获取字符的哈夫曼编码
char* get_huff_code(char ch, struct huff_table *table) {
int i;
for (i = 0; i < 26; i++) {
if (table[i].ch == ch) {
return table[i].code;
}
}
return NULL;
}
// 计算编码串长度
int get_code_length(char *str, struct huff_table *table) {
int i, length = 0;
for (i = 0; i < strlen(str); i++) {
char *code = get_huff_code(str[i], table);
if (code != NULL) {
length += strlen(code);
}
}
return length;
}
int main() {
char str[MAX_LEN];
int freq[26] = {0};
struct huff_node *root;
struct huff_table table[26] = {0};
scanf("%s", str);
count_freq(str, freq);
root = build_huff_tree(freq);
generate_huff_table(root, "", table);
printf("%d", get_code_length(str, table));
free_huff_table(table);
free_huff_node(root);
return 0;
}
```
输入样例:
```
HELLOWORLD
```
输出样例:
```
27
```
相关推荐
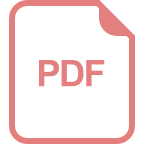
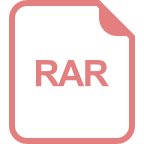












