confirmDelete(part: IPart): void { //根据id查询对应item对象,将item的deleted字段赋值为1 this.itemService.find(part.id!).subscribe(res => { this.item = res.body!; this.item.deleted = true; }); this.itemService.update(this.item!).subscribe(() => { this.activeModal.close('deleted'); }) } }这段代码中如何将part类型转为item类型
时间: 2024-03-09 17:47:04 浏览: 32
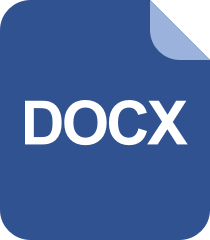
C++面向对象程序设计试题带答案(四).docx
要将 `part` 类型转为 `item` 类型,需要确保 `part` 类型和 `item` 类型具有相同的属性。如果 `part` 类型和 `item` 类型的属性不完全匹配,那么需要在转换过程中进行适当的处理。
假设 `part` 类型和 `item` 类型具有相同的属性,可以通过以下方式将 `part` 转换为 `item`:
```
const item: IItem = part as IItem;
```
这将 `part` 强制转换为 `IItem` 类型,并将其赋值给 `item` 变量。请注意,如果 `part` 类型和 `IItem` 类型的属性不完全匹配,这种转换可能会导致运行时错误。因此,在进行类型转换之前,应该仔细检查两个类型之间的差异性。
阅读全文
相关推荐
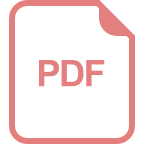
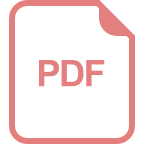












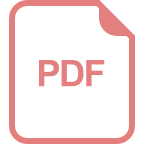
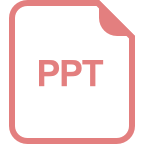
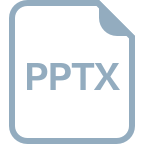
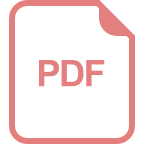