VBA如何循环多列
时间: 2023-07-04 08:19:20 浏览: 60
在 VBA 中循环多列可以使用 `For Each` 语句,以下是示例代码:
```vba
Sub LoopMultipleColumns()
Dim ws As Worksheet
Dim lastRow As Long
Dim col As Range
Set ws = ThisWorkbook.Worksheets("Sheet1") ' 更改为你的工作表名称
lastRow = ws.Cells(ws.Rows.Count, "A").End(xlUp).Row ' 获取最后一行
For Each col In ws.Range("A1:C1").Columns ' 循环 A、B、C 三列
For i = 2 To lastRow ' 从第二行开始循环每列
' 在这里进行你的操作,例如:
' Debug.Print ws.Cells(i, col.Column).Value
Next i
Next col
End Sub
```
这将循环 A、B、C 三列,并在每列中从第二行开始循环每个单元格。你可以在循环内对每个单元格进行你的操作。需要注意的是,`col.Column` 将返回当前列的列号,例如 A 列的列号为 1,B 列的列号为 2。
相关问题
VBA 文件合并成多列
你可以使用VBA来合并多个文件的数据到一个工作表的多列中。下面是一个简单的示例代码:
```vba
Sub 合并多个文件到多列()
Dim FolderPath As String
Dim FileName As String
Dim ws As Worksheet
Dim LastRow As Long
Dim i As Integer
' 设置文件夹路径
FolderPath = "你的文件夹路径"
' 设置要合并的文件类型
FileName = Dir(FolderPath & "\*.xlsx")
' 设置要合并到的工作表
Set ws = ThisWorkbook.Sheets("合并后的数据")
' 清空合并后的数据
ws.Cells.Clear
' 设置初始行数
LastRow = 1
' 循环遍历文件夹中的文件
Do While FileName <> ""
' 打开文件
Workbooks.Open (FolderPath & "\" & FileName)
' 将文件中的数据复制到合并后的工作表中
With Workbooks(FileName).Sheets(1)
.UsedRange.Copy ws.Cells(LastRow, 1)
LastRow = ws.Cells(ws.Rows.Count, 1).End(xlUp).Row + 1
End With
' 关闭文件
Workbooks(FileName).Close SaveChanges:=False
' 获取下一个文件名
FileName = Dir
Loop
' 调整列宽
ws.Columns.AutoFit
' 提示合并完成
MsgBox "文件合并完成!"
End Sub
```
在上面的代码中,你需要将`FolderPath`变量设置为包含要合并文件的文件夹的路径。然后,你需要将`ws`变量设置为要将数据合并到的工作表。代码将遍历文件夹中的所有Excel文件,将每个文件的数据复制到合并后的工作表的下一行。最后,它将自动调整列宽,并显示一个消息框提示合并完成。
请注意,这个示例假设要合并的所有文件都在同一个文件夹中,并且要合并的数据在每个文件的第一个工作表中。如果你的情况有所不同,可能需要对代码进行适当的修改。
VBA将多列数据转置为行数据脚本
可以使用下面的VBA脚本将多列数据转置为行数据:
```VBA
Sub TransposeData()
Dim inputRange As Range
Dim outputRange As Range
Dim i As Integer
Dim j As Integer
Dim rowCount As Integer
Dim colCount As Integer
Set inputRange = Application.InputBox("Select the range of data to transpose", Type:=8)
rowCount = inputRange.Rows.Count
colCount = inputRange.Columns.Count
Set outputRange = Application.InputBox("Select the cell where the transposed data should be placed", Type:=8)
For i = 1 To rowCount
For j = 1 To colCount
outputRange.Offset(j - 1, i - 1).Value = inputRange.Cells(i, j).Value
Next j
Next i
End Sub
```
运行此宏后,它将提示您选择要转置的数据范围和输出范围。然后将使用嵌套的循环将数据从列转置为行,并将其放置在指定的输出范围中。
相关推荐
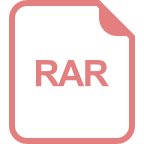
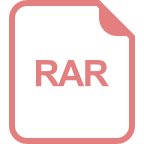
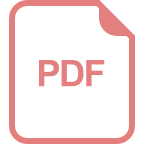












