用java写一个调用acmeshAPI自动部署证书到nginx的接口
时间: 2023-05-22 21:07:11 浏览: 234
好的,这是一个编程类问题,以下是代码示例:
```
import org.apache.http.client.fluent.Request;
public class DeployCert {
private final String ACME_API_URL = "https://acme-v02.api.letsencrypt.org/acme";
public void deploy(String domain, String certPath, String keyPath) throws Exception {
String certContent = readContentFromFile(certPath);
String keyContent = readContentFromFile(keyPath);
String privateKey = generatePrivateKey();
String csr = generateCSR(domain, privateKey);
String order = requestOrder(csr);
String authorization = getAuthorization(order);
String token = getToken(authorization);
String challengeResponse = getChallengeResponse(token);
String status = fulfillChallenge(authorization, challengeResponse);
if (status.equals("valid")) {
String cert = requestCert(order, csr);
uploadCert(domain, cert, privateKey);
}
}
private String readContentFromFile(String path) throws Exception {
return Files.readString(Paths.get(path));
}
private String generatePrivateKey() {
// TODO: implement
}
private String generateCSR(String domain, String privateKey) {
// TODO: implement
}
private String requestOrder(String csr) throws Exception {
String url = ACME_API_URL + "/order";
String payload = "{\"identifiers\":[{\"type\":\"dns\",\"value\":\"example.com\"}],\"notBefore\":\"2021-03-15T17:29:54Z\",\"notAfter\":\"2021-06-13T17:29:54Z\",\"csr\":\"" + csr + "\"}";
String response = Request.Post(url)
.bodyString(payload, ContentType.APPLICATION_JSON)
.execute()
.returnContent()
.asString();
return parseOrderUrl(response);
}
private String parseOrderUrl(String response) {
// TODO: implement
}
private String getAuthorization(String order) throws Exception {
String url = getOrderUrl(order) + "/authorizations";
String response = Request.Get(url)
.execute()
.returnContent()
.asString();
return parseAuthorizationUrl(response);
}
private String parseAuthorizationUrl(String response) {
// TODO: implement
}
private String getToken(String authorization) throws Exception {
String url = getAuthorizationUrl(authorization);
String response = Request.Get(url)
.execute()
.returnContent()
.asString();
return parseToken(response);
}
private String parseToken(String response) {
// TODO: implement
}
private String getChallengeResponse(String token) {
// TODO: implement
}
private String fulfillChallenge(String authorization, String challengeResponse) throws Exception {
String url = getAuthorizationUrl(authorization) + "/challenge";
String payload = "{\"keyAuthorization\":\"" + challengeResponse + "\"}";
String response = Request.Post(url)
.bodyString(payload, ContentType.APPLICATION_JSON)
.execute()
.returnContent()
.asString();
return parseChallengeStatus(response);
}
private String parseChallengeStatus(String response) {
// TODO: implement
}
private String requestCert(String order, String csr) throws Exception {
String url = getOrderUrl(order) + "/certificate";
String payload = "{\"csr\":\"" + csr + "\"}";
String response = Request.Post(url)
.bodyString(payload, ContentType.APPLICATION_JSON)
.execute()
.returnContent()
.asString();
return parseCert(response);
}
private String parseCert(String response) {
// TODO: implement
}
private void uploadCert(String domain, String cert, String privateKey) throws Exception {
// TODO: implement
}
private String getOrderUrl(String order) {
// TODO: implement
}
private String getAuthorizationUrl(String authorization) {
// TODO: implement
}
}
```
这个类提供了一个 deploy 方法,用于部署证书。你需要将 domain、certPath 和 keyPath 替换为你自己的值,然后调用 deploy 方法即可。注意,这个代码中有很多 TODO,你需要根据实际情况实现这些部分。这个类依赖于 Apache HttpComponents,你需要将它添加到你的项目中。
阅读全文
相关推荐
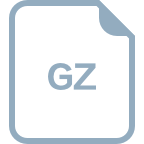
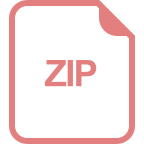
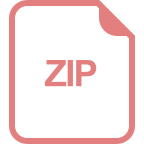
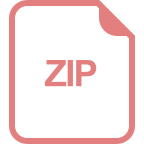
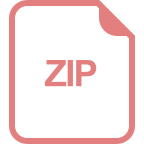
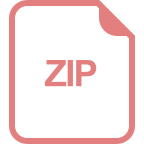
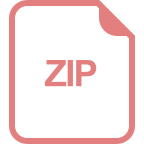
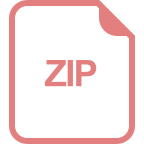
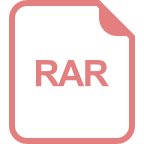
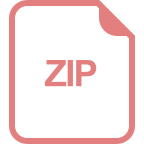
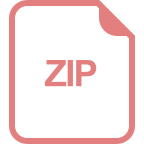
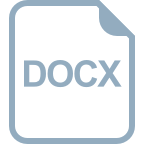