用python写一个树莓派控制霍尔编码电机的PID测速程序
时间: 2023-10-01 19:09:11 浏览: 180
下面是一个使用Python编写的树莓派控制霍尔编码电机的PID测速程序的示例代码,供参考:
```python
import RPi.GPIO as GPIO
import time
# 设置GPIO模式为BCM
GPIO.setmode(GPIO.BCM)
# 定义三个引脚的GPIO编号
encoder_pin1 = 17
encoder_pin2 = 18
motor_pin = 27
# 设置GPIO为输入模式
GPIO.setup(encoder_pin1, GPIO.IN)
GPIO.setup(encoder_pin2, GPIO.IN)
GPIO.setup(motor_pin, GPIO.OUT)
# 设置PID参数
Kp = 0.1
Ki = 0.01
Kd = 0.05
# 初始化PID误差
last_error = 0
integral = 0
# 定义PID计算函数
def pid_control(current_speed, target_speed):
global last_error, integral
error = target_speed - current_speed
integral += error
derivative = error - last_error
last_error = error
output = Kp * error + Ki * integral + Kd * derivative
return output
# 定义读取编码器函数
def read_encoder():
global encoder_counter
if GPIO.input(encoder_pin1) == GPIO.input(encoder_pin2):
encoder_counter += 1
else:
encoder_counter -= 1
# 定义电机控制函数
def motor_control(speed):
if speed >= 0:
GPIO.output(motor_pin, GPIO.HIGH)
else:
GPIO.output(motor_pin, GPIO.LOW)
pwm = GPIO.PWM(motor_pin, 1000)
pwm.start(abs(speed))
# 初始化编码器计数器和速度
encoder_counter = 0
last_encoder_counter = 0
current_speed = 0
# 定义目标速度
target_speed = 50
# 记录开始时间
start_time = time.time()
# 循环读取编码器和控制电机
while True:
read_encoder()
current_time = time.time() - start_time
if current_time >= 0.1:
current_encoder_counter = encoder_counter
current_speed = (current_encoder_counter - last_encoder_counter) / current_time
output_speed = pid_control(current_speed, target_speed)
motor_control(output_speed)
last_encoder_counter = current_encoder_counter
start_time = time.time()
```
注意,这只是一个示例代码,具体根据实际情况进行修改和优化。此外,还需要根据具体的硬件连接情况和编码器的工作方式进行相应的配置和调整。
相关推荐
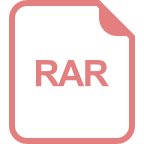
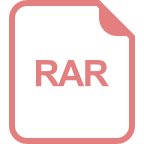
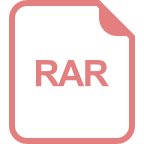














