请给我一个简单的网络并运用tensorboard进行可视化
时间: 2023-08-01 09:09:05 浏览: 77
好的,可以参考以下代码:
```
import tensorflow as tf
# 创建输入层
with tf.name_scope('input'):
x = tf.placeholder(tf.float32, [None, 784], name='x-input')
y = tf.placeholder(tf.float32, [None, 10], name='y-input')
# 创建隐藏层1
with tf.name_scope('layer1'):
with tf.name_scope('weights'):
W1 = tf.Variable(tf.truncated_normal([784, 256], stddev=0.1), name='W1')
with tf.name_scope('biases'):
b1 = tf.Variable(tf.zeros([256]) + 0.1, name='b1')
with tf.name_scope('Wx_plus_b'):
L1 = tf.nn.relu(tf.matmul(x, W1) + b1)
# 创建隐藏层2
with tf.name_scope('layer2'):
with tf.name_scope('weights'):
W2 = tf.Variable(tf.truncated_normal([256, 128], stddev=0.1), name='W2')
with tf.name_scope('biases'):
b2 = tf.Variable(tf.zeros([128]) + 0.1, name='b2')
with tf.name_scope('Wx_plus_b'):
L2 = tf.nn.relu(tf.matmul(L1, W2) + b2)
# 创建输出层
with tf.name_scope('output'):
with tf.name_scope('weights'):
W3 = tf.Variable(tf.truncated_normal([128, 10], stddev=0.1), name='W3')
with tf.name_scope('biases'):
b3 = tf.Variable(tf.zeros([10]) + 0.1, name='b3')
with tf.name_scope('Wx_plus_b'):
prediction = tf.nn.softmax(tf.matmul(L2, W3) + b3)
# 定义损失函数和优化器
with tf.name_scope('loss'):
loss = tf.reduce_mean(tf.nn.softmax_cross_entropy_with_logits(labels=y, logits=prediction))
tf.summary.scalar('loss', loss)
with tf.name_scope('train'):
train_step = tf.train.AdamOptimizer(0.001).minimize(loss)
# 加载数据
from tensorflow.examples.tutorials.mnist import input_data
mnist = input_data.read_data_sets('MNIST_data', one_hot=True)
# 训练模型
with tf.Session() as sess:
merged = tf.summary.merge_all()
writer = tf.summary.FileWriter('logs/', sess.graph)
sess.run(tf.global_variables_initializer())
for epoch in range(51):
for batch_xs, batch_ys in mnist.train.next_batch(100):
sess.run(train_step, feed_dict={x: batch_xs, y: batch_ys})
# 每迭代10次记录一次日志
if epoch % 10 == 0:
result = sess.run(merged, feed_dict={x: mnist.test.images, y: mnist.test.labels})
writer.add_summary(result, epoch)
```
这段代码创建了一个包含两个隐藏层的神经网络,并使用了TensorBoard进行可视化。首先,我们使用`tf.name_scope()`给每个操作取名字,这样在TensorBoard中可以更清晰地看到图的结构。在代码中,我们使用了`tf.summary.scalar()`记录了损失函数的值。然后在训练过程中,我们使用`tf.summary.merge_all()`将所有的日志文件合并起来,使用`tf.summary.FileWriter()`将其写入到logs目录下,最后,在训练过程中每迭代10次,我们记录一次日志,以便在TensorBoard中观察训练的情况。
阅读全文
相关推荐
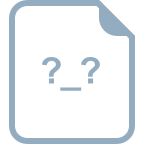
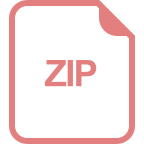
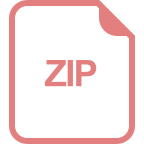












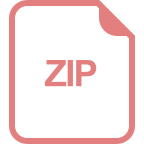
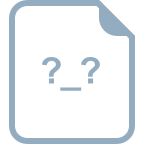
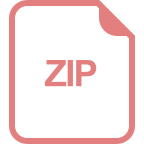
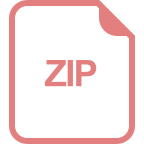