新建content.txt文本文件,使用文件字节输出流写入“English:”,然后使用文件字节输入输出流将“English.txt”中的内容读取出来追加到content.txt中,然后添加换行符,再使用文件字符输出流写入“中文:”,然后文件字符输入输出流将“Chinese.txt”中的内容读取出来追加到content.txt中。
时间: 2023-06-26 10:09:07 浏览: 146
以下是Java代码实现:
```java
import java.io.*;
public class FileIOExample {
public static void main(String[] args) {
// 写入英文
try (FileOutputStream fos = new FileOutputStream("content.txt")) {
String content = "English:";
byte[] bytes = content.getBytes();
fos.write(bytes);
} catch (IOException e) {
e.printStackTrace();
}
// 读取并追加英文
try (FileInputStream fis = new FileInputStream("English.txt");
FileOutputStream fos = new FileOutputStream("content.txt", true)) {
byte[] bytes = new byte[1024];
int len;
while ((len = fis.read(bytes)) > 0) {
fos.write(bytes, 0, len);
}
fos.write('\n'); // 换行符
} catch (IOException e) {
e.printStackTrace();
}
// 写入中文
try (FileWriter fw = new FileWriter("content.txt", true)) {
String content = "中文:";
fw.write(content);
} catch (IOException e) {
e.printStackTrace();
}
// 读取并追加中文
try (BufferedReader br = new BufferedReader(new InputStreamReader(new FileInputStream("Chinese.txt"), "UTF-8"));
BufferedWriter bw = new BufferedWriter(new OutputStreamWriter(new FileOutputStream("content.txt", true), "UTF-8"))) {
String line;
while ((line = br.readLine()) != null) {
bw.write(line);
}
} catch (IOException e) {
e.printStackTrace();
}
}
}
```
上述代码实现了以下步骤:
1. 创建一个新的文本文件"content.txt"
2. 使用文件字节输出流将"English:"写入"content.txt"
3. 使用文件字节输入输出流将"English.txt"中的内容读取出来追加到"content.txt"中
4. 在"content.txt"中添加换行符
5. 使用文件字符输出流将"中文:"写入"content.txt"
6. 使用文件字符输入输出流将"Chinese.txt"中的内容读取出来追加到"content.txt"中
注意,在第2步中,我们使用了文件字节输出流的构造函数中的第2个参数"true",表示以追加模式打开文件,以便在文件末尾追加内容。在第5步和第6步中,我们也使用了文件字符输出流的构造函数中的第2个参数"true",意味着以追加模式打开文件,以便在文件末尾追加内容。
阅读全文
相关推荐
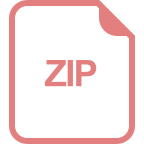
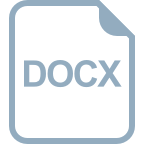
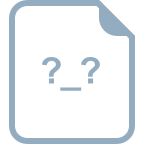
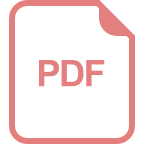
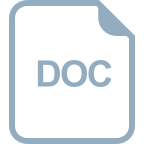
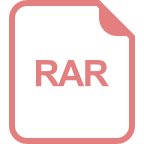
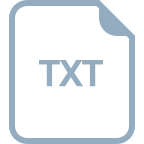
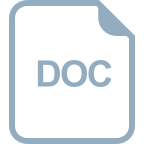
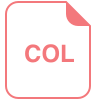
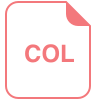
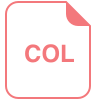
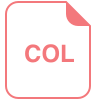
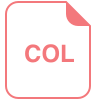
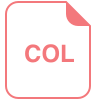


