springboot+mybatis一对多实现代码
时间: 2023-12-11 11:03:27 浏览: 67
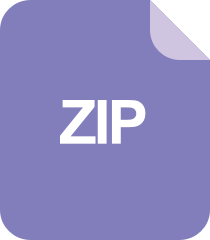
springboot+mybatis示例代码
假设有两张表,一张是学生表(student),一张是课程表(course),一个学生可以选多门课程,一门课程也可以被多个学生选中。这种情况下,我们可以使用一对多的关系来实现。下面是一个简单的实现代码:
1. 定义学生表(student)和课程表(course)的实体类
```java
public class Student {
private Long id;
private String name;
private List<Course> courses;
//省略getter和setter方法
}
public class Course {
private Long id;
private String name;
//省略getter和setter方法
}
```
2. 定义学生表(student)和课程表(course)的mapper接口
```java
public interface StudentMapper {
Student selectStudentById(Long id);
}
public interface CourseMapper {
List<Course> selectCoursesByStudentId(Long studentId);
}
```
3. 在StudentMapper.xml中定义一对多的关系
```xml
<mapper namespace="com.example.mapper.StudentMapper">
<resultMap id="studentMap" type="Student">
<id column="id" property="id" />
<result column="name" property="name" />
<collection property="courses" ofType="Course" resultMap="courseMap" />
</resultMap>
<resultMap id="courseMap" type="Course">
<id column="id" property="id" />
<result column="name" property="name" />
</resultMap>
<select id="selectStudentById" resultMap="studentMap">
SELECT s.id, s.name, c.id AS course_id, c.name AS course_name
FROM student s
LEFT JOIN student_course sc ON s.id = sc.student_id
LEFT JOIN course c ON sc.course_id = c.id
WHERE s.id = #{id}
</select>
</mapper>
```
4. 在CourseMapper.xml中定义查询课程信息的SQL语句
```xml
<mapper namespace="com.example.mapper.CourseMapper">
<select id="selectCoursesByStudentId" resultType="Course">
SELECT c.id, c.name
FROM course c
LEFT JOIN student_course sc ON c.id = sc.course_id
WHERE sc.student_id = #{studentId}
</select>
</mapper>
```
5. 编写Controller和Service层代码
```java
@RestController
public class StudentController {
@Autowired
private StudentService studentService;
@GetMapping("/students/{id}")
public Student getStudentById(@PathVariable Long id) {
return studentService.getStudentById(id);
}
}
@Service
public class StudentServiceImpl implements StudentService {
@Autowired
private StudentMapper studentMapper;
@Autowired
private CourseMapper courseMapper;
@Override
public Student getStudentById(Long id) {
Student student = studentMapper.selectStudentById(id);
List<Course> courses = courseMapper.selectCoursesByStudentId(id);
student.setCourses(courses);
return student;
}
}
```
以上就是一对多的实现代码。
阅读全文
相关推荐
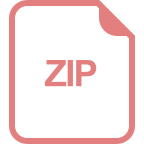
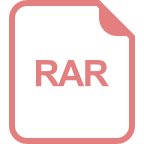
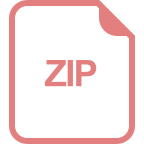
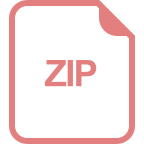
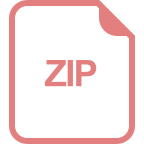
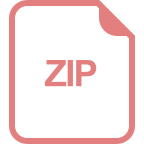
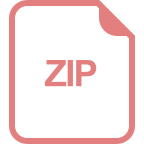
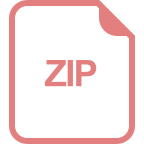
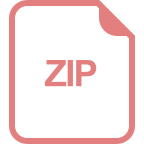
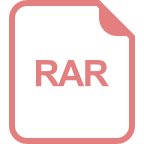
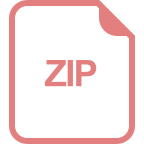
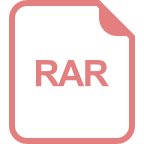
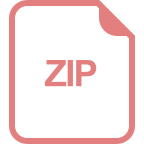
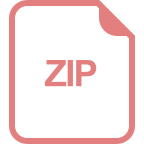
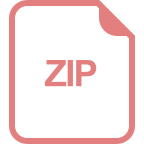
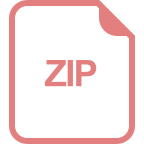
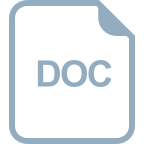