body.getBoundingClientRect()
时间: 2023-08-26 10:14:34 浏览: 70
body.getBoundingClientRect()是一个JavaScript方法,用于获取元素的大小和位置信息。它返回一个DOMRect对象,包含了元素的左上角和右下角相对于视口的位置坐标以及元素的宽度和高度。
使用该方法可以获取包围整个页面内容的body元素的大小和位置信息。例如,可以通过以下方式获取元素的宽度和高度:
```javascript
const bodyRect = document.body.getBoundingClientRect();
const width = bodyRect.width;
const height = bodyRect.height;
console.log("Width: " + width);
console.log("Height: " + height);
```
同样,可以通过以下方式获取元素相对于视口的位置坐标:
```javascript
const bodyRect = document.body.getBoundingClientRect();
const top = bodyRect.top;
const left = bodyRect.left;
console.log("Top: " + top);
console.log("Left: " + left);
```
请注意,该方法返回的坐标是相对于视口的,而不是相对于文档的。如果需要获取相对于文档的位置坐标,可以使用element.offsetTop和element.offsetLeft属性。
相关问题
element.getBoundingClientRect( )方法
`element.getBoundingClientRect()` 方法返回一个 `DOMRect` 对象,该对象提供了元素的大小及其相对于视口的位置。该方法返回的是一个矩形对象,包含了该元素的位置、大小等信息。该方法常用于获取元素的位置信息,以便进行后续的操作。
以下是一个使用 `getBoundingClientRect()` 方法获取元素位置信息的例子:
```html
<!DOCTYPE html>
<html>
<head>
<title>getBoundingClientRect() 方法示例</title>
<style type="text/css">
#box {
width: 100px;
height: 100px;
background-color: red;
position: absolute;
left: 50px;
top: 50px;
}
</style>
</head>
<body>
<div id="box"></div>
<script type="text/javascript">
var box = document.getElementById('box');
var rect = box.getBoundingClientRect();
console.log(rect); </script>
</body>
</html>
```
运行上述代码后,可以在浏览器的控制台中看到 `rect` 对象的信息,包括元素的位置、大小等信息。
<!DOCTYPE html> <html> <head> <script src="https://polyfill.io/v3/polyfill.min.js?features=es6"></script> <style> #canvas { width: 500px; height: 300px; border: 1px solid black; } </style> </head> <body> <svg id="canvas" xmlns="http://www.w3.org/2000/svg"> <text x="10" y="50" font-size="20">Drag the formula</text> <text x="10" y="70" font-size="20">Release the mouse to stop dragging</text> <foreignObject id="formula" x="100" y="100" width="200" height="100"> <!-- Insert your MathJax formula here --> <div xmlns="http://www.w3.org/1999/xhtml" style="font-size: 20px;"> \(x^2 + y^2 = r^2\) </div> </foreignObject> </svg> <script> const canvas = document.getElementById('canvas'); const formula = document.getElementById('formula'); let isDragging = false; let offsetX = 0; let offsetY = 0; function handleMouseDown(event) { isDragging = true; offsetX = event.clientX - formula.getBoundingClientRect().left; offsetY = event.clientY - formula.getBoundingClientRect().top; } function handleMouseMove(event) { if (isDragging) { formula.setAttribute('x', event.clientX - offsetX); formula.setAttribute('y', event.clientY - offsetY); } } function handleMouseUp() { isDragging = false; } canvas.addEventListener('mousedown', handleMouseDown); canvas.addEventListener('mousemove', handleMouseMove); canvas.addEventListener('mouseup', handleMouseUp); </script> </body> </html>
这段代码是一个简单的HTML页面,其目的是在一个SVG画布上展示一个文本,并允许用户通过拖动鼠标来移动这个文本。
页面中的SVG元素具有一个id为"canvas"的属性,用于绘制画布。在这个画布上,有一个文本元素和一个foreignObject元素。
文本元素用于显示一条提示信息,告诉用户可以拖动公式。foreignObject元素用于嵌入HTML内容,其中包含了一个MathJax公式。
在JavaScript部分,代码通过监听鼠标事件来实现拖拽效果。当鼠标按下时,记录下当前鼠标位置和公式的偏移量。在鼠标移动时,根据鼠标位置和偏移量来更新公式的位置。当鼠标松开时,停止拖拽操作。
此代码使用了Polyfill库来提供ES6特性的支持。
请注意,这段代码需要在支持SVG和MathJax的浏览器中运行。
阅读全文
相关推荐





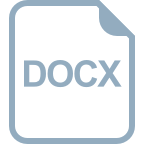
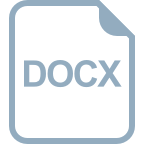
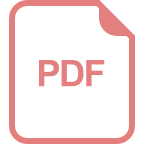
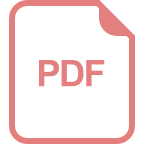
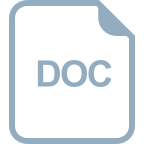
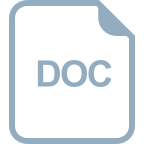



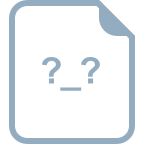