// 添加登录注册面板 JPanel loginPanel = new JPanel(); loginPanel.setBounds(200, 150, 555, 333); loginPanel.setBackground(Color.WHITE); loginPanel.setLayout(null); layeredPane.add(loginPanel, new Integer(1)); // 添加用户名和密码输入框 JLabel usernameLabel = new JLabel("用户名:"); usernameLabel.setBounds(50, 50, 80, 30); loginPanel.add(usernameLabel); JTextField usernameField = new JTextField(); usernameField.setBounds(140, 50, 200, 30); loginPanel.add(usernameField); JLabel passwordLabel = new JLabel("密码:"); passwordLabel.setBounds(50, 100, 80, 30); loginPanel.add(passwordLabel); JPasswordField passwordField = new JPasswordField(); passwordField.setBounds(140, 100, 200, 30); loginPanel.add(passwordField); 为什么面板输入框启动加载缓慢
时间: 2024-03-11 19:45:58 浏览: 114
面板和输入框加载缓慢可能和以下几个因素有关:
1. 组件数量过多:如果面板中包含大量组件,加载时间就会变长。可以考虑简化界面或者使用延迟加载等技术来提高加载速度。
2. 布局方式不合理:如果使用了复杂的布局方式,或者是使用了null布局,也可能会导致组件加载缓慢。建议使用合理的布局管理器,如GridLayout、BorderLayout、BoxLayout等。
3. 硬件性能不足:如果计算机性能不足,也会影响组件的加载速度。可以升级硬件或者优化代码来提高性能。
可以检查以上因素并根据具体情况进行优化。另外,也可以考虑使用多线程或者异步加载等技术来提高加载速度。
相关问题
package xjdz; import javax.swing.ImageIcon; import javax.swing.JFrame; import javax.swing.JLabel; public class GameWin extends JFrame{ public void launch(){ //launch启动 this.setVisible(true);//设置窗口是否可见 this.setSize(999, 666);//设置窗口大小 this.setLocationRelativeTo(null);//设置居中打开 this.setTitle("oqcw星际大战仿飞机大战小游戏");//设置窗口标题 this.setResizable(false);//设置游戏界面不可以改 this.setDefaultCloseOperation(EXIT_ON_CLOSE);//设置关闭窗口时退出程序 // this.setContentPane(new JLabel(new ImageIcon("src/bg.jpg")));//设置窗口背景 } public static void main(String[] args) { GameWin gameWin = new GameWin(); gameWin.launch(); } } 在此代码的基础上,加入一个登录注册面板
可以通过在 GameWin 类中添加一个 JPanel,来实现登录注册面板的功能。具体实现步骤如下:
1. 在 GameWin 类中添加一个 JPanel 变量,用于存放登录注册面板。
2. 在 launch 方法中,初始化登录注册面板,设置其大小和位置,并添加到窗口中。
3. 在登录注册面板中添加必要的组件,如文本框、标签和按钮等,用于实现登录和注册功能。
4. 根据需要,可以在登录注册面板中添加监听器,用于响应用户的操作。
下面是修改后的代码示例:
```java
package xjdz;
import javax.swing.ImageIcon;
import javax.swing.JButton;
import javax.swing.JFrame;
import javax.swing.JLabel;
import javax.swing.JPanel;
import javax.swing.JTextField;
public class GameWin extends JFrame{
private JPanel loginPanel; // 登录注册面板
public void launch(){
this.setVisible(true);
this.setSize(999, 666);
this.setLocationRelativeTo(null);
this.setTitle("oqcw星际大战仿飞机大战小游戏");
this.setResizable(false);
this.setDefaultCloseOperation(EXIT_ON_CLOSE);
// 初始化登录注册面板
loginPanel = new JPanel();
loginPanel.setLayout(null); // 使用绝对布局
loginPanel.setBounds(300, 200, 400, 200); // 设置大小和位置
this.getContentPane().add(loginPanel); // 添加到窗口中
// 在登录注册面板中添加组件
JLabel usernameLabel = new JLabel("用户名:");
usernameLabel.setBounds(50, 50, 60, 30);
loginPanel.add(usernameLabel);
JTextField usernameField = new JTextField();
usernameField.setBounds(120, 50, 200, 30);
loginPanel.add(usernameField);
JLabel passwordLabel = new JLabel("密码:");
passwordLabel.setBounds(50, 90, 60, 30);
loginPanel.add(passwordLabel);
JTextField passwordField = new JTextField();
passwordField.setBounds(120, 90, 200, 30);
loginPanel.add(passwordField);
JButton loginButton = new JButton("登录");
loginButton.setBounds(100, 130, 80, 30);
loginPanel.add(loginButton);
JButton registerButton = new JButton("注册");
registerButton.setBounds(220, 130, 80, 30);
loginPanel.add(registerButton);
}
public static void main(String[] args) {
GameWin gameWin = new GameWin();
gameWin.launch();
}
}
```
那在此窗口中加一个登录注册面板
好的,可以使用Java Swing来实现一个登录注册面板。以下是一个简单的示例代码:
```
import javax.swing.*;
import java.awt.*;
public class GameWin extends JFrame {
public GameWin() {
// 设置窗口属性
this.setVisible(true);
this.setSize(999, 666);
this.setLocationRelativeTo(null);
this.setTitle("oqcw星际大战仿飞机大战小游戏");
this.setResizable(false);
this.setDefaultCloseOperation(EXIT_ON_CLOSE);
// 设置背景图片
JLayeredPane layeredPane = new JLayeredPane();
setContentPane(layeredPane);
JLabel backgroundImageLabel = new JLabel(new ImageIcon("src/bg.jpg"));
backgroundImageLabel.setBounds(0, 0, getWidth(), getHeight());
layeredPane.add(backgroundImageLabel, new Integer(0));
// 添加登录注册面板
JPanel loginPanel = new JPanel();
loginPanel.setBounds(300, 200, 400, 200);
loginPanel.setBackground(Color.WHITE);
loginPanel.setLayout(null);
layeredPane.add(loginPanel, new Integer(1));
// 添加用户名和密码输入框
JLabel usernameLabel = new JLabel("用户名:");
usernameLabel.setBounds(50, 50, 80, 30);
loginPanel.add(usernameLabel);
JTextField usernameField = new JTextField();
usernameField.setBounds(140, 50, 200, 30);
loginPanel.add(usernameField);
JLabel passwordLabel = new JLabel("密码:");
passwordLabel.setBounds(50, 100, 80, 30);
loginPanel.add(passwordLabel);
JPasswordField passwordField = new JPasswordField();
passwordField.setBounds(140, 100, 200, 30);
loginPanel.add(passwordField);
// 添加登录和注册按钮
JButton loginButton = new JButton("登录");
loginButton.setBounds(100, 150, 80, 30);
loginPanel.add(loginButton);
JButton registerButton = new JButton("注册");
registerButton.setBounds(220, 150, 80, 30);
loginPanel.add(registerButton);
}
public static void main(String[] args) {
new GameWin();
}
}
```
这段代码中,我们创建了一个JPanel来表示登录注册面板,并将它添加到窗口的第一层级中(层级为1)。在这个面板中,我们添加了用户名和密码输入框,以及登录和注册按钮。可以通过设置setLayout(null)来取消默认的布局管理器,然后使用setBounds()方法来设置组件的位置和大小。这里设置了面板的背景颜色为白色,以便与窗口背景区分开来。
您可以根据需要修改这段代码,以满足您的需求。希望这能帮到您!
阅读全文
相关推荐
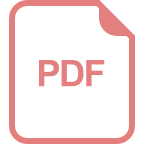
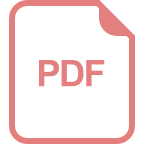
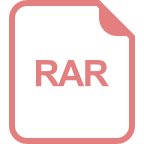











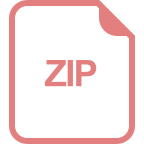
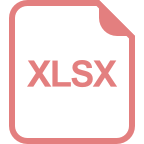