python DASH 如何开发自己的组件给个具体代码
时间: 2024-04-29 12:25:34 浏览: 12
以下是一个简单的 Python DASH 组件的代码示例:
```python
import dash
import dash_html_components as html
from dash.dependencies import Input, Output, State
import dash_core_components as dcc
# 创建自定义组件
class MyComponent(dcc.Component):
# 组件的唯一 ID
_namespace = 'mycomponent'
# 组件的默认属性
_default_props = {'label': 'Hello World!'}
# 组件的属性列表
_prop_names = ['label']
# 组件的 HTML 元素
_type = 'div'
# 组件的构造函数
def __init__(self, id, label=None, **kwargs):
super().__init__(id=id, **kwargs)
self.label = label or self._default_props['label']
# 组件的渲染方法
def render(self):
return html.Div(self.label, id=self.id)
# 创建 Dash 应用
app = dash.Dash(__name__)
# 创建自定义组件实例
my_component = MyComponent('my-component', label='My Custom Component')
# 将组件添加到应用布局中
app.layout = html.Div([
html.H1('My App'),
my_component,
html.Button('Update Component', id='update-button'),
html.Div(id='output')
])
# 定义更新组件的回调函数
@app.callback(Output('my-component', 'label'), [Input('update-button', 'n_clicks')])
def update_label(n_clicks):
if n_clicks:
return f'Updated Label ({n_clicks})'
else:
return my_component.label
if __name__ == '__main__':
app.run_server(debug=True)
```
这个例子中,我们首先定义了一个名为 `MyComponent` 的自定义组件类。这个类继承自 `dcc.Component`,并定义了组件的 ID、默认属性、属性列表和 HTML 元素类型等信息。类中还包含了构造函数和渲染方法等。
在应用布局中,我们创建了一个 `MyComponent` 的实例,并将其添加到了布局中。我们还定义了一个按钮,用于更新组件的标签。在回调函数中,我们使用 `Input` 和 `Output` 装饰器,将按钮的点击事件作为输入,将组件的标签作为输出。回调函数会根据按钮点击次数更新标签。
这个例子只是一个简单的示例,你可以根据自己的需求来定义自己的组件。
相关推荐
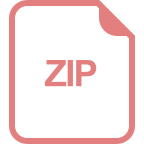
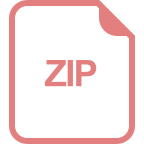
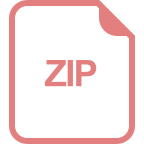














