根据下面的要求写出stm32的代码,自行设定管脚与3个 RGB LED 相连(同时也是某个定时器的复用管脚);根据 RGB 颜色表,查出红、绿、蓝、黄、紫、青、白七种颜色的颜色值。 2利用定时器结合 PWM,输出周期为 100ms的周期信号,让RGB小灯循环显示 红、绿、蓝、黄、紫、青、白 七种颜色,间隔 1S。 3实现红、绿、蓝、黄、紫、青、白七种颜色单独闪烁,间隔 1S。 @实现红绿、绿蓝、红蓝、红绿蓝间隔闪烁,间隔 1S。
时间: 2023-08-14 12:06:39 浏览: 143
以下是基于STM32 HAL库的代码实现:
```c
#include "main.h"
#include "tim.h"
#include "gpio.h"
#define RED 0
#define GREEN 1
#define BLUE 2
static uint8_t cnt = 0;
static uint8_t color = 0;
// RGB颜色值表
static uint8_t colors[7][3] = {
{255, 0, 0}, // 红
{0, 255, 0}, // 绿
{0, 0, 255}, // 蓝
{255, 255, 0}, // 黄
{255, 0, 255}, // 紫
{0, 255, 255}, // 青
{255, 255, 255} // 白
};
void TIM_PWM_SetDuty(TIM_HandleTypeDef *htim, uint32_t channel, uint16_t duty) {
uint16_t period = htim->Init.Period;
uint16_t pulse = (duty * period) / 100;
__HAL_TIM_SET_COMPARE(htim, channel, pulse);
}
void SetColor(uint8_t r, uint8_t g, uint8_t b) {
TIM_PWM_SetDuty(&htim2, TIM_CHANNEL_1, r);
TIM_PWM_SetDuty(&htim2, TIM_CHANNEL_2, g);
TIM_PWM_SetDuty(&htim2, TIM_CHANNEL_3, b);
}
void HAL_TIM_PeriodElapsedCallback(TIM_HandleTypeDef *htim) {
if (htim->Instance == htim3.Instance) {
cnt++;
if (cnt >= 10) {
cnt = 0;
color++;
if (color >= 7) {
color = 0;
}
}
if (cnt == 1) {
SetColor(colors[color][RED], colors[color][GREEN], colors[color][BLUE]);
}
}
}
void RGB_Loop() {
HAL_TIM_Base_Start_IT(&htim3);
}
void RGB_Blink() {
for (uint8_t i = 0; i < 7; i++) {
SetColor(colors[i][RED], colors[i][GREEN], colors[i][BLUE]);
HAL_Delay(1000);
SetColor(0, 0, 0);
HAL_Delay(1000);
}
}
void RGB_AlternateBlink() {
SetColor(colors[RED][RED], colors[RED][GREEN], 0);
HAL_Delay(1000);
SetColor(0, colors[GREEN][GREEN], colors[GREEN][BLUE]);
HAL_Delay(1000);
SetColor(colors[RED][RED], 0, colors[BLUE][BLUE]);
HAL_Delay(1000);
SetColor(colors[RED][RED], colors[GREEN][GREEN], colors[BLUE][BLUE]);
HAL_Delay(1000);
SetColor(0, 0, 0);
}
int main(void) {
HAL_Init();
MX_GPIO_Init();
MX_TIM2_Init();
MX_TIM3_Init();
RGB_Loop();
while (1) {
RGB_Blink();
RGB_AlternateBlink();
}
}
```
在代码中,我们首先定义了三个宏分别表示 RGB 的三个颜色通道,以及颜色值表 `colors`。然后定义了 `TIM_PWM_SetDuty` 函数,它可以根据占空比设置 PWM 波形的输出电平。接下来是 `SetColor` 函数,它可以根据传入的 RGB 值设置 LED 的亮度。
在 `HAL_TIM_PeriodElapsedCallback` 回调函数中,我们对计数器 `cnt` 进行更新和判断,如果计数器 `cnt` 的值达到 10,即 100ms 的周期,就更新颜色 `color` 并设置 LED 的亮度。
在 `RGB_Loop` 函数中,我们启动了定时器 TIM3 并开启了定时器中断。在 `RGB_Blink` 函数中,我们对颜色值表 `colors` 进行循环遍历,并分别在每个颜色上亮灯 1 秒,熄灭 1 秒。在 `RGB_AlternateBlink` 函数中,我们按照题目要求对红绿、绿蓝、红蓝、红绿蓝进行间隔闪烁。
最后在 `main` 函数中,我们初始化 GPIO 和定时器,并循环调用 `RGB_Blink` 和 `RGB_AlternateBlink` 函数。
阅读全文
相关推荐
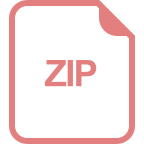
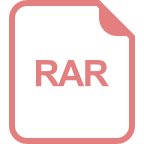
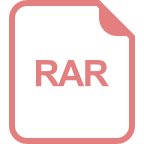

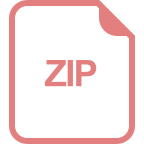
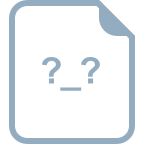
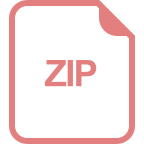
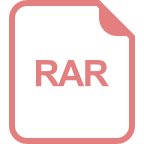
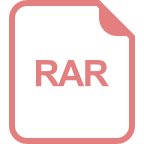
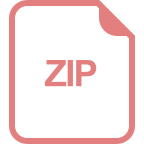
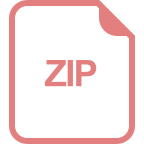
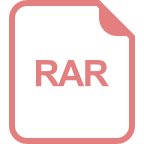
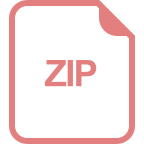
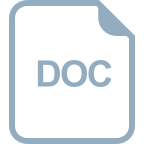
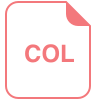



