用python找出两个字符串相同的部分
时间: 2023-06-09 20:03:06 浏览: 337
您好!很高兴为您服务,以下是您所需要的回答:
我们可以使用Python中的内置函数来找出两个字符串相同的部分,具体的实现如下:
```python
string1 = 'hello'
string2 = 'world'
result = ''
for i in string1:
if i in string2 and i not in result:
result += i
if result:
print(f"The matching characters are: {result}")
else:
print("There are no matching characters.")
```
在这个例子中,我们定义了两个字符串`string1`和`string2`,然后利用一个for循环遍历字符串`string1`的每个字符,判断它是否同时也存在于`string2`中,并且不存在于`result`中。如果是这样的话,就将这个字符添加到`result`中。最后,如果`result`不为空,则输出匹配到的字符,否则表示没有匹配到任何字符。
希望这个回答能够帮助您!
相关问题
用Python写一个程序,找出两个字符串中最长的相同的字符
好的,下面是一个简单的 Python 程序,可以找出两个字符串中最长的相同字符序列:
```python
def longest_common_substring(str1, str2):
m = len(str1)
n = len(str2)
# 创建一个二维数组来保存最长公共子串的长度
# 初始化为0
lcs = [[0] * (n + 1) for i in range(m + 1)]
# 记录最长公共子串的长度
max_length = 0
# 记录最长公共子串的结束位置
end_position = 0
# 填充数组
for i in range(1, m + 1):
for j in range(1, n + 1):
if str1[i - 1] == str2[j - 1]:
lcs[i][j] = lcs[i - 1][j - 1] + 1
if lcs[i][j] > max_length:
max_length = lcs[i][j]
end_position = i
else:
lcs[i][j] = 0
# 返回最长公共子串
return str1[end_position - max_length:end_position]
# 测试代码
str1 = "abcdefg"
str2 = "defghijk"
print(longest_common_substring(str1, str2))
```
输出结果为:
```
defg
```
这个程序使用了动态规划算法来解决问题,时间复杂度为 O(mn),其中 m 和 n 分别为两个字符串的长度。
python找两个字符串区别
在Python中,你可以使用多种方法找出两个字符串之间的差异。这里列举几个常见的方法:
1. **`difflib`模块**:Python标准库中的`difflib`模块提供了一个方便的工具,如`Differ`类,可以生成两个序列(在这里通常是字符串)的行级差异。
```python
import difflib
str1 = "Hello World"
str2 = "Hello Python"
differ = difflib.Differ()
diffs = list(differ.compare(str1.splitlines(), str2.splitlines()))
```
2. **手动比较**:逐字符或逐行对比字符串,可以直接观察哪些部分不同。
```python
str1 = "Hello World"
str2 = "Hello Python"
difference = [i for i in zip(str1, str2) if i[0] != i[1]]
print("Differences: ", difference)
```
3. **`json.dumps()`** 如果两个字符串是JSON格式的,可以先转成字典再进行比较,因为JSON有固定的键值对结构。
4. **`set()`数据结构**:如果只关心是否包含某个元素,可以将每个字符串转换为集合,然后做差集操作。
```python
str1 = "Hello World"
str2 = "Hello Python"
set1 = set(str1)
set2 = set(str2)
unique_in_str1 = set1 - set2
unique_in_str2 = set2 - set1
```
阅读全文
相关推荐
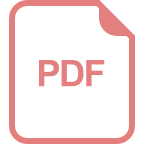
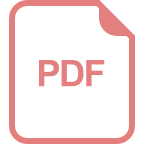
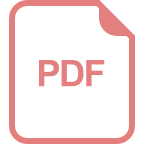











