java 如何把多个视频文件以流的形式传给前端
时间: 2024-03-02 19:52:44 浏览: 57
在 Java 中,可以使用 Spring MVC 框架的 `StreamingResponseBody` 接口将多个视频文件以流的形式传给前端。以下是一个示例代码:
```java
import org.springframework.core.io.InputStreamResource;
import org.springframework.http.HttpHeaders;
import org.springframework.http.HttpStatus;
import org.springframework.http.MediaType;
import org.springframework.http.ResponseEntity;
import org.springframework.stereotype.Controller;
import org.springframework.web.bind.annotation.GetMapping;
import org.springframework.web.bind.annotation.ResponseBody;
import org.springframework.web.bind.annotation.ResponseStatus;
import org.springframework.web.servlet.mvc.method.annotation.StreamingResponseBody;
import java.io.*;
import java.util.ArrayList;
import java.util.List;
import java.util.zip.ZipEntry;
import java.util.zip.ZipOutputStream;
@Controller
public class VideoController {
@GetMapping("/videos")
@ResponseBody
public ResponseEntity<StreamingResponseBody> getVideos() throws IOException {
List<File> videoFiles = new ArrayList<>();
videoFiles.add(new File("video1.mp4"));
videoFiles.add(new File("video2.mp4"));
StreamingResponseBody responseBody = outputStream -> {
try (ZipOutputStream zipOutputStream = new ZipOutputStream(outputStream)) {
for (File videoFile : videoFiles) {
try (InputStream inputStream = new FileInputStream(videoFile)) {
ZipEntry zipEntry = new ZipEntry(videoFile.getName());
zipOutputStream.putNextEntry(zipEntry);
byte[] buffer = new byte[1024];
int length;
while ((length = inputStream.read(buffer)) > 0) {
zipOutputStream.write(buffer, 0, length);
}
zipOutputStream.closeEntry();
}
}
zipOutputStream.finish();
}
};
HttpHeaders headers = new HttpHeaders();
headers.setContentType(MediaType.APPLICATION_OCTET_STREAM);
headers.setContentDispositionFormData("attachment", "videos.zip");
return new ResponseEntity<>(responseBody, headers, HttpStatus.OK);
}
}
```
在这个示例中,我们定义了一个 `VideoController` 类,并在其中实现了一个 `/videos` 接口。在这个接口中,我们定义了一个 `StreamingResponseBody` 对象,并在其中将多个视频文件压缩成一个 zip 文件,然后以流的形式传回给前端。我们还定义了一个 `HttpHeaders` 对象,用于设置返回的内容类型和文件名。
在前端中,我们可以通过 `XMLHttpRequest` 对象来调用这个接口,然后在回调函数中处理返回的 zip 文件。具体的代码可以参考以下示例:
```javascript
function downloadVideos() {
const xhr = new XMLHttpRequest();
xhr.open('GET', '/videos', true);
xhr.responseType = 'blob';
xhr.onreadystatechange = function () {
if (xhr.readyState === 4 && xhr.status === 200) {
const blob = xhr.response;
const link = document.createElement('a');
link.href = window.URL.createObjectURL(blob);
link.download = 'videos.zip';
link.click();
}
};
xhr.send();
}
```
在这个示例中,我们使用 `XMLHttpRequest` 对象来调用 `/videos` 接口,并将响应类型设置为 blob。在回调函数中,我们将响应内容转换为一个 blob 对象,并创建一个下载链接,用于下载返回的 zip 文件。
阅读全文
相关推荐
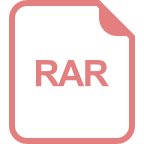
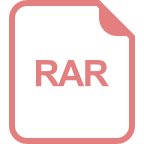
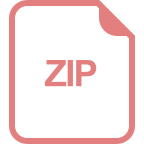
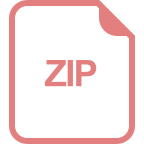
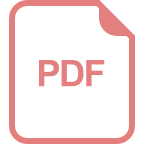
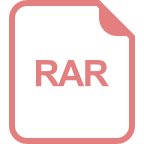
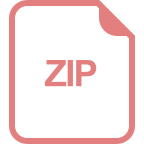
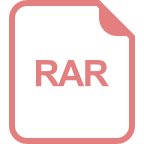
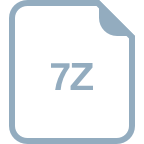
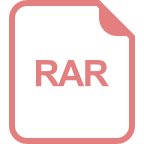
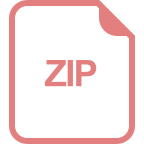
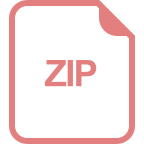
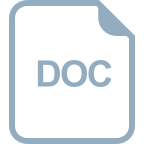
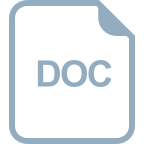
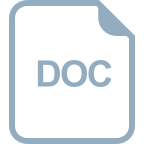
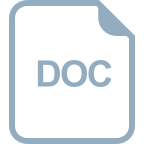