python 写一个端口转发从而实现局域网内没有外网的电脑可以远程连接代码 请写出实现的每一步的步骤
时间: 2024-02-17 21:00:51 浏览: 64
好的,以下是实现端口转发的每一步骤:
1. 导入 `socket` 模块,使用其中的函数实现网络通信和套接字操作。
```python
import socket
```
2. 定义一个函数 `port_forward`,该函数接受三个参数:本地端口、远程主机和远程端口。在该函数中,我们首先创建一个本地套接字,绑定到本地端口,并开始监听连接请求。
```python
def port_forward(local_port, remote_host, remote_port):
with socket.socket(socket.AF_INET, socket.SOCK_STREAM) as local_sock:
local_sock.bind(('0.0.0.0', local_port))
local_sock.listen(1)
print(f'Listening on port {local_port}...')
```
3. 接着,我们使用 `accept()` 方法等待客户端连接。当有连接请求时,我们创建一个远程套接字,连接到远程主机和端口,并打印连接信息。
```python
while True:
conn, addr = local_sock.accept()
print(f'Accepted connection from {addr[0]}:{addr[1]}')
with socket.socket(socket.AF_INET, socket.SOCK_STREAM) as remote_sock:
remote_sock.connect((remote_host, remote_port))
print(f'Connected to {remote_host}:{remote_port}')
```
4. 后面我们需要将从本地套接字接收到的数据发送到远程套接字,并将从远程套接字接收到的响应发送回本地套接字。为此,我们使用 `recv()` 方法从本地套接字接收数据,然后使用 `sendall()` 方法将数据发送到远程套接字。我们还打印每个数据包的大小。
```python
while True:
data = conn.recv(1024)
if not data:
break
remote_sock.sendall(data)
print(f'Sent {len(data)} bytes to {remote_host}:{remote_port}')
```
5. 最后,我们关闭本地和远程套接字,并打印连接关闭的信息。
```python
conn.close()
print(f'Connection closed by {addr[0]}:{addr[1]}')
```
6. 在 `main` 函数中,我们调用 `port_forward` 函数,设置本地端口为 `8080`,远程主机为 `example.com`,远程端口为 `80`。
```python
if __name__ == '__main__':
port_forward(8080, 'example.com', 80)
```
到这里,我们就实现了从本地端口转发到远程主机和端口的端口转发程序。
阅读全文
相关推荐
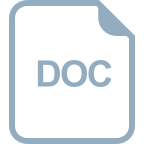
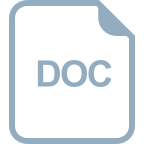
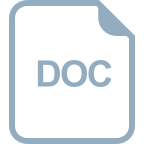
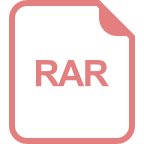
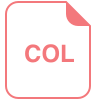
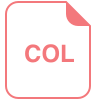
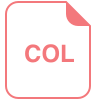
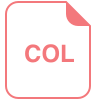
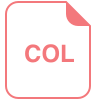
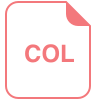
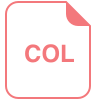
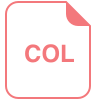
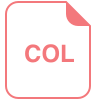
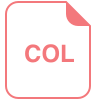
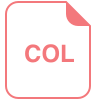
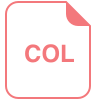
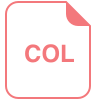
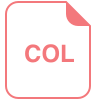
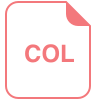