c++计算器功能模拟程序设计 实现一个具有一定功能的计算器,能够进行整数、实数、复数的加、减、乘、除、乘方和求对数等运算。使用时算式采用后缀输入法,每个操作数、操作符之间都以空白符分隔。例如,若要计算"3+5"则输入"3 5 +"。乘方运算符用"^"表示。要求: (1)每次运算在前次结果基础上进行,若要将前次运算结果清除,可键入"c"。当键入"q"时程序结束; (2)界面交互性好。
时间: 2024-02-25 14:59:30 浏览: 27
以下是一个基本的 C++ 计算器程序,它支持整数、实数和复数的加、减、乘、除、乘方和求对数等运算,并使用后缀输入法。
```c++
#include <iostream>
#include <stack>
#include <cmath>
#include <complex>
#include <cstring>
using namespace std;
// 判断是否为数字
bool is_number(char c) {
return (c >= '0' && c <= '9') || c == '.' || c == 'i' || c == 'j';
}
// 判断是否为操作符
bool is_operator(char c) {
return c == '+' || c == '-' || c == '*' || c == '/' || c == '^' || c == 'L';
}
// 判断操作符优先级
int operator_priority(char c) {
if (c == '+' || c == '-') {
return 1;
} else if (c == '*' || c == '/') {
return 2;
} else if (c == '^') {
return 3;
} else if (c == 'L') {
return 4;
}
return 0;
}
// 将字符串转换为数字
template <typename T>
void str_to_num(const char* str, T& num) {
stringstream ss(str);
ss >> num;
}
// 处理操作符
void handle_operator(char op, stack<complex<double>>& nums) {
complex<double> num2 = nums.top();
nums.pop();
complex<double> num1 = nums.top();
nums.pop();
switch (op) {
case '+':
nums.push(num1 + num2);
break;
case '-':
nums.push(num1 - num2);
break;
case '*':
nums.push(num1 * num2);
break;
case '/':
nums.push(num1 / num2);
break;
case '^':
nums.push(pow(num1, num2));
break;
case 'L':
nums.push(log(num1) / log(num2));
break;
}
}
int main() {
stack<complex<double>> nums; // 存储数字的栈
string input; // 存储用户输入的字符串
while (true) {
cout << ">>> ";
getline(cin, input);
if (input == "q") { // 退出程序
break;
} else if (input == "c") { // 清空栈
while (!nums.empty()) {
nums.pop();
}
cout << "Stack cleared." << endl;
continue;
}
// 将输入字符串按空格分割为多个子字符串
vector<string> tokens;
char* token = strtok((char*)input.c_str(), " ");
while (token) {
tokens.push_back(string(token));
token = strtok(NULL, " ");
}
// 处理每个子字符串
for (auto& token : tokens) {
if (is_number(token[0])) { // 处理数字
if (token.back() == 'i' || token.back() == 'j') { // 处理复数
complex<double> num;
str_to_num(token.substr(0, token.length() - 1).c_str(), num.real());
num.imag(1);
nums.push(num);
} else { // 处理实数
double num;
str_to_num(token.c_str(), num);
nums.push(num);
}
} else if (is_operator(token[0])) { // 处理操作符
while (!nums.empty() && is_operator(token[0]) && operator_priority(token[0]) <= operator_priority(to_string(nums.top())[0])) {
handle_operator(token[0], nums);
}
}
}
// 输出结果
if (!nums.empty()) {
cout << nums.top() << endl;
}
}
return 0;
}
```
使用后缀输入法的计算器程序,可以避免使用括号来表示运算优先级。例如,要计算 "(3 + 5) * 2",使用后缀输入法可以写成 "3 5 + 2 *",程序会按照正确的优先级进行计算。
相关推荐
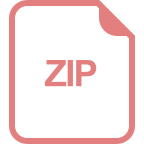
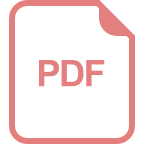
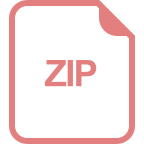







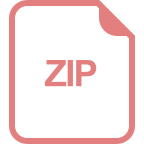
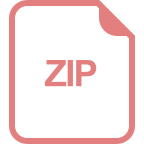
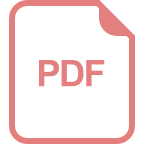