用Python代码实现:以15数码问题为例实现A*算法的求解程序
时间: 2024-11-25 13:14:53 浏览: 31
在Python中实现A*搜索算法解决15数码问题,首先你需要定义状态空间、代价函数和启发式函数。以下是一个简单的例子,假设我们有一个二维网格世界,目标是找到从起点到终点的最短路径:
```python
import heapq
# 定义节点类
class Node:
def __init__(self, state, parent=None, cost=0, heuristic=0):
self.state = state
self.parent = parent
self.cost = cost
self.heuristic = heuristic
def __lt__(self, other):
return self.total_cost() < other.total_cost()
def total_cost(self):
return self.cost + self.heuristic
# 假设grid是一个表示地图状态的二维数组,start和end分别是起始点和终点坐标
def a_star_search(grid, start, end):
open_list = [Node(start)]
closed_list = []
while open_list:
current = heapq.heappop(open_list)
# 如果当前节点是终点
if current.state == end:
path = []
while current is not None:
path.append(current.state)
current = current.parent
return path[::-1] # 返回路径
closed_list.append(current)
# 邻接节点探索
for neighbor in get_neighbors(grid, current.state):
tentative_g_score = current.cost + 1 # 这里用1作为代价,实际情况下根据环境可能不同
if neighbor not in closed_list or tentative_g_score < neighbor.cost:
neighbor.parent = current
neighbor.cost = tentative_g_score
neighbor.heuristic = heuristic_function(neighbor.state, end) # 填充启发式函数计算值
heapq.heappush(open_list, neighbor) # 推入优先队列
# 获取邻居函数,根据实际情况修改,这里假设网格相邻单元格是可达的
def get_neighbors(grid, pos):
neighbors = [(pos+dx, pos+dy) for dx, dy in [(0, -1), (0, 1), (-1, 0), (1, 0)]]
valid_neighbors = [(x, y) for x, y in neighbors if 0 <= x < len(grid) and 0 <= y < len(grid) and grid[x][y] != 'blocked']
return valid_neighbors
# 假设heuristic_function是估计从当前位置到终点的曼哈顿距离或其他合适的启发式方法
heuristic_function = lambda x, y: abs(x-y) + abs(x-y)
# 初始化并调用A*搜索
solution = a_star_search(grid, (0, 0), (len(grid)-1, len(grid)-1))
```
请注意这只是一个基础框架,实际应用中可能需要对启发式函数、成本评估和边界处理等部分进行优化。如果你提供特定的网格结构或启发式函数需求,我可以给出更详细的代码实现。
阅读全文
相关推荐
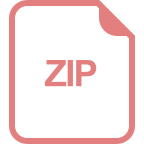
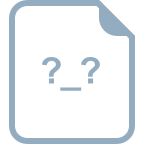
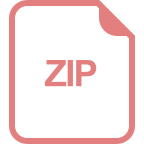
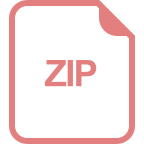
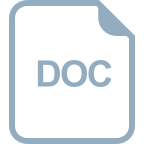

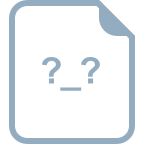
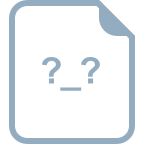
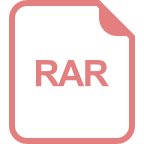
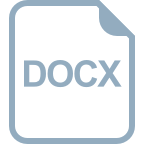
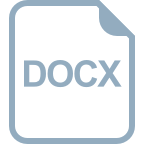
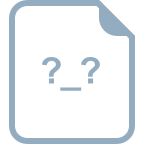
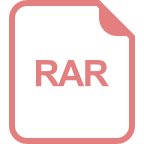
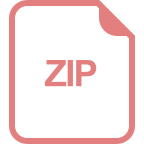
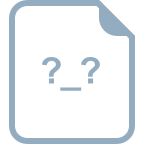
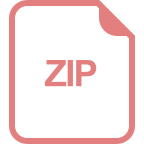
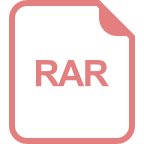