编写c语言算法:输入数个字符利用尾插法建立字符单链表。在单链表上练习①打印输出该单链表;②输出单链表长度;③按值查找;④插入元素。
时间: 2024-09-21 10:06:21 浏览: 45
在C语言中,我们可以使用结构体表示链表节点,并通过指针操作来实现链表。以下是创建、插入、打印和查找的基本步骤:
1. **创建链表头结点:**
```c
typedef struct Node {
char data;
struct Node* next;
} Node;
```
2. **输入字符并构建链表:**(尾插法)
```c
Node* insert(char c) {
Node* newNode = (Node*)malloc(sizeof(Node));
if (newNode == NULL) {
printf("Memory allocation failed.\n");
return NULL;
}
newNode->data = c;
newNode->next = NULL;
if (head == NULL) { // 链表为空
head = newNode;
} else {
Node* temp = head;
while (temp->next != NULL) {
temp = temp->next;
}
temp->next = newNode; // 尾部插入
}
return head;
}
```
3. **打印链表:**(遍历)
```c
void printList(Node* node) {
while (node != NULL) {
printf("%c ", node->data);
node = node->next;
}
printf("\n");
}
```
4. **计算链表长度:**
```c
int listLength(Node* node) {
int count = 0;
while (node != NULL) {
count++;
node = node->next;
}
return count;
}
```
5. **按值查找:**
```c
Node* search(char target, Node* node) {
while (node != NULL) {
if (node->data == target) {
return node;
}
node = node->next;
}
return NULL; // 如果未找到目标,返回NULL
}
```
6. **插入元素:**在指定位置插入
```c
void insertAtPosition(int position, char value, Node** headRef) {
if (position <= 0 || *headRef == NULL) {
return;
}
Node* newNode = (Node*)malloc(sizeof(Node));
if (newNode == NULL) {
printf("Memory allocation failed.\n");
return;
}
newNode->data = value;
newNode->next = NULL;
if (position == 1) { // 插入在第一个位置
newNode->next = *headRef;
*headRef = newNode;
} else {
Node* prevNode = *headRef;
int i;
for (i = 1; i < position - 1 && prevNode != NULL; i++) {
prevNode = prevNode->next;
}
if (prevNode == NULL) {
printf("Invalid position.\n");
return;
}
newNode->next = prevNode->next;
prevNode->next = newNode;
}
}
```
阅读全文
相关推荐
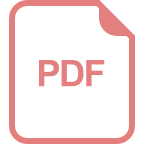
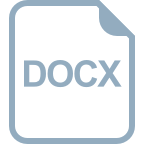
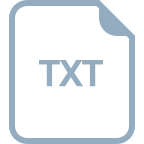
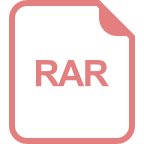
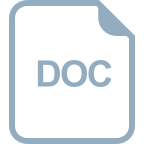
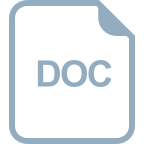
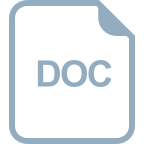
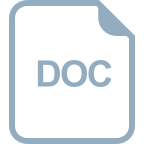
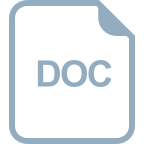
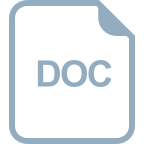
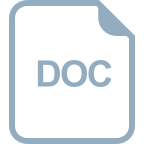
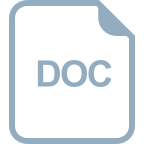
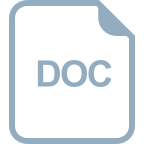
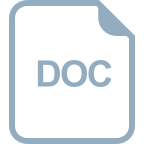
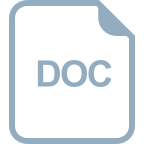
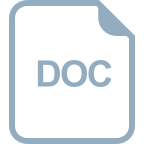