图书管理系统数组程序代码
时间: 2024-08-12 11:00:36 浏览: 45
图书管理系统通常会使用数组来存储书籍的信息,如书名、作者、ISBN号等。这里是一个简单的图书管理系统的数组代码示例,使用C++语言:
```cpp
#include <iostream>
#include <string>
class Book {
public:
std::string title;
std::string author;
std::string isbn;
Book(std::string t, std::string a, std::string i) : title(t), author(a), isbn(i) {}
};
class Library {
private:
Book books[100]; // 假设最多有100本书
int bookCount = 0;
public:
void addBook(const std::string& title, const std::string& author, const std::string& isbn) {
if (bookCount < 100) {
books[bookCount++] = Book(title, author, isbn);
std::cout << "Book added successfully: " << title << " by " << author << ", ISBN: " << isbn << std::endl;
} else {
std::cout << "No more space in the library." << std::endl;
}
}
void displayBooks() {
for (int i = 0; i < bookCount; i++) {
std::cout << "Title: " << books[i].title << ", Author: " << books[i].author << ", ISBN: " << books[i].isbn << std::endl;
}
}
};
int main() {
Library library;
library.addBook("The Great Gatsby", "F. Scott Fitzgerald", "978-0743273565");
library.addBook("To Kill a Mockingbird", "Harper Lee", "978-0446310789");
library.displayBooks();
return 0;
}
```
在这个例子中,`Book` 类定义了书籍的基本属性,`Library` 类有一个数组 `books` 存储书籍,并提供了添加书籍和显示所有书籍的方法。`main` 函数展示了如何创建和操作图书馆。
阅读全文
相关推荐
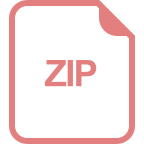
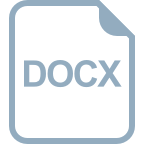
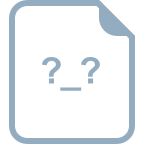
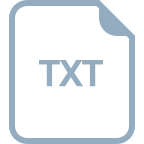
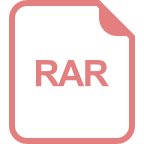
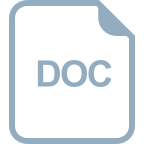

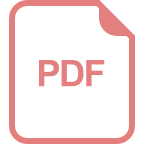
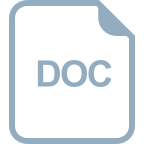
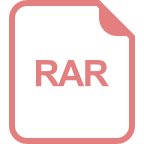
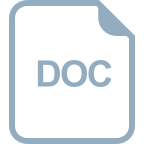
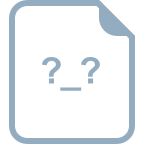
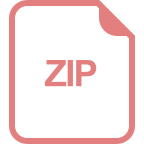
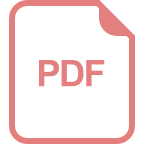
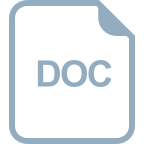
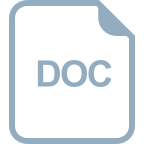
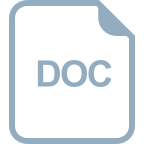