c++条件语句大小写字母转换
时间: 2024-06-26 20:01:40 浏览: 149
在C++中,你可以使用条件语句(如if-else、switch等)结合字符处理函数来实现大小写字母的转换。以下是一些基本的方法:
1. 使用标准库函数`islower()`和`isupper()`检查字符是否为小写或大写,然后用`toupper()`或`tolower()`进行转换:
```cpp
char ch;
if (islower(ch)) {
ch = toupper(ch);
} else if (isupper(ch)) {
ch = tolower(ch);
}
```
2. 对于`std::string`类型的字符串,可以遍历每个字符并应用转换:
```cpp
std::string str;
for (char &c : str) {
c = islower(c) ? toupper(c) : tolower(c);
}
```
3. 使用`std::transform()`和`std::tolower()`或`toupper()`函数批量转换字符串:
```cpp
std::transform(str.begin(), str.end(), str.begin(), ::tolower); // 转换为小写
// 或者
std::transform(str.begin(), str.end(), str.begin(), ::toupper); // 转换为大写
```
相关问题
c++条件语句大小写字母转换代码
在C++中,你可以使用条件语句(如if-else、switch等)配合字符类型的操作来实现大小写字母的转换。这里是一个简单的例子,展示了如何将小写字母转换为大写和大写字母转换为小写:
```cpp
#include <iostream>
#include <cctype> // 引入cctype库,包含对字符大小写的处理函数
// 将小写字母转为大写
char toUpper(char c) {
return islower(c) ? toupper(c) : c; // 使用islower判断是否为小写,然后用toupper转换
}
// 将大写字母转为小写
char toLower(char c) {
return isupper(c) ? tolower(c) : c; // 使用isupper判断是否为大写,然后用tolower转换
}
int main() {
std::string input;
std::cout << "Enter a string: ";
std::getline(std::cin, input);
for (char& ch : input) { // 遍历字符串中的每个字符
if (islower(ch)) { // 如果是小写字母
ch = toUpper(ch); // 转换为大写
} else if (isupper(ch)) { // 如果是大写字母
ch = toLower(ch); // 转换为小写
}
}
std::cout << "Converted string: " << input << std::endl;
return 0;
}
```
c语言大小写字母转换
C语言中大小写字母转换可以使用不同的方法来实现。下面我将介绍三种常见的方法:
方法(1):使用条件判断语句
```c
#include <stdio.h>
int main() {
char ch;
printf("请输入一个字符:\n");
scanf("%c", &ch);
if (ch >= 'a' && ch <= 'z') {
ch -= 32;
printf("%c\n", ch);
} else if (ch >= 'A' && ch <= 'Z') {
ch += 32;
printf("%c\n", ch);
} else {
printf("输入的不是大写或者小写字母\n");
}
return 0;
}
```
方法(2):使用ASCII码进行转换
```c
#include <stdio.h>
void main() {
char a;
printf("请输入一个字母:");
scanf("%c", &a);
if (a >= 'A' && a <= 'Z') {
a += 32;
printf("该字母的小写形式为:%c\n", a);
} else if (a >= 'a' && a <= 'z') {
a -= 32;
printf("该字母的大写形式为:%c\n", a);
} else {
printf("输入的不是字母\n");
}
}
```
方法(3):使用C++中的ctype库函数
```c++
#include <iostream>
#include <ctype.h>
#include <cstring>
using namespace std;
int main() {
char a[100];
int n, i;
cin >> a;
n = strlen(a);
for (i = 0; i < n; i++) {
a[i] = toupper(a[i]); //小写转大写
}
cout << a << endl;
for (i = 0; i < n; i++) {
a[i] = tolower(a[i]); //大写转小写字母(只能是对字母有效)
}
cout << a << endl;
return 0;
}
```
阅读全文
相关推荐
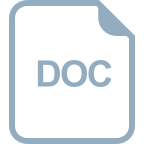
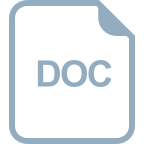
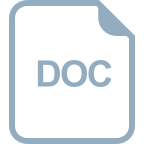

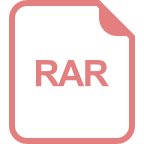
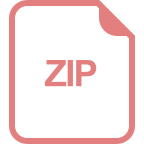
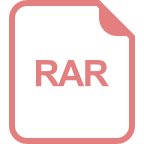
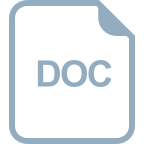
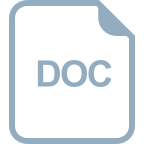
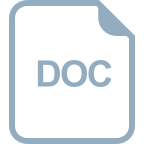
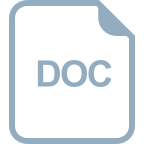





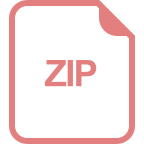
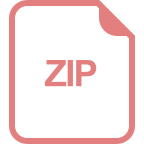