在C#编程中,如何处理byte数组,以便仅保留其中间连续的零('00'),同时删除末尾多余的零?
时间: 2024-11-15 18:27:29 浏览: 14
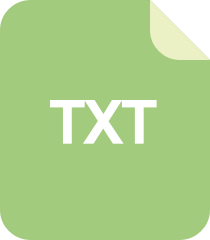
C# 如何判断一个Byte数组中是否存在某些连续的数据
在C#中,要处理byte数组并仅保留中间连续的零,可以按照以下步骤操作:
1. 定义一个函数来过滤连续的零:
```csharp
public static byte[] RemoveTrailingZeros(byte[] array)
{
int start = 0;
bool foundNonZero = false;
for (int i = 0; i < array.Length; i++)
{
if (array[i] != 0)
{
foundNonZero = true;
if (start < i) // 如果之前有非零元素,则更新起始位置
start = i;
}
}
if (!foundNonZero) // 如果数组全为零,返回空数组
return new byte[0];
Array.Resize(ref array, start + 1); // 缩小数组以去除多余零
return array;
}
```
2. 使用该函数过滤输入的byte数组:
```csharp
byte[] inputArray = { 1, 0, 0, 0, 2, 0, 0, 0, 3 }; // 假设这是你要处理的数组
byte[] filteredArray = RemoveTrailingZeros(inputArray);
```
这个函数会遍历数组,找到第一个非零元素的位置作为新的开始位置,并保持这个位置之后的元素不变。如果数组全为零,则返回一个新的空数组。
阅读全文
相关推荐
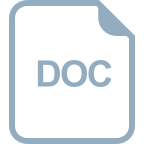
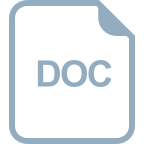
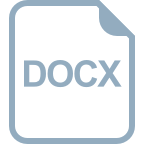
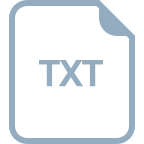
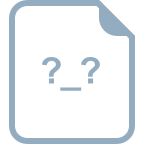
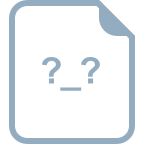
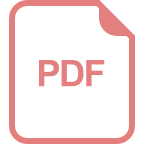
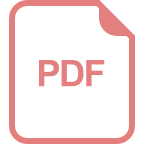
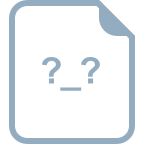
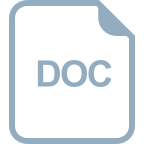
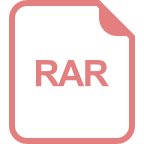
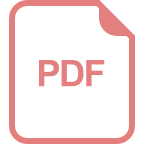
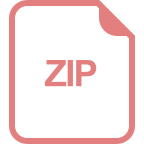
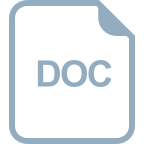
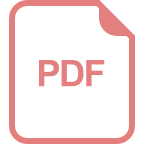
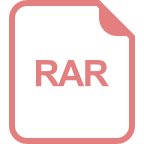
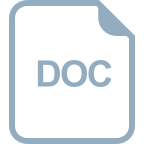