用c语言写顺序表的插入、删除、按位置查找元素、根据元素值在表中进行查找、求顺序表长的程序
时间: 2024-10-07 12:00:43 浏览: 35
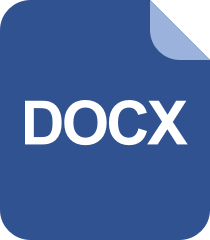
编写函数,建立一个顺序表并输出,计算该顺序表的长度。 实现在顺序表中查找第i个元素的值。实现在顺序表中第i个位置上插入值为x的

在C语言中,我们可以创建一个简单的顺序表结构(如数组实现),然后编写函数来执行插入、删除、查找和计算长度操作。以下是基本的代码示例:
```c
#include <stdio.h>
// 定义顺序表节点结构
typedef struct {
int data;
struct Node* next; // 指向下一个节点
} Node;
// 初始化顺序表的函数
Node* createList() {
return NULL;
}
// 插入元素到列表尾部的函数
void insert(int value, Node** head) {
Node* newNode = (Node*)malloc(sizeof(Node));
if (newNode != NULL) {
newNode->data = value;
newNode->next = *head;
*head = newNode;
}
else {
printf("Memory allocation failed.\n");
}
}
// 删除指定位置元素的函数
void deleteElement(int index, Node** head) {
if (*head == NULL || index < 0) {
return;
}
Node* temp = *head;
for (int i = 0; i < index && temp != NULL; i++) {
temp = temp->next;
}
if (temp == NULL) {
printf("Invalid index.\n");
} else {
Node* toDelete = temp->next;
*temp = temp->next;
free(toDelete);
}
}
// 查找指定位置的元素函数
int searchElement(int index, const Node* head) {
int count = 0;
Node* temp = head;
while (temp != NULL && count < index) {
temp = temp->next;
count++;
}
return temp ? temp->data : -1; // 如果找到返回数据,未找到则返回-1
}
// 计算顺序表长度的函数
int length(const Node* head) {
int count = 0;
Node* temp = head;
while (temp != NULL) {
count++;
temp = temp->next;
}
return count;
}
// 示例主函数
int main() {
Node* head = createList();
// 插入、删除和查找操作...
int listLength = length(head);
printf("List length: %d\n", listLength);
return 0;
}
```
阅读全文
相关推荐
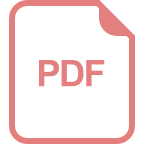
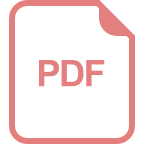















