用Keras写一个non local block,用def方法写,不要class
时间: 2023-08-31 09:31:28 浏览: 62
### 回答1:
好的,下面是一个使用 Keras 的 def 方法编写 non-local block 的示例:
```python
from keras.layers import Conv2D, MaxPooling2D, Add, Activation, Lambda
def non_local_block(input_x, inter_channel):
# 获取输入张量的维度
input_shape = input_x.shape
# 将输入张量进行转置操作,将通道维度放在最后一个维度
input_x = Lambda(lambda x: K.permute_dimensions(x, (0, 3, 1, 2)))(input_x)
# 计算输入张量的空间维度
spatial_shape = input_shape[1:3]
# 对输入张量进行 1x1 卷积降维,得到 theta、phi 和 g 三个张量
theta = Conv2D(inter_channel, kernel_size=1, strides=1, padding='same')(input_x)
phi = Conv2D(inter_channel, kernel_size=1, strides=1, padding='same')(input_x)
g = Conv2D(inter_channel, kernel_size=1, strides=1, padding='same')(input_x)
# 对 theta 和 phi 张量进行转置操作,将通道维度放在最后一个维度
theta = Lambda(lambda x: K.permute_dimensions(x, (0, 2, 3, 1)))(theta)
phi = Lambda(lambda x: K.permute_dimensions(x, (0, 2, 3, 1)))(phi)
# 计算 theta 和 phi 张量的内积,并将结果进行归一化
f = Lambda(lambda x: K.batch_dot(x[0], x[1], axes=[3, 3]))([theta, phi])
f = Activation('softmax')(f)
# 对 g 张量进行转置操作,将通道维度放在最后一个维度
g = Lambda(lambda x: K.permute_dimensions(x, (0, 2, 3, 1)))(g)
# 计算非局部块的输出张量
y = Lambda(lambda x: K.batch_dot(x[0], x[1], axes=[3, 2]))([f, g])
y = Lambda(lambda x: K.permute_dimensions(x, (0, 3, 1, 2)))(y)
y = Conv2D(input_shape[3], kernel_size=1, strides=1, padding='same')(y)
y = Add()([input_x, y])
# 返回非局部块的输出张量
return y
```
在这个实现中,我们首先将输入张量进行转置操作,将通道维度放在最后一个维度,然后对输入张量进行 1x1 卷积降维,得到 theta、phi 和 g 三个张量。接下来,我们对 theta 和 phi 张量进行转置操作,将通道维度放在最后一个维度,并计算它们的内积。然后,我们对 g 张量进行转置操作,将通道维度放在最后一个维度,并计算非局部块的输出张量。最后,我们将非局部块的输出张量与输入张量相加,得到最终的输出张量。
### 回答2:
在Keras中,可以使用def方法编写一个non-local block。非局部块是一种深度学习中的注意力机制,用于在图像或视频等数据中捕获长距离的依赖关系。
以下是一个使用def方法编写的non-local block代码示例:
```python
import tensorflow as tf
from tensorflow.keras.layers import Conv2D, BatchNormalization, Activation
def non_local_block(input_tensor):
input_shape = input_tensor.shape
# 计算输入特征图的维度
num_channels = input_shape[-1]
# 计算输入特征图的尺寸
img_height = input_shape[1]
img_width = input_shape[2]
# 将输入特征图进行重新构造,每个像素变为一个维度为num_channels的特征向量
reshaped_input = tf.reshape(input_tensor, [-1, img_height*img_width, num_channels])
# 计算注意力权重
attention = tf.keras.layers.Conv1D(filters=num_channels, kernel_size=1, activation='relu')(reshaped_input)
attention = tf.keras.layers.Conv1D(filters=num_channels, kernel_size=1, activation='sigmoid')(attention)
# 将注意力权重与输入特征图相乘得到加权特征图
weighted_features = tf.keras.layers.Multiply()([attention, reshaped_input])
# 将加权特征图进行相加并重构为原始形状
output = tf.keras.layers.Add()([input_tensor, tf.reshape(weighted_features, tf.shape(input_tensor))])
return output
```
在这个示例中,我们首先通过tf.reshape重新构造输入特征图,然后将其输入到两个1D卷积层中,分别用于计算注意力权重。然后,我们使用tf.keras.layers.Multiply()将注意力权重与输入特征图相乘,得到加权特征图。最后,我们将加权特征图与输入特征图进行相加,并使用tf.keras.layers.Add()将结果重构为原始形状。
这样,我们就成功地编写了一个非局部块的Keras实现。你可以根据自己的需求将该non local block应用于自己的模型中。
阅读全文
相关推荐
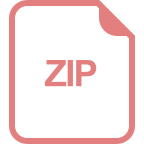
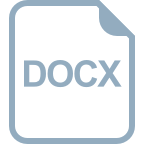

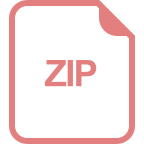
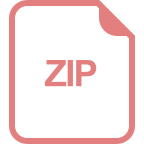
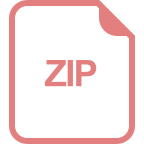
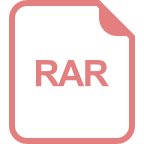
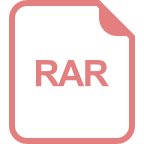
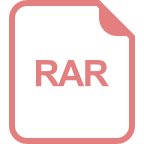
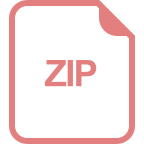
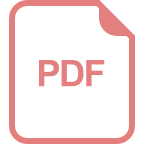
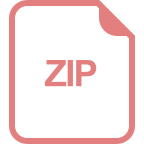
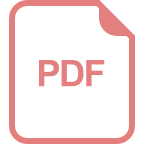
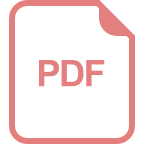