设计并实现一个具有图形用户界面的文本文件加密工具
时间: 2024-05-08 16:20:33 浏览: 282
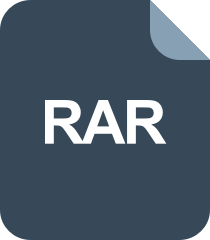
文本加密工具
为了实现这个文本文件加密工具,我们需要以下几个步骤:
1. 设计图形用户界面,包括选择文件、输入密码、加密/解密等功能。
2. 使用 Python 语言编写加密算法,比如 AES 或者 DES。
3. 实现文件读取和写入功能,可以使用 Python 内置的文件操作函数来完成。
4. 将界面和算法进行整合,实现加密和解密功能。
下面我们逐步具体实现:
1. 设计图形用户界面
我们可以使用 Python 的 Tkinter 库来实现图形用户界面。具体实现可以参考以下代码:
```python
import tkinter as tk
from tkinter import filedialog
from Crypto.Cipher import AES
class TextEncryptionTool:
def __init__(self, master):
self.master = master
self.master.title("Text Encryption Tool")
self.file_path = ""
self.password = ""
# 文件选择框
self.file_label = tk.Label(self.master, text="请选择需要加密/解密的文件:")
self.file_label.grid(row=0, column=0)
self.file_entry = tk.Entry(self.master, width=50)
self.file_entry.grid(row=0, column=1)
self.file_button = tk.Button(self.master, text="选择文件", command=self.select_file)
self.file_button.grid(row=0, column=2)
# 密码框
self.password_label = tk.Label(self.master, text="请输入密码:")
self.password_label.grid(row=1, column=0)
self.password_entry = tk.Entry(self.master, width=50, show="*")
self.password_entry.grid(row=1, column=1)
# 加密/解密按钮
self.encrypt_button = tk.Button(self.master, text="加密", command=self.encrypt)
self.encrypt_button.grid(row=2, column=0)
self.decrypt_button = tk.Button(self.master, text="解密", command=self.decrypt)
self.decrypt_button.grid(row=2, column=1)
def select_file(self):
self.file_path = filedialog.askopenfilename()
self.file_entry.delete(0, tk.END)
self.file_entry.insert(0, self.file_path)
def encrypt(self):
self.password = self.password_entry.get()
with open(self.file_path, "rb") as f:
plaintext = f.read()
cipher = AES.new(self.password.encode(), AES.MODE_EAX)
ciphertext, tag = cipher.encrypt_and_digest(plaintext)
with open(self.file_path + ".enc", "wb") as f:
[f.write(x) for x in (cipher.nonce, tag, ciphertext)]
def decrypt(self):
self.password = self.password_entry.get()
with open(self.file_path, "rb") as f:
nonce, tag, ciphertext = [f.read(x) for x in (16, 16, -1)]
cipher = AES.new(self.password.encode(), AES.MODE_EAX, nonce)
plaintext = cipher.decrypt_and_verify(ciphertext, tag)
with open(self.file_path.replace(".enc", ""), "wb") as f:
f.write(plaintext)
if __name__ == "__main__":
root = tk.Tk()
app = TextEncryptionTool(root)
root.mainloop()
```
2. 使用 Python 语言编写加密算法
我们可以使用 PyCryptodome 库来实现 AES 算法的加密和解密。具体实现可以参考以下代码:
```python
from Crypto.Cipher import AES
def encrypt(plaintext, password):
cipher = AES.new(password.encode(), AES.MODE_EAX)
ciphertext, tag = cipher.encrypt_and_digest(plaintext)
return [cipher.nonce, tag, ciphertext]
def decrypt(nonce, tag, ciphertext, password):
cipher = AES.new(password.encode(), AES.MODE_EAX, nonce)
plaintext = cipher.decrypt_and_verify(ciphertext, tag)
return plaintext
```
3. 实现文件读取和写入功能
我们可以使用 Python 内置的文件操作函数来实现文件的读取和写入。具体实现可以参考以下代码:
```python
def read_file(file_path):
with open(file_path, "rb") as f:
return f.read()
def write_file(file_path, data):
with open(file_path, "wb") as f:
f.write(data)
```
4. 整合界面和算法
我们可以在加密和解密函数中调用上述的加密和解密算法以及文件读取和写入函数,实现加密和解密功能。具体实现可以参考以下代码:
```python
def encrypt_file(file_path, password):
plaintext = read_file(file_path)
ciphertext = encrypt(plaintext, password)
write_file(file_path + ".enc", b"".join(ciphertext))
def decrypt_file(file_path, password):
ciphertext = read_file(file_path)
nonce, tag, ciphertext = ciphertext[:16], ciphertext[16:32], ciphertext[32:]
plaintext = decrypt(nonce, tag, ciphertext, password)
write_file(file_path.replace(".enc", ""), plaintext)
```
完整代码如下:
阅读全文
相关推荐



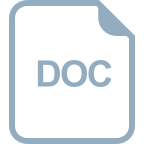
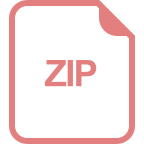
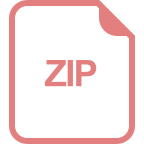
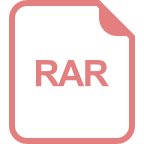
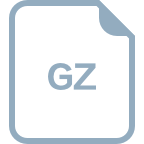
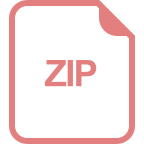
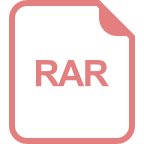
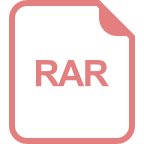
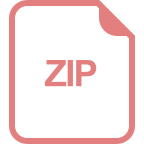
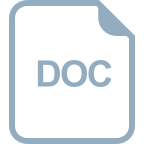
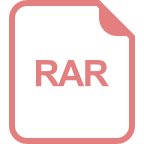
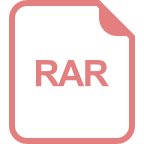
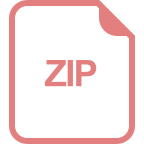