用qt写一段socket代理层的代码实现两个上位机同时连接一个下位机,并将下位机的数据呈现在两台上位机上
时间: 2024-03-20 11:43:29 浏览: 68
好的,我可以为您提供一份简单的 Qt Socket 代理层的代码,实现两个上位机同时连接一个下位机,并将下位机的数据呈现在两台上位机上。以下是代码示例:
```cpp
#include <QtNetwork>
#include <QtCore>
class Proxy : public QObject
{
Q_OBJECT
public:
Proxy(QObject *parent = nullptr)
: QObject(parent)
, m_downstreamSocket(new QTcpSocket(this))
{
// Connect downstreamSocket signals
connect(m_downstreamSocket, &QTcpSocket::readyRead, this, &Proxy::onDownstreamReadyRead);
connect(m_downstreamSocket, &QTcpSocket::disconnected, this, &Proxy::onDownstreamDisconnected);
}
void setDownstreamHost(const QString &host, quint16 port)
{
m_downstreamHost = host;
m_downstreamPort = port;
}
void addUpstreamConnection(QTcpSocket *socket)
{
// Connect upstreamSocket signals
connect(socket, &QTcpSocket::readyRead, this, &Proxy::onUpstreamReadyRead);
connect(socket, &QTcpSocket::disconnected, this, &Proxy::onUpstreamDisconnected);
// Add the upstream socket to the list
m_upstreamSockets.append(socket);
// If this is the first upstream socket, connect to the downstream host
if (m_upstreamSockets.count() == 1) {
m_downstreamSocket->connectToHost(m_downstreamHost, m_downstreamPort);
}
}
private slots:
void onDownstreamReadyRead()
{
// Forward the downstream data to all upstream sockets
for (QTcpSocket *socket : qAsConst(m_upstreamSockets)) {
socket->write(m_downstreamSocket->readAll());
}
}
void onDownstreamDisconnected()
{
// Disconnect all upstream sockets
for (QTcpSocket *socket : qAsConst(m_upstreamSockets)) {
socket->disconnectFromHost();
}
}
void onUpstreamReadyRead()
{
// Forward the upstream data to the downstream socket
QTcpSocket *socket = qobject_cast<QTcpSocket*>(sender());
if (socket) {
m_downstreamSocket->write(socket->readAll());
}
}
void onUpstreamDisconnected()
{
// Remove the upstream socket from the list
QTcpSocket *socket = qobject_cast<QTcpSocket*>(sender());
if (socket) {
m_upstreamSockets.removeOne(socket);
}
// If there are no more upstream sockets, disconnect from the downstream host
if (m_upstreamSockets.isEmpty()) {
m_downstreamSocket->disconnectFromHost();
}
}
private:
QString m_downstreamHost;
quint16 m_downstreamPort;
QTcpSocket *m_downstreamSocket;
QList<QTcpSocket*> m_upstreamSockets;
};
```
这个代理层有一个下游套接字 `m_downstreamSocket` 和多个上游套接字 `m_upstreamSockets`。当下游套接字连接到下位机时,所有的上游套接字都将连接到代理层。当下游套接字收到数据时,代理层将数据转发到所有上游套接字。当一个上游套接字断开连接时,它将从上游套接字列表中删除。如果没有上游套接字,代理层将断开与下位机的连接。
要使用此代理层,请在您的主应用程序中创建一个 `Proxy` 对象,并使用 `setDownstreamHost()` 设置下游主机和端口。每当您的应用程序接受一个新的上游套接字时,调用 `addUpstreamConnection()` 将其添加到代理层中。
希望这可以帮助您完成您的项目!
阅读全文
相关推荐
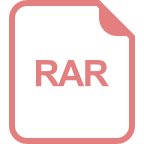



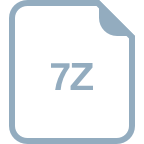
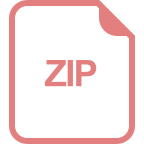
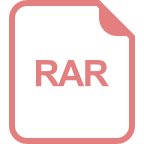