使用java编写财务信息类,输入公司名称、定金收入、押金收入、租金收入、其它收入、员工成本、房租、水电、税收及其它费用,输出收入计算、成本计算、净收入计算。
时间: 2023-09-23 22:11:31 浏览: 55
可以使用以下代码实现:
```
public class FinancialInfo {
private String companyName;
private double downPaymentIncome;
private double depositIncome;
private double rentIncome;
private double otherIncome;
private double employeeCosts;
private double rentCost;
private double utilitiesCost;
private double taxCost;
private double otherCosts;
public FinancialInfo(String companyName, double downPaymentIncome, double depositIncome, double rentIncome, double otherIncome, double employeeCosts, double rentCost, double utilitiesCost, double taxCost, double otherCosts) {
this.companyName = companyName;
this.downPaymentIncome = downPaymentIncome;
this.depositIncome = depositIncome;
this.rentIncome = rentIncome;
this.otherIncome = otherIncome;
this.employeeCosts = employeeCosts;
this.rentCost = rentCost;
this.utilitiesCost = utilitiesCost;
this.taxCost = taxCost;
this.otherCosts = otherCosts;
}
public void printIncomeCalculation() {
System.out.println("Income Calculation for " + companyName);
System.out.println("Down Payment Income: " + downPaymentIncome);
System.out.println("Deposit Income: " + depositIncome);
System.out.println("Rent Income: " + rentIncome);
System.out.println("Other Income: " + otherIncome);
double totalIncome = downPaymentIncome + depositIncome + rentIncome + otherIncome;
System.out.println("Total Income: " + totalIncome);
}
public void printCostCalculation() {
System.out.println("Cost Calculation for " + companyName);
System.out.println("Employee Costs: " + employeeCosts);
System.out.println("Rent Costs: " + rentCost);
System.out.println("Utilities Costs: " + utilitiesCost);
System.out.println("Tax Costs: " + taxCost);
System.out.println("Other Costs: " + otherCosts);
double totalCosts = employeeCosts + rentCost + utilitiesCost + taxCost + otherCosts;
System.out.println("Total Costs: " + totalCosts);
}
public void printNetIncomeCalculation() {
System.out.println("Net Income Calculation for " + companyName);
double totalIncome = downPaymentIncome + depositIncome + rentIncome + otherIncome;
double totalCosts = employeeCosts + rentCost + utilitiesCost + taxCost + otherCosts;
double netIncome = totalIncome - totalCosts;
System.out.println("Net Income: " + netIncome);
}
}
```
然后,可以在主函数中创建一个实例并调用相应的方法:
```
public static void main(String[] args) {
FinancialInfo financialInfo = new FinancialInfo("ABC Company", 5000.0, 2000.0, 10000.0, 500.0, 8000.0, 3000.0, 1000.0, 2000.0, 500.0);
financialInfo.printIncomeCalculation();
financialInfo.printCostCalculation();
financialInfo.printNetIncomeCalculation();
}
```
输出结果如下:
```
Income Calculation for ABC Company
Down Payment Income: 5000.0
Deposit Income: 2000.0
Rent Income: 10000.0
Other Income: 500.0
Total Income: 17500.0
Cost Calculation for ABC Company
Employee Costs: 8000.0
Rent Costs: 3000.0
Utilities Costs: 1000.0
Tax Costs: 2000.0
Other Costs: 500.0
Total Costs: 14500.0
Net Income Calculation for ABC Company
Net Income: 3000.0
```
相关推荐
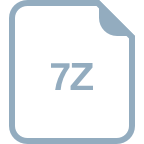
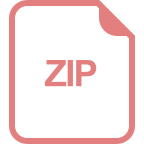
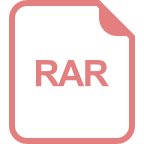














