写一个五子棋游戏的代码
时间: 2023-05-17 07:05:11 浏览: 66
以下是一个简单的五子棋游戏的代码示例:
```python
import numpy as np
# 定义棋盘大小
BOARD_SIZE = 15
# 定义棋子类型
EMPTY = 0
BLACK = 1
WHITE = 2
class Gobang:
def __init__(self):
self.board = np.zeros((BOARD_SIZE, BOARD_SIZE), dtype=int)
self.current_player = BLACK
def play(self, x, y):
if self.board[x][y] != EMPTY:
return False
self.board[x][y] = self.current_player
if self.check_win(x, y):
print("Player {} wins!".format(self.current_player))
return True
self.current_player = BLACK if self.current_player == WHITE else WHITE
return False
def check_win(self, x, y):
# 检查行
count = 1
for i in range(1, 5):
if y - i < 0 or self.board[x][y - i] != self.current_player:
break
count += 1
for i in range(1, 5):
if y + i >= BOARD_SIZE or self.board[x][y + i] != self.current_player:
break
count += 1
if count >= 5:
return True
# 检查列
count = 1
for i in range(1, 5):
if x - i < 0 or self.board[x - i][y] != self.current_player:
break
count += 1
for i in range(1, 5):
if x + i >= BOARD_SIZE or self.board[x + i][y] != self.current_player:
break
count += 1
if count >= 5:
return True
# 检查对角线
count = 1
for i in range(1, 5):
if x - i < 0 or y - i < 0 or self.board[x - i][y - i] != self.current_player:
break
count += 1
for i in range(1, 5):
if x + i >= BOARD_SIZE or y + i >= BOARD_SIZE or self.board[x + i][y + i] != self.current_player:
break
count += 1
if count >= 5:
return True
# 检查反对角线
count = 1
for i in range(1, 5):
if x - i < 0 or y + i >= BOARD_SIZE or self.board[x - i][y + i] != self.current_player:
break
count += 1
for i in range(1, 5):
if x + i >= BOARD_SIZE or y - i < 0 or self.board[x + i][y - i] != self.current_player:
break
count += 1
if count >= 5:
return True
return False
```
你可以通过创建一个 Gobang 实例来开始游戏,例如:
```python
game = Gobang()
game.play(7, 7) # 黑方下在中心位置
game.play(7, 8) # 白方下在中心偏上位置
game.play(6, 7) # 黑方下在中心偏左位置
# ...
```
请注意,这只是一个简单的示例,实际的五子棋游戏可能需要更复杂的算法来实现 AI 玩家。
相关推荐
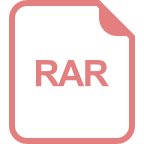
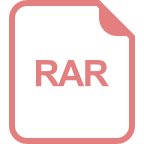
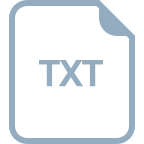













