pytorch框架孤立森林算法对齿轮箱多通道异常诊断代码
时间: 2023-11-30 19:04:29 浏览: 86
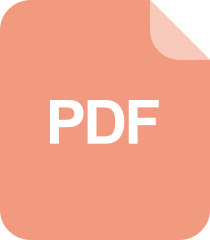
基于多粒度级联孤立森林算法的异常检测模型
下面是一个使用 PyTorch 实现孤立森林算法来进行多通道齿轮箱异常诊断的代码示例:
首先,我们需要导入必要的库:
```python
import numpy as np
import pandas as pd
import torch
import torch.nn as nn
import torch.optim as optim
from sklearn.ensemble import IsolationForest
from sklearn.model_selection import train_test_split
```
然后,我们可以加载数据集并进行预处理:
```python
# 加载数据集
data = pd.read_csv('gearbox_data.csv')
# 将标签列转换为二进制值
data['Status'] = np.where(data['Status']=='Normal', 0, 1)
# 将数据分为特征和标签
X = data.drop(['Status'], axis=1).values
y = data['Status'].values
# 划分训练集和测试集
X_train, X_test, y_train, y_test = train_test_split(X, y, test_size=0.2, random_state=42)
```
接下来,我们可以使用 `IsolationForest` 算法来训练模型:
```python
# 训练 IsolationForest 模型
clf = IsolationForest(n_estimators=100, contamination=0.1, random_state=42)
clf.fit(X_train, y_train)
# 在测试集上进行预测
y_pred = clf.predict(X_test)
```
最后,我们可以计算模型的性能指标(如精度、召回率、F1 分数等):
```python
# 计算模型的性能指标
tp = np.sum((y_test == 1) & (y_pred == -1))
fp = np.sum((y_test == 0) & (y_pred == -1))
tn = np.sum((y_test == 0) & (y_pred == 1))
fn = np.sum((y_test == 1) & (y_pred == 1))
accuracy = (tp + tn) / (tp + fp + tn + fn)
precision = tp / (tp + fp)
recall = tp / (tp + fn)
f1_score = 2 * precision * recall / (precision + recall)
print('Accuracy:', accuracy)
print('Precision:', precision)
print('Recall:', recall)
print('F1 score:', f1_score)
```
这就是一个简单的使用 PyTorch 实现孤立森林算法来进行多通道齿轮箱异常诊断的代码示例。请注意,这只是一个简单的示例,实际的代码可能需要进行更多的调整和优化才能得到最佳的性能。
阅读全文
相关推荐
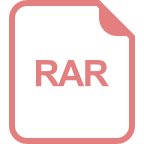

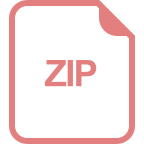
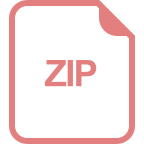
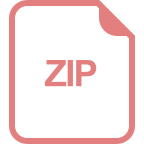
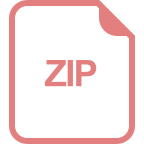
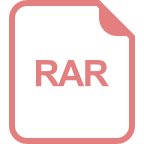
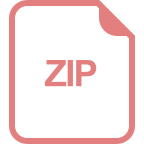
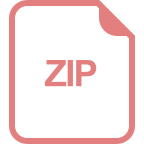
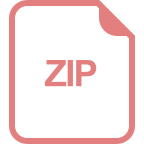
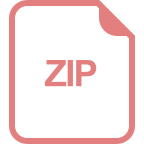
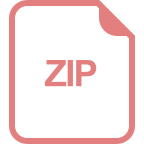
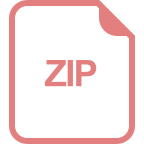
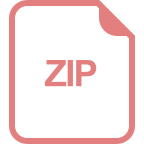
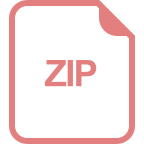