使用matplotlib库来可视化迪杰斯特拉算法的最短路径
时间: 2023-02-28 12:54:13 浏览: 68
可以使用Matplotlib库来可视化迪杰斯特拉算法的最短路径。具体的,需要首先定义图形,然后通过运行算法计算出最短路径,最后使用Matplotlib库绘制图形并显示最短路径。
首先,需要导入Matplotlib库,然后使用函数`plot()`绘制图形。接下来,可以使用函数`annotate()`在图形上添加标签,以显示节点间的距离。最后,可以使用函数`show()`显示图形。
以下是一个简单的示例:
```
import matplotlib.pyplot as plt
# 定义节点坐标
x = [0, 1, 2, 3]
y = [0, 1, 2, 3]
# 绘制图形
plt.plot(x, y, 'ro')
# 添加标签
plt.annotate("A", (x[0], y[0]))
plt.annotate("B", (x[1], y[1]))
plt.annotate("C", (x[2], y[2]))
plt.annotate("D", (x[3], y[3]))
# 显示图形
plt.show()
```
这只是一个简单的示例,实际上可以根据需要使用更复杂的绘图语法和功能进行定制。
相关问题
使用Matplotlib库对分类结果进行可视化展示
假设我们有以下分类结果:
```python
import numpy as np
from sklearn.datasets import make_classification
from sklearn.linear_model import LogisticRegression
# 生成数据集
X, y = make_classification(n_features=2, n_redundant=0, n_informative=1, n_clusters_per_class=1, random_state=42)
# 训练分类器
clf = LogisticRegression(random_state=42)
clf.fit(X, y)
# 预测分类结果
y_pred = clf.predict(X)
```
我们可以使用Matplotlib库将这个分类结果可视化展示出来。
```python
import matplotlib.pyplot as plt
# 绘制散点图,用不同的颜色表示不同的分类
plt.scatter(X[:, 0], X[:, 1], c=y_pred)
plt.title('Classification Result')
plt.xlabel('Feature 1')
plt.ylabel('Feature 2')
plt.show()
```
运行上述代码,就可以得到一个简单的散点图,其中不同的颜色表示不同的分类。
如何使用Matplotlib实现数据可视化在pygame实现
要在pygame中使用Matplotlib实现数据可视化,需要按照以下步骤进行操作:
1. 安装Matplotlib库和pygame库:
首先,需要确保已经在Python环境中安装了Matplotlib库和pygame库。可以使用以下命令安装这些库:
```
pip install matplotlib
pip install pygame
```
2. 创建pygame窗口:
使用pygame库创建一个窗口,可以使用以下代码:
```
import pygame
# 初始化pygame
pygame.init()
# 创建窗口
screen = pygame.display.set_mode((640, 480))
# 游戏循环
while True:
# 处理事件
for event in pygame.event.get():
if event.type == pygame.QUIT:
# 退出游戏
pygame.quit()
sys.exit()
# 刷新屏幕
pygame.display.update()
```
这个代码会创建一个640x480的窗口,并启动一个游戏循环,可以用于展示数据可视化效果。
3. 使用Matplotlib绘制图形:
然后,使用Matplotlib库绘制所需的图形。一般来说,可以使用Matplotlib绘制直方图、折线图、散点图等。例如,以下代码可以绘制一个简单的散点图:
```
import numpy as np
import matplotlib.pyplot as plt
# 生成随机数据
x = np.random.normal(size=100)
y = np.random.normal(size=100)
# 绘制散点图
plt.scatter(x, y)
plt.show()
```
4. 将Matplotlib图形绘制到pygame窗口中:
最后,在pygame的游戏循环中,将Matplotlib绘制的图形转换成一个pygame Surface,并将其显示在pygame窗口中。可以使用以下代码将Matplotlib图形转换成pygame Surface:
```
import pygame
import matplotlib.pyplot as plt
from matplotlib.backends.backend_agg import FigureCanvasAgg as FigureCanvas
from matplotlib.figure import Figure
# 创建Matplotlib图形
fig = Figure()
ax = fig.add_subplot(111)
ax.plot([1, 2, 3], [4, 5, 6])
# 将Matplotlib图形转换成pygame Surface
canvas = FigureCanvas(fig)
canvas.draw()
renderer = canvas.get_renderer()
raw_data = renderer.tostring_rgb()
# 将Surface显示在pygame窗口中
surf = pygame.image.fromstring(raw_data, fig.canvas.get_width_height(), "RGB")
screen.blit(surf, (0, 0))
pygame.display.update()
```
这个代码可以将Matplotlib图形绘制到pygame窗口中,实现数据可视化的效果。
完整的实现代码如下:
```
import pygame
import numpy as np
import matplotlib.pyplot as plt
from matplotlib.backends.backend_agg import FigureCanvasAgg as FigureCanvas
from matplotlib.figure import Figure
# 初始化pygame
pygame.init()
# 创建窗口
screen = pygame.display.set_mode((640, 480))
# 创建Matplotlib图形
fig = Figure()
ax = fig.add_subplot(111)
ax.plot([1, 2, 3], [4, 5, 6])
# 游戏循环
while True:
# 处理事件
for event in pygame.event.get():
if event.type == pygame.QUIT:
# 退出游戏
pygame.quit()
sys.exit()
# 将Matplotlib图形转换成pygame Surface
canvas = FigureCanvas(fig)
canvas.draw()
renderer = canvas.get_renderer()
raw_data = renderer.tostring_rgb()
# 将Surface显示在pygame窗口中
surf = pygame.image.fromstring(raw_data, fig.canvas.get_width_height(), "RGB")
screen.blit(surf, (0, 0))
pygame.display.update()
```
这个代码可以在pygame窗口中显示一个由Matplotlib绘制的简单图形。可以根据需要修改Matplotlib代码,以生成不同类型的图形。
相关推荐
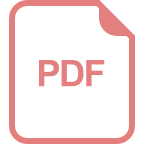
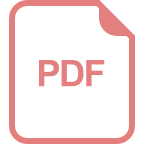
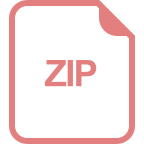












