利用python爬虫,提取C:/Users/test/Desktop/DIDItest文件夹下多个文件内的html文件源代码,并提取源代码中的ID、时间、发送号码、接收号码、发送内容,如果发送内容为音频则提取音频所在位置,反之则保留发送内容,并将爬取的内容写入csv中 其中一个网页内源代码模板如下: <html> <meta http-equiv="Content=-Type" content="text/html; charset=utf-8"> <head> <link href="http://192.167.1.101/hint.css" rel="stylesheet"></link> </head> <body>[talkid:138031361]2014年4月20日 03:55:45 , <span class="hint-success" data-hint"">434343</span> 向 <span class="hint-success" data-hint="">232323</span> 发送 我们已经是好友了,开始聊天吧! <p> ()</p> [talkid:138031362]2014年4月20日 04:45:45 , <span class="hint-success" data-hint"">434343</span> 向 <span class="hint-success" data-hint="">123456</span> 发送 音频 :[<ahref="files/f/f123fsasfsfsjdfrhf_n.m4a"]>音频</a> <p> ()</p> [talkid:138031363]2014年4月20日 04:55:45 , <span class="hint-success" data-hint"">434343</span> 向 <span class="hint-success" data-hint="">123456</span> 发送 音频 :[<ahref="files/f/f123fsasfsfsjdfrhf_n.jpg"]>图片</a> <p> ()</p> [talkid:138031364]2014年4月20日 05:55:45 , <span class="hint-success" data-hint"">434343</span> 向 <span class="hint-success" data-hint="">3234221</span> 发送 我们已经是好友了,开始聊天吧! <p> ()</p> [talkid:138031365]2014年4月20日 06:55:45 , <span class="hint-success" data-hint"">434343</span> 向 <span class="hint-success" data-hint="">1359075</span> 发送 我们已经是好友了,开始聊天吧! <p> ()</p> </body> </html>
时间: 2023-08-14 14:58:26 浏览: 104
你可以使用Python的爬虫库BeautifulSoup和csv库来提取文件夹中的HTML文件源代码,并从源代码中提取所需的信息。以下是一个示例代码,可以满足你的需求:
```python
import os
import csv
from bs4 import BeautifulSoup
folder_path = 'C:/Users/test/Desktop/DIDItest'
output_file = 'output.csv'
# 定义要提取的字段
fields = ['ID', '时间', '发送号码', '接收号码', '发送内容']
# 创建CSV文件并写入字段名
with open(output_file, 'w', newline='', encoding='utf-8') as file:
writer = csv.DictWriter(file, fieldnames=fields)
writer.writeheader()
# 遍历文件夹中的HTML文件
for filename in os.listdir(folder_path):
if filename.endswith('.html'):
file_path = os.path.join(folder_path, filename)
# 打开HTML文件并解析源代码
with open(file_path, 'r', encoding='utf-8') as html_file:
soup = BeautifulSoup(html_file, 'html.parser')
# 提取所需的信息
for talkid in soup.find_all('span', class_='hint-success'):
info = {}
info['ID'] = talkid['talkid']
info['时间'] = talkid.next_sibling.strip().split(',')[0]
info['发送号码'] = talkid.get_text()
info['接收号码'] = talkid.find_next('span', class_='hint-success').get_text()
info['发送内容'] = talkid.find_next('p').next_sibling.strip()
# 如果发送内容为音频,则提取音频所在位置
if '音频' in info['发送内容']:
audio_link = talkid.find_next('a')['href']
audio_path = os.path.join(folder_path, audio_link)
info['发送内容'] = audio_path
# 写入CSV文件
writer.writerow(info)
```
你只需要将`folder_path`变量设置为你的文件夹路径,并将`output_file`变量设置为你想要保存结果的CSV文件路径。这个代码将遍历文件夹中的所有HTML文件,提取所需的信息,并将结果写入CSV文件中。如果发送内容是音频,则提取音频所在位置,否则保留发送内容。
请确保安装了BeautifulSoup库,你可以使用`pip install beautifulsoup4`命令进行安装。
阅读全文
相关推荐




网页内源代码模板如下: <html> <meta http-equiv="Content=-Type" content="text/html; charset=utf-8"> <head> </head> <body>[talkid:138031370]2014年4月20日 03:55:45 , 111222 向 232323 发送 我们已经是好友了,开始聊天吧! () [talkid:138031371]2014年4月20日 04:45:45 , 111222 向 123456 发送 音频 :[<ahref="files/f/f123fsasfsfsjdfrhf_n.m4a"]>音频
() [talkid:138031372]2014年4月20日 04:55:45 , 111222 向 123456 发送 图片 :[<ahref="files/f/f123fsasfsfsjdfrhf_n.jpg"]>图片 () </body> </html> 利用python爬虫,打开C:/Users/test/Desktop/DIDItest文件夹下多个文件夹内的html文件源代码,并爬取源代码中的ID、时间、发送号码、接收号码、发送内容,如果发送内容不为文本,则提取文件所在链接地址,并将爬取的内容写入csv中
网页内源代码模板如下: <html> <meta http-equiv="Content=-Type" content="text/html; charset=utf-8"> <head> </head> <body>[talkid:138031370]2014年4月20日 03:55:45 , 111222 向 232323 发送 我们已经是好友了,开始聊天吧! () [talkid:138031371]2014年4月20日 04:45:45 , 111222 向 123456 发送 音频 :[<ahref="files/f/f123fsasfsfsjdfrhf_n.m4a"]>音频
() [talkid:138031372]2014年4月20日 04:55:45 , 111222 向 123456 发送 图片 :[<ahref="files/f/f123fsasfsfsjdfrhf_n.jpg"]>图片 () </body> </html> 利用python爬虫,打开C:/Users/test/Desktop/DIDItest文件夹下多个文件夹内的html文件源代码,并将源代码转换为字符串,爬取源代码字符串中的ID、时间、发送号码、接收号码、信息类型、发送内容,如果发送内容不为文本,则提取文件所在链接地址,并将爬取的内容写入csv中,talkid提取[]中talkid:后的数字、时间精确至年月日时分秒、发送号码提取第一个 data-hint"">之间的数字,接收号码提取第二个data-hint"">,信息类型就提取 发送与:之间的文字,如果没有:则定义为文字
利用python爬虫,提取C:/Users/test/Desktop/DIDItest文件夹下多个文件内的html文件源代码,并提取源代码中的ID、时间、发送号码、接收号码、发送内容,如果发送内容为音频则提取音频所在位置,反之则保留发送内容,并将爬取的内容写入csv中 网页内源代码如下: <html> <meta http-equiv="Content=-Type" content="text/html; charset=utf-8"> <head> </head> <body>[talkid:138031361]2014年4月20日 03:55:45 , 434343 向 232323 发送 我们已经是好友了,开始聊天吧! () [talkid:138031362]2014年4月20日 04:45:45 , 434343 向 123456 发送 音频 :[<ahref="files/f/f123fsasfsfsjdfrhf_n.m4a"]>音频
() [talkid:138031363]2014年4月20日 04:55:45 , 434343 向 123456 发送 音频 :[<ahref="files/f/f123fsasfsfsjdfrhf_n.jpg"]>图片 () [talkid:138031364]2014年4月20日 05:55:45 , 434343 向 3234221 发送 我们已经是好友了,开始聊天吧! () [talkid:138031365]2014年4月20日 06:55:45 , 434343 向 1359075 发送 我们已经是好友了,开始聊天吧! () </body> </html>
利用python爬虫,提取C:/Users/test/Desktop/DIDItest文件夹下每个文件夹内的html文件源代码,并提取源代码中的ID、时间、发送号码、接收号码、发送内容,如果发送内容为音频则提取音频所在位置,反之则保留发送内容,并将爬取的内容写入csv中 网页内源代码如下: <html> <meta http-equiv="Content=-Type" content="text/html; charset=utf-8"> <head> </head> <body>[talkid:138031361]2014年4月20日 03:55:45 , 434343 向 232323 发送 我们已经是好友了,开始聊天吧! () [talkid:138031362]2014年4月20日 04:45:45 , 434343 向 123456 发送 音频 :[<ahref="files/f/f123fsasfsfsjdfrhf_n.m4a"]>音频
() [talkid:138031363]2014年4月20日 04:55:45 , 434343 向 123456 发送 音频 :[<ahref="files/f/f123fsasfsfsjdfrhf_n.jpg"]>图片 () [talkid:138031364]2014年4月20日 05:55:45 , 434343 向 3234221 发送 我们已经是好友了,开始聊天吧! () [talkid:138031365]2014年4月20日 06:55:45 , 434343 向 1359075 发送 我们已经是好友了,开始聊天吧! () </body> </html>
<html> <meta http-equiv="Content=-Type" content="text/html; charset=utf-8"> <head> </head> <body>[talkid:138031370]2014年4月20日 03:55:45 , 111222 向 232323 发送 我们已经是好友了,开始聊天吧! () [talkid:138031371]2014年4月20日 04:45:45 , 111222 向 123456 发送 音频 :[<ahref="files/f/f123fsasfsfsjdfrhf_n.m4a"]>音频
() [talkid:138031372]2014年4月20日 04:55:45 , 111222 向 123456 发送 图片 :[<ahref="files/f/f123fsasfsfsjdfrhf_n.jpg"]>图片 () [talkid:138031373]2014年4月20日 05:55:45 , 111222 向 3234221 发送 我们已经是好友了,开始聊天吧! () [talkid:138031374]2014年4月20日 06:55:45 , 111222 向 1359075 发送 我们已经是好友了,开始聊天吧! () </body> </html>利用python爬虫,打开C:/Users/test/Desktop/DIDItest文件夹下多个文件夹内的html文件源代码,并爬取源代码中的ID、时间、发送号码、接收号码、发送内容,如果发送内容不为文本,则提取文件所在链接地址,并将爬取的内容写入csv中
网页内源代码模板如下: <body> [talkid:138031370]2014年4月20日 03:55:45 , 111222 向 232323 发送 我们已经是好友了,开始聊天吧! () [talkid:138031371]2014年4月20日 04:45:45 , 111222 向 123456 发送 音频 :[音频 () [talkid:138031372]2014年4月20日 04:55:45 , 111222 向 123456 发送 图片 :[图片 () [talkid:137031382]2014年4月22日 04:45:45 , 2323234 在群 20011 中发言 音频 :[音频 () [talkid:137031383]2014年4月23日 04:55:45 , 2323234 在群 20011 中发言 图片 :[图片 () [talkid:137031384]2014年4月24日 05:55:45 , 2323234 在群 20011 中发言 我们已经是好友了,开始聊天吧! () </body> </html> 利用python爬虫,打开C:/Users/test/Desktop/DIDItest文件夹下所有文件夹内的html文件源代码,并提取每一行<body>之间的数据,剔除 ()

网页内源代码模板如下: <body> [talkid:138031370]2014年4月20日 03:55:45 , 111222 向 232323 发送 我们已经是好友了,开始聊天吧! () [talkid:138031371]2014年4月20日 04:45:45 , 111222 向 123456 发送 音频 :[音频 () [talkid:138031372]2014年4月20日 04:55:45 , 111222 向 123456 发送 图片 :[图片 () [talkid:137031382]2014年4月22日 04:45:45 , 2323234 在群 20011 中发言 音频 :[音频 () [talkid:137031383]2014年4月23日 04:55:45 , 2323234 在群 20011 中发言 图片 :[图片 () [talkid:137031384]2014年4月24日 05:55:45 , 2323234 在群 20011 中发言 我们已经是好友了,开始聊天吧! () </body> </html> 利用python爬虫,打开C:/Users/test/Desktop/DIDItest文件夹下所有文件夹内的html文件源代码,



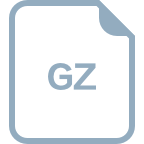