vue控制一个图片随机移动,首先先生成下一次图片的位置,然后慢慢移动过去,并且能看到移动轨迹(虚线),请给我写个详细的例子
时间: 2024-02-21 22:59:24 浏览: 106
好的,以下是一个基于Vue的随机移动图片的例子:
HTML部分:
```html
<div id="app">
<div class="container">
<img class="moving-img" :src="imgSrc" :style="imgStyle" />
<svg class="moving-path" :width="pathWidth" :height="pathHeight">
<path :d="path" stroke-dasharray="5,5" stroke="#ccc" fill="none"></path>
</svg>
</div>
</div>
```
CSS部分:
```css
.container {
position: relative;
width: 500px;
height: 500px;
border: 1px solid #ccc;
}
.moving-img {
position: absolute;
}
.moving-path {
position: absolute;
top: 0;
left: 0;
pointer-events: none;
}
```
JavaScript部分:
```javascript
new Vue({
el: "#app",
data() {
return {
imgSrc: "https://picsum.photos/200",
imgStyle: {},
pathWidth: 500,
pathHeight: 500,
path: "",
duration: 1000 // 移动时间,单位为毫秒
}
},
mounted() {
this.moveImage();
},
methods: {
moveImage() {
const container = document.querySelector(".container");
const img = document.querySelector(".moving-img");
const path = document.querySelector(".moving-path path");
const containerWidth = container.clientWidth;
const containerHeight = container.clientHeight;
const imgWidth = img.clientWidth;
const imgHeight = img.clientHeight;
const x1 = Math.floor(Math.random() * (containerWidth - imgWidth));
const y1 = Math.floor(Math.random() * (containerHeight - imgHeight));
const x2 = Math.floor(Math.random() * (containerWidth - imgWidth));
const y2 = Math.floor(Math.random() * (containerHeight - imgHeight));
const pathData = `M${x1},${y1} L${x2},${y2}`;
path.setAttribute("d", pathData);
this.imgStyle = {
left: `${x1}px`,
top: `${y1}px`,
transition: `transform ${this.duration}ms linear`
};
setTimeout(() => {
this.imgStyle.transform = `translate(${x2 - x1}px, ${y2 - y1}px)`;
}, 0);
setTimeout(() => {
this.moveImage();
}, this.duration);
}
}
})
```
解释一下代码:
1. 首先在HTML中定义了一个容器,包含了一个图片和一个SVG路径。图片使用了绝对定位,SVG路径使用了相对定位,将其放置在图片上方,并使用CSS中的`pointer-events: none`使其不会阻挡鼠标事件。
2. 在Vue实例中定义了一些数据,包括图片的路径和样式,SVG路径的宽度、高度和路径数据,以及图片移动的时间。
3. 在Vue的`mounted`生命周期钩子中调用了`moveImage`方法,开始执行图片的移动。
4. `moveImage`方法中首先获取了容器、图片和SVG路径的一些信息,包括宽度、高度和位置。
5. 然后使用`Math.random`方法生成了下一次图片的位置,并根据这个位置生成了SVG路径的数据。路径数据的格式为`Mx1,y1 Lx2,y2`,表示从`x1,y1`到`x2,y2`的一条直线。
6. 将路径数据设置给SVG路径的`d`属性,将图片的样式设置为绝对定位,并使用`transition`属性设置了移动的时间和动画效果。
7. 通过`setTimeout`方法将图片的`transform`属性设置为`translate(x2 - x1, y2 - y1)`,实现图片的移动。
8. 在移动完成后,通过`setTimeout`方法再次调用`moveImage`方法,开始下一次移动。
这样就实现了一个随机移动图片的效果,同时也能看到移动的轨迹。
阅读全文
相关推荐
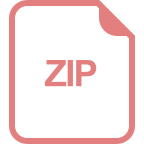
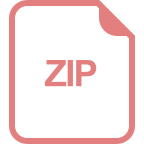
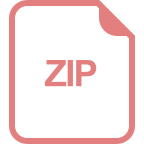
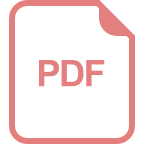
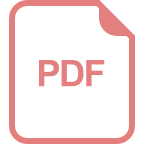
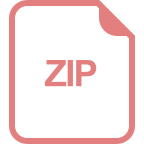
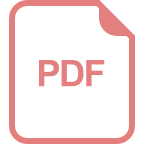
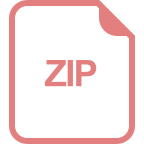
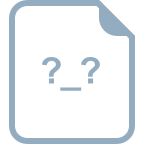
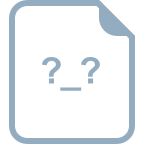
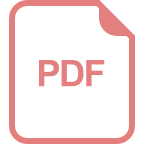
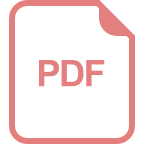
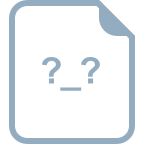