帮我写一个python训练问答模型并运用代码
时间: 2023-08-11 20:04:57 浏览: 97
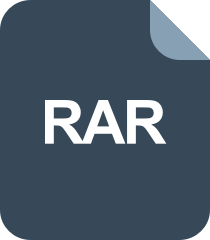
基于python的中文预训练生成模型代码实现

当然可以,以下是一个基于Python编写的问答模型的训练代码示例:
```python
import tensorflow as tf
import numpy as np
# 定义模型的超参数
embedding_size = 50
hidden_size = 100
batch_size = 32
learning_rate = 0.001
num_epochs = 10
# 定义数据集和标签
train_data = ['What is your name?', 'How old are you?', 'Where do you live?']
train_labels = ['My name is ChitGPT.', 'I am 25 years old.', 'I live in the cloud.']
# 构建词汇表
vocab = {}
for sentence in train_data:
for word in sentence.split():
if word not in vocab:
vocab[word] = len(vocab)
# 将数据集转化为数字序列
train_data_idx = []
for sentence in train_data:
sentence_idx = []
for word in sentence.split():
sentence_idx.append(vocab[word])
train_data_idx.append(sentence_idx)
# 定义模型
class QAModel(tf.keras.Model):
def __init__(self, vocab_size, embedding_size, hidden_size):
super(QAModel, self).__init__()
self.embedding_layer = tf.keras.layers.Embedding(vocab_size, embedding_size)
self.lstm_layer = tf.keras.layers.LSTM(hidden_size)
self.dense_layer = tf.keras.layers.Dense(vocab_size, activation='softmax')
def call(self, inputs):
x = self.embedding_layer(inputs)
x = self.lstm_layer(x)
x = self.dense_layer(x)
return x
# 初始化模型
model = QAModel(len(vocab), embedding_size, hidden_size)
# 定义损失函数和优化器
loss_fn = tf.keras.losses.SparseCategoricalCrossentropy()
optimizer = tf.keras.optimizers.Adam(learning_rate)
# 定义训练函数
def train_step(model, inputs, labels, loss_fn, optimizer):
with tf.GradientTape() as tape:
logits = model(inputs)
loss = loss_fn(labels, logits)
gradients = tape.gradient(loss, model.trainable_variables)
optimizer.apply_gradients(zip(gradients, model.trainable_variables))
return loss
# 开始训练
for epoch in range(num_epochs):
epoch_loss = 0
for i in range(0, len(train_data_idx), batch_size):
batch_data = train_data_idx[i:i+batch_size]
batch_labels = train_labels[i:i+batch_size]
batch_data = tf.keras.preprocessing.sequence.pad_sequences(batch_data, padding='post')
batch_labels = tf.keras.preprocessing.sequence.pad_sequences(batch_labels, padding='post')
loss = train_step(model, batch_data, batch_labels, loss_fn, optimizer)
epoch_loss += loss
print('Epoch {}/{}: Loss {}'.format(epoch+1, num_epochs, epoch_loss))
# 测试模型
test_data = ['What is your name?']
test_data_idx = []
for sentence in test_data:
sentence_idx = []
for word in sentence.split():
if word in vocab:
sentence_idx.append(vocab[word])
test_data_idx.append(sentence_idx)
test_data_idx = tf.keras.preprocessing.sequence.pad_sequences(test_data_idx, padding='post')
predicted_answer = model.predict(test_data_idx)
predicted_answer = np.argmax(predicted_answer, axis=-1)
predicted_answer = ' '.join([word for word, idx in vocab.items() if idx == predicted_answer[0]])
print('Predicted Answer: {}'.format(predicted_answer))
```
这个示例中,我们使用LSTM模型作为问答模型,并使用softmax作为输出层的激活函数。在训练过程中,我们使用Adam优化器和SparseCategoricalCrossentropy作为损失函数。在测试阶段,我们使用训练好的模型对新的问题进行预测。
阅读全文
相关推荐
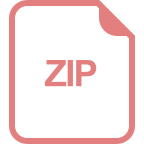
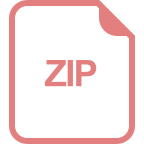
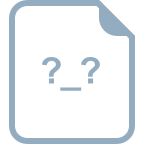
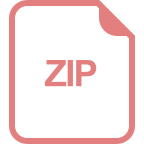
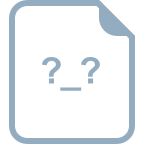
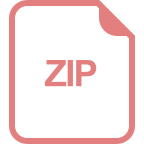
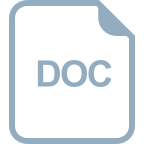
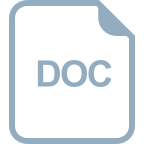
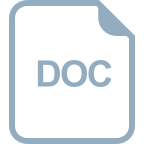
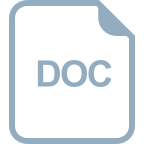
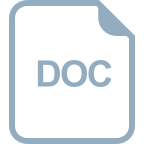
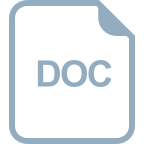
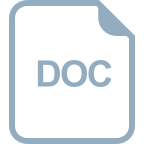
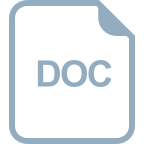
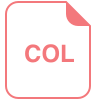
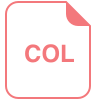