windows下画图工具怎么实现的多位位图转换单色位图代码
时间: 2024-05-05 08:17:41 浏览: 74
以下是一个简单的 C++ 代码示例,它将多位位图转换为单色位图:
```cpp
#include <Windows.h>
int main()
{
// 打开多位位图文件
HBITMAP hBitmap = (HBITMAP)LoadImage(NULL, L"input.bmp", IMAGE_BITMAP, 0, 0, LR_LOADFROMFILE);
// 获取位图信息
BITMAP bitmap;
GetObject(hBitmap, sizeof(bitmap), &bitmap);
// 创建单色位图
HDC hdc = GetDC(NULL);
HDC hdcMem = CreateCompatibleDC(hdc);
HBITMAP hMonoBitmap = CreateBitmap(bitmap.bmWidth, bitmap.bmHeight, 1, 1, NULL);
HBITMAP hOldBitmap = (HBITMAP)SelectObject(hdcMem, hMonoBitmap);
// 使用BitBlt函数将多位位图转换为单色位图
SetStretchBltMode(hdcMem, COLORONCOLOR);
SetBitmapBits(hMonoBitmap, bitmap.bmWidth * bitmap.bmHeight / 8, NULL);
BitBlt(hdcMem, 0, 0, bitmap.bmWidth, bitmap.bmHeight, hdc, 0, 0, SRCCOPY);
// 保存单色位图
SaveBitmap(hMonoBitmap, L"output.bmp");
// 清理资源
SelectObject(hdcMem, hOldBitmap);
DeleteObject(hBitmap);
DeleteDC(hdcMem);
ReleaseDC(NULL, hdc);
return 0;
}
// 保存位图到文件
void SaveBitmap(HBITMAP hBitmap, LPCTSTR lpFileName)
{
BITMAP bitmap;
GetObject(hBitmap, sizeof(bitmap), &bitmap);
BITMAPFILEHEADER fileHeader;
fileHeader.bfType = 0x4d42;
fileHeader.bfSize = sizeof(BITMAPFILEHEADER) + sizeof(BITMAPINFOHEADER) + bitmap.bmWidth * bitmap.bmHeight / 8;
fileHeader.bfReserved1 = 0;
fileHeader.bfReserved2 = 0;
fileHeader.bfOffBits = sizeof(BITMAPFILEHEADER) + sizeof(BITMAPINFOHEADER);
BITMAPINFOHEADER infoHeader;
infoHeader.biSize = sizeof(BITMAPINFOHEADER);
infoHeader.biWidth = bitmap.bmWidth;
infoHeader.biHeight = bitmap.bmHeight;
infoHeader.biPlanes = 1;
infoHeader.biBitCount = 1;
infoHeader.biCompression = BI_RGB;
infoHeader.biSizeImage = bitmap.bmWidth * bitmap.bmHeight / 8;
infoHeader.biXPelsPerMeter = 0;
infoHeader.biYPelsPerMeter = 0;
infoHeader.biClrUsed = 0;
infoHeader.biClrImportant = 0;
HANDLE hFile = CreateFile(lpFileName, GENERIC_WRITE, 0, NULL, CREATE_ALWAYS, FILE_ATTRIBUTE_NORMAL, NULL);
DWORD dwWritten = 0;
WriteFile(hFile, &fileHeader, sizeof(fileHeader), &dwWritten, NULL);
WriteFile(hFile, &infoHeader, sizeof(infoHeader), &dwWritten, NULL);
BYTE* pBits = new BYTE[bitmap.bmWidth * bitmap.bmHeight / 8];
GetBitmapBits(hBitmap, bitmap.bmWidth * bitmap.bmHeight / 8, pBits);
WriteFile(hFile, pBits, bitmap.bmWidth * bitmap.bmHeight / 8, &dwWritten, NULL);
CloseHandle(hFile);
delete[] pBits;
}
```
此代码使用 `LoadImage` 函数从文件中加载多位位图,然后使用 `CreateBitmap` 函数创建一个与原始位图大小相同的单色位图。接下来,使用 `BitBlt` 函数将多位位图转换为单色位图,并使用 `SaveBitmap` 函数将单色位图保存到文件中。
请注意,此代码仅适用于单色位图中每个像素只有一位的情况。如果单色位图中每个像素具有多个位,则需要更改代码以相应地处理。
阅读全文
相关推荐
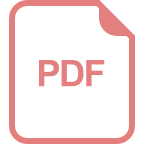
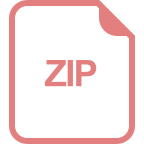
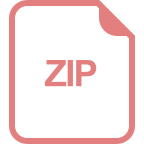

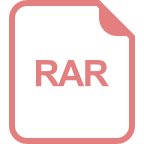
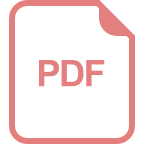
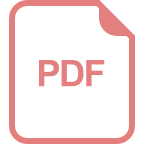
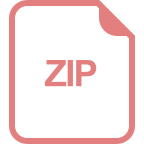
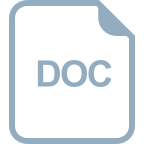
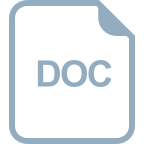
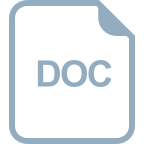
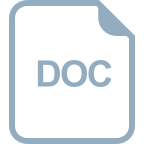
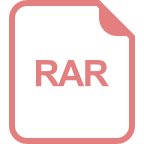
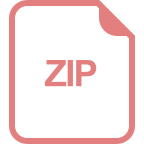
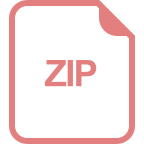
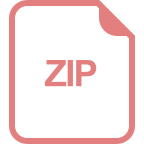
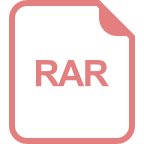
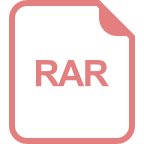