如何用cv2将非封闭的且有枝杈的正方形的图像处理成标准的正方形
时间: 2024-03-23 08:43:25 浏览: 13
如果要将非封闭的、有枝杈的正方形的图像处理成标准的正方形,可以使用以下步骤:
1. 使用cv2读取图像并获取其宽度和高度。
2. 找到图像中最大的轮廓。可以使用cv2.findContours函数找到所有的轮廓,然后选择面积最大的轮廓。
3. 使用cv2.minAreaRect函数获取最大轮廓的最小外接矩形。
4. 将最小外接矩形旋转到水平位置,使其长边与图像的边对齐。可以使用cv2.getRotationMatrix2D和cv2.warpAffine函数实现旋转。
5. 使用cv2.getRectSubPix函数从旋转后的图像中提取一个正方形区域。
6. 对提取的正方形区域进行缩放,使其变成标准的正方形。
下面是一个示例代码:
``` python
import cv2
import numpy as np
# 读取图像
img = cv2.imread('image.jpg')
# 转换为灰度图像
gray = cv2.cvtColor(img, cv2.COLOR_BGR2GRAY)
# 二值化
_, thresh = cv2.threshold(gray, 127, 255, cv2.THRESH_BINARY)
# 找到所有轮廓
contours, _ = cv2.findContours(thresh, cv2.RETR_TREE, cv2.CHAIN_APPROX_NONE)
# 找到面积最大的轮廓
max_area = 0
max_contour = None
for contour in contours:
area = cv2.contourArea(contour)
if area > max_area:
max_area = area
max_contour = contour
# 获取最小外接矩形
rect = cv2.minAreaRect(max_contour)
# 计算旋转角度
angle = rect[-1]
# 计算旋转后的图像尺寸
width, height = rect[1]
if angle < -45:
angle += 90
width, height = height, width
# 计算旋转矩阵
rotation_matrix = cv2.getRotationMatrix2D(rect[0], angle, 1)
# 旋转图像
rotated_img = cv2.warpAffine(img, rotation_matrix, (img.shape[1], img.shape[0]))
# 提取正方形区域
square_size = min(width, height)
square_center = rect[0]
square_roi = cv2.getRectSubPix(rotated_img, (square_size, square_size), square_center)
# 缩放为标准尺寸
standard_size = 256
resized_roi = cv2.resize(square_roi, (standard_size, standard_size))
# 显示图像
cv2.imshow('Resized ROI', resized_roi)
cv2.waitKey(0)
cv2.destroyAllWindows()
```
这样,就可以将非封闭的、有枝杈的正方形的图像处理成标准的正方形。
相关推荐
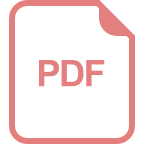
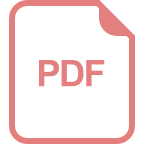




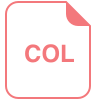









