java给PFD添加文字水印
时间: 2023-12-08 21:06:38 浏览: 93
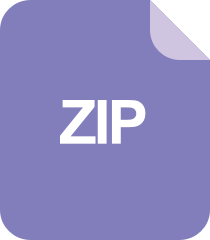
java.util实现图片水印添加功能,有添加图片水印和文字水印,可以设置水印位置,透明度等,可以参考代码加以改进成小工具
可以通过使用Java的Graphics2D类来添加文字水印到PDF文件中。以下是一个示例代码:
```java
import java.awt.Color;
import java.awt.Font;
import java.awt.Graphics2D;
import java.awt.geom.AffineTransform;
import java.io.File;
import java.io.FileOutputStream;
import java.io.IOException;
import org.apache.pdfbox.pdmodel.PDDocument;
import org.apache.pdfbox.pdmodel.PDPage;
import org.apache.pdfbox.pdmodel.PDPageContentStream;
import org.apache.pdfbox.pdmodel.font.PDType1Font;
public class AddTextWatermarkToPDF {
public static void main(String[] args) {
String inputFile = "input.pdf";
String outputFile = "output.pdf";
String watermarkText = "Confidential";
try {
PDDocument document = PDDocument.load(new File(inputFile));
for (PDPage page : document.getPages()) {
PDPageContentStream contentStream = new PDPageContentStream(document, page, PDPageContentStream.AppendMode.APPEND, true, true);
// set font and font size
contentStream.setFont(PDType1Font.HELVETICA_BOLD, 72);
// set text color to red
contentStream.setNonStrokingColor(Color.RED);
// rotate the text 45 degrees
AffineTransform transform = new AffineTransform();
transform.rotate(Math.toRadians(45), 0, 0);
contentStream.setTextMatrix(transform);
// get the width and height of the text
float textWidth = PDType1Font.HELVETICA_BOLD.getStringWidth(watermarkText) / 1000 * 72;
float textHeight = PDType1Font.HELVETICA_BOLD.getFontDescriptor().getFontBoundingBox().getHeight() / 1000 * 72;
// set the position of the text
float x = (page.getMediaBox().getWidth() - textWidth) / 2;
float y = (page.getMediaBox().getHeight() - textHeight) / 2;
// write the text to the content stream
contentStream.beginText();
contentStream.newLineAtOffset(x, y);
contentStream.showText(watermarkText);
contentStream.endText();
contentStream.close();
}
// save the PDF document with the watermark
document.save(new FileOutputStream(outputFile));
document.close();
} catch (IOException e) {
e.printStackTrace();
}
}
}
```
这个示例代码使用Apache PDFBox库来操作PDF文件。它打开一个PDF文件,遍历每个页面,然后在每个页面上添加一个旋转的、红色的、加粗的文本水印。如果需要更改水印文本、字体、颜色或位置,可以修改代码中的相关行。
阅读全文
相关推荐
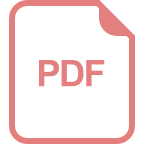
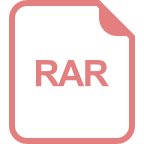
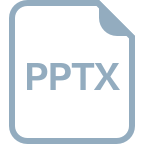
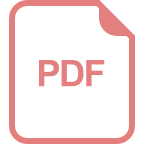
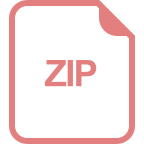





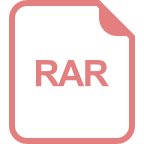
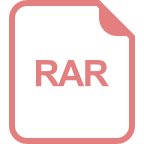
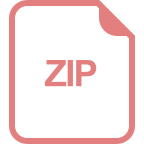
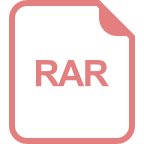
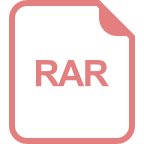
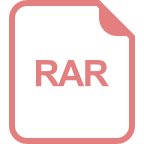