java单链表基本操作的实现 [问题描述] 1、用顺序表方式实现在线通讯录的设计,可以对通讯录作插入、删除、显示、查找、修改等操作。(必做题) 2、用单链表方式实现在线通讯录的设计,可以对通讯录作插入、删除、显示、查找、修改等操作。(必做题)
时间: 2023-10-19 21:20:42 浏览: 82
单链表基本操作的实现:
1. 定义节点类Node:
```java
public class Node {
public String name;
public String phoneNumber;
public Node next;
public Node(String name, String phoneNumber) {
this.name = name;
this.phoneNumber = phoneNumber;
this.next = null;
}
}
```
2. 定义单链表类LinkedList:
```java
public class LinkedList {
private Node head;
public LinkedList() {
head = null;
}
// 插入节点
public void insert(String name, String phoneNumber) {
Node newNode = new Node(name, phoneNumber);
if (head == null) {
head = newNode;
} else {
Node current = head;
while (current.next != null) {
current = current.next;
}
current.next = newNode;
}
}
// 删除节点
public void delete(String name) {
if (head == null) {
return;
}
if (head.name.equals(name)) {
head = head.next;
return;
}
Node current = head;
while (current.next != null) {
if (current.next.name.equals(name)) {
current.next = current.next.next;
return;
}
current = current.next;
}
}
// 显示所有节点
public void display() {
Node current = head;
while (current != null) {
System.out.println(current.name + " " + current.phoneNumber);
current = current.next;
}
}
// 查找节点
public Node find(String name) {
Node current = head;
while (current != null) {
if (current.name.equals(name)) {
return current;
}
current = current.next;
}
return null;
}
// 修改节点
public void modify(String name, String newPhoneNumber) {
Node node = find(name);
if (node != null) {
node.phoneNumber = newPhoneNumber;
}
}
}
```
3. 测试代码:
```java
public class Test {
public static void main(String[] args) {
LinkedList list = new LinkedList();
// 插入节点
list.insert("张三", "123456");
list.insert("李四", "234567");
list.insert("王五", "345678");
// 显示所有节点
list.display();
// 查找节点
Node node = list.find("李四");
if (node != null) {
System.out.println(node.phoneNumber);
}
// 修改节点
list.modify("张三", "111111");
// 删除节点
list.delete("王五");
// 显示所有节点
list.display();
}
}
```
阅读全文
相关推荐



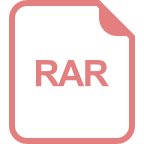




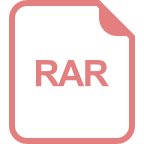


