np.asarray举例
时间: 2023-10-18 22:04:38 浏览: 76
好的,关于 np.asarray 的使用,举例如下:
```python
import numpy as np
# 通过列表创建ndarray
a = [1, 2, 3]
arr1 = np.asarray(a)
print(arr1) # 输出 [1 2 3]
# 通过元组创建ndarray,并指定数据类型为float
b = (4, 5, 6)
arr2 = np.asarray(b, dtype=np.float)
print(arr2) # 输出 [4. 5. 6.]
# 通过数组创建ndarray,并修改其数据类型为int8
c = np.array([7, 8, 9])
arr3 = np.asarray(c, dtype=np.int8)
print(arr3) # 输出 [7 8 9]
```
阅读全文
相关推荐









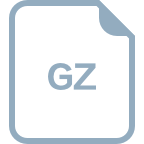




