python 读取elf文件至txt中
时间: 2023-06-22 15:44:20 浏览: 103
您可以使用Python中的`readelf`命令来读取ELF文件,然后将其输出到一个文本文件中。以下是一个示例代码:
```python
import subprocess
# 调用 readelf 命令读取 ELF 文件信息
result = subprocess.run(['readelf', '-a', 'your_elf_file'], stdout=subprocess.PIPE)
# 将结果输出到一个文本文件中
with open('output.txt', 'w') as f:
f.write(result.stdout.decode())
```
请将 `your_elf_file` 替换为您要读取的ELF文件的文件名。运行此代码将会生成一个名为 `output.txt` 的文本文件,其中包含了该 ELF 文件的所有信息。
相关问题
python 读取elf文件中所有信息至txt中
可以使用Python的`elftools`库来读取ELF文件的信息,然后将所需信息写入到txt文件中。
以下是一个示例代码,可以读取ELF文件的节表信息,并将其写入到txt文件中:
```python
from elftools.elf.elffile import ELFFile
# 打开ELF文件
with open('sample.elf', 'rb') as f:
elf = ELFFile(f)
# 获取节表信息
sections = elf.iter_sections()
# 写入txt文件
with open('output.txt', 'w') as output:
for section in sections:
# 获取节表名称和偏移
name = section.name
offset = section.header.sh_offset
# 读取节表内容
f.seek(offset)
content = f.read(section.header.sh_size)
# 将节表信息写入txt文件
output.write(f'Section Name: {name}\n')
output.write(f'Content:\n{content}\n\n')
```
注意:此示例代码仅演示如何读取ELF文件的节表信息,并将其写入到txt文件中。如果需要读取其他信息,可以参考`elftools`库的文档。同时,要注意保护好自己的ELF文件,不要泄露给他人。
python 读取完整的elf文件至txt中
可以使用Python中的`objdump`工具来读取elf文件并将其输出到txt文件中。可以使用`subprocess`模块来调用`objdump`命令,示例代码如下:
```python
import subprocess
elf_file = "example.elf"
txt_file = "example.txt"
# 调用objdump命令将elf文件输出到txt文件中
subprocess.run(["objdump", "-D", elf_file, "-M", "intel", "-b", "elf32", "-m", "i386", "-EB", "-z", "-w", "-j", ".text", ">", txt_file], shell=True)
```
这段代码会将`example.elf`文件中的`.text`段输出到`example.txt`文件中,并使用Intel格式的汇编代码。你可以根据需要修改命令中的参数来获取其它段或格式的数据。
相关推荐
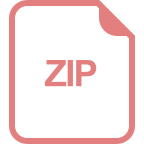
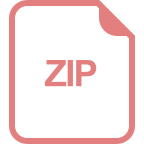
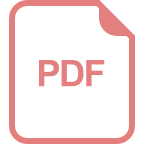










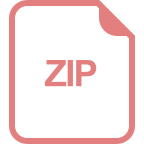