有python画一个心形
时间: 2023-02-27 14:17:14 浏览: 6
可以使用Matplotlib库来画一个心形。
下面是一个示例代码:
```
import numpy as np
import matplotlib.pyplot as plt
t = np.linspace(0, 2*np.pi, 1000)
x = 16 * np.sin(t) ** 3
y = 13 * np.cos(t) - 5 * np.cos(2*t) - 2 * np.cos(3*t) - np.cos(4*t)
plt.plot(x, y)
plt.show()
```
运行这段代码,就可以得到一个心形的图形了。
相关问题
用python画一个心形
### 回答1:
可以使用 Python 的 `matplotlib` 库来画出心形。具体的实现方法如下:
1. 首先需要导入 `matplotlib.pyplot` 库,并使用 `figure` 函数创建一个新图形。
2. 然后使用 `plot` 函数绘制出心形的曲线。可以使用参数 `t` 控制心形的形状。可以算出心形的两个数学函数,x=16sin^3(t),y=13cos(t)-5cos(2t)-2cos(3t)-cos(4t)
3. 使用 `show` 函数显示图形。
下面是一个示例代码:
```
import numpy as np
import matplotlib.pyplot as plt
t = np.linspace(0, 2 * np.pi, 1000)
x = 16 * np.power(np.sin(t), 3)
y = 13 * np.cos(t) - 5 * np.cos(2*t) - 2 * np.cos(3*t) - np.cos(4*t)
plt.plot(x, y)
plt.axis("equal") # 设置坐标轴比例
plt.show()
```
运行上述代码后,会弹出一个新窗口,显示出一个心形。
### 回答2:
要用Python画一个心形,可以使用turtle库来实现。下面是一个简单的代码示例:
```python
import turtle
# 设置画布的尺寸
turtle.setup(800, 600)
# 设置画笔的颜色和粗细
turtle.pensize(5)
turtle.pencolor("red")
# 绘制左侧的半个心形
turtle.left(45)
turtle.circle(100, 180)
# 绘制右侧的半个心形
turtle.seth(45)
turtle.circle(100, 180)
# 隐藏画笔
turtle.hideturtle()
# 结束绘制
turtle.done()
```
运行以上代码,就可以在一个画布上看到用Python绘制的一个心形图案。这段代码使用了turtle库的一些基本函数,比如`setup()`用于设置画布的尺寸,`pensize()`设置画笔的粗细,`pencolor()`设置画笔的颜色,`left()`设置旋转角度,`circle()`用于绘制圆弧,`hideturtle()`隐藏画笔,`done()`结束绘制。通过适当的调整参数和初始角度,可以画出不同尺寸和形态的心形图案。
### 回答3:
可以使用turtle模块来使用Python画一个心形,代码如下:
```python
import turtle
def draw_heart():
turtle.speed(5)
turtle.penup()
turtle.goto(0, -100) # 移动到画图的起始位置
turtle.pendown()
turtle.begin_fill()
turtle.left(140) # 旋转角度
turtle.forward(224) # 前进
curve()
turtle.left(120)
curve()
turtle.forward(224)
turtle.end_fill()
turtle.hideturtle() # 隐藏海龟图标
def curve():
for i in range(200):
turtle.right(1) # 右转角度
turtle.forward(1) # 前进
turtle.setup(600, 400) # 设置窗口大小
turtle.color('red') # 设置画笔颜色
turtle.fillcolor('pink') # 设置填充颜色
draw_heart()
turtle.done()
```
这段代码使用turtle模块来绘制一个心形图案。首先,我们导入turtle模块。然后定义了一个函数`draw_heart()`来画心形。在函数内部,我们设置了画笔的速度,然后将画笔移动到画图的起始位置,后续使用`pendown()`方法开始绘制。我们使用`begin_fill()`和`end_fill()`方法来进行填充,填充颜色为粉色。然后,我们通过一系列的移动和旋转来绘制两个弧线,形成了一个心形图案。最后,使用`hideturtle()`方法隐藏海龟图标。接下来,我们设置了窗口的大小,画笔的颜色以及填充颜色,并调用`draw_heart()`函数来绘制心形图案。最后,使用`turtle.done()`来保持窗口的显示。
运行代码后,就可以在窗口中看到绘制出的心形图案。
用Python画一个心形
### 回答1:
可以使用Python的Matplotlib库来画一个心形。例如,你可以使用以下代码:import matplotlib.pyplot as plt x = [1,2,3,4,5,6,7,8,9] y = [9,8,7,6,5,4,3,2,1] plt.plot(x,y) plt.show()
### 回答2:
在Python中,我们可以使用turtle模块来绘制一个心形。下面是一个画心形的Python代码:
```python
import turtle
# 设置画笔的形状和颜色
turtle.shape('turtle')
turtle.color('red')
# 设置起始点的位置
turtle.penup()
turtle.goto(0, -100)
turtle.pendown()
# 绘制一个半个心形
turtle.begin_fill()
turtle.left(140)
turtle.forward(224)
turtle.circle(-90, 200)
turtle.left(120)
turtle.circle(-90, 200)
turtle.forward(224)
turtle.end_fill()
# 隐藏画笔
turtle.hideturtle()
# 让窗口停留
turtle.mainloop()
```
将上述代码复制到Python环境中执行,即可得到一个绘制出的心形图案。
### 回答3:
Python是一种编程语言,可以用来实现各种各样的功能,包括绘图。下面我将给出一种使用Python绘制心形的方式。
首先,我们需要导入一个用于绘图的库,比如matplotlib。然后,我们可以使用其中的plot函数来绘制心形曲线。
下面是代码示例:
```python
import matplotlib.pyplot as plt
import numpy as np
# 生成心形曲线上的所有点的坐标
t = np.linspace(0, 2*np.pi, 1000)
x = 16*np.power(np.sin(t), 3)
y = 13*np.cos(t) - 5*np.cos(2*t) - 2*np.cos(3*t) - np.cos(4*t)
# 绘制心形曲线
plt.plot(x, y, color='red')
# 设置坐标轴范围
plt.xlim(-20, 20)
plt.ylim(-20, 20)
# 去掉坐标轴
plt.axis('off')
# 显示绘制结果
plt.show()
```
运行这段代码,就可以在图形窗口中看到一个绘制好的心形。
这段代码中,我们使用了参数方程来生成心形曲线上的所有点的坐标。然后,通过plot函数将这些点连成一条曲线,并设置了颜色为红色。接着,通过xlim和ylim函数设置了坐标轴范围,可以根据需要进行调整。最后,通过axis函数将坐标轴隐藏起来,使得只显示心形曲线。
希望以上内容能对你有所帮助。如果有任何疑问,请随时追问。
阅读全文
相关推荐
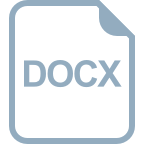
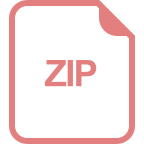
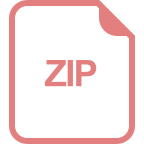




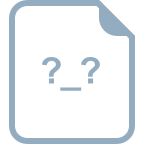
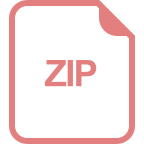
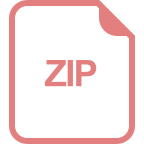
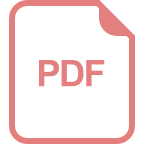
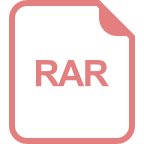
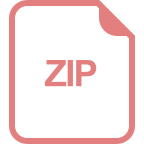
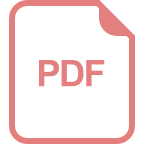
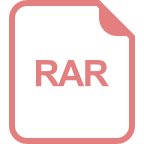