python resultset转字符串
时间: 2024-10-25 22:01:28 浏览: 32
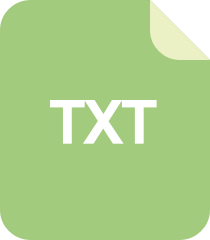
java字符串编码转换

在Python中,如果你有一个数据库查询的结果集(通常是一个游标或者从数据库库如SQLite、MySQL等返回的数据集),将其转换成字符串通常涉及到迭代遍历结果并连接它们。例如,如果你使用的是sqlite3库:
```python
import sqlite3
# 假设 cursor 是一个查询后的游标对象
cursor.execute("SELECT * FROM your_table")
# 使用 ''.join() 和 format() 或者 map() + join() 来拼接字符串
# 这里我们假设每一行有两列,column1和column2
rows = cursor.fetchall()
str_output = '\n'.join(['{}: {}'.format(row[0], row[1]) for row in rows])
print(str_output)
```
这里我们使用了`'\n'.join()`将每行数据用逗号分隔,并添加换行符,形成一个字符串列表后再合并。
如果你使用的是ORM框架如SQLAlchemy,处理方式可能会略有不同,但基本思路是一致的,都是遍历结果并构建字符串。
阅读全文
相关推荐
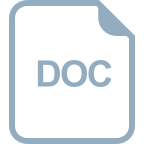
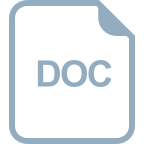



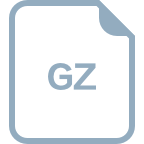
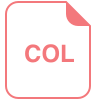
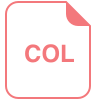
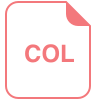
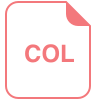
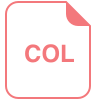
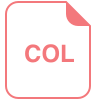
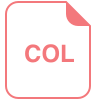
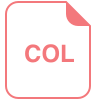
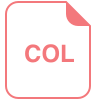
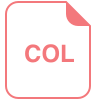
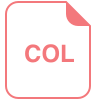
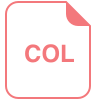