用Java语言读取doc或者docx类型的word文档,读取文档中的标题,标题级别,文本内容
时间: 2024-04-30 15:22:41 浏览: 7
可以使用Apache POI库来读取doc或者docx类型的word文档。
以下是一个示例代码:
```java
import java.io.FileInputStream;
import java.io.IOException;
import java.io.InputStream;
import org.apache.poi.hwpf.HWPFDocument;
import org.apache.poi.hwpf.usermodel.Paragraph;
import org.apache.poi.hwpf.usermodel.Range;
import org.apache.poi.xwpf.usermodel.XWPFDocument;
import org.apache.poi.xwpf.usermodel.XWPFParagraph;
public class WordReader {
public static void main(String[] args) {
String filePath = "path/to/word/document";
try (InputStream inputStream = new FileInputStream(filePath)) {
if (filePath.endsWith(".doc")) {
// Read .doc file
HWPFDocument document = new HWPFDocument(inputStream);
Range range = document.getRange();
for (int i = 0; i < range.numParagraphs(); i++) {
Paragraph paragraph = range.getParagraph(i);
// get the text content of the paragraph
String text = paragraph.text();
// get the heading level of the paragraph
int level = paragraph.getLvl();
// do something with the text and level
}
} else if (filePath.endsWith(".docx")) {
// Read .docx file
XWPFDocument document = new XWPFDocument(inputStream);
for (XWPFParagraph paragraph : document.getParagraphs()) {
// get the text content of the paragraph
String text = paragraph.getText();
// get the heading level of the paragraph
String style = paragraph.getStyle();
int level = 0;
if (style != null && style.startsWith("Heading")) {
level = Integer.parseInt(style.substring("Heading".length()));
}
// do something with the text and level
}
} else {
throw new IllegalArgumentException("Unsupported file format: " + filePath);
}
} catch (IOException e) {
e.printStackTrace();
}
}
}
```
在上面的代码中,我们首先判断文件类型是.doc还是.docx,然后使用HWPFDocument或XWPFDocument类来读取文档内容。对于每个段落,我们可以使用Paragraph或XWPFParagraph类来获取文本内容和标题级别。如果是.docx文件,我们需要使用getStyle()方法来获取段落的样式,然后从样式中提取标题级别。
相关推荐
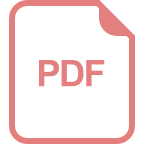
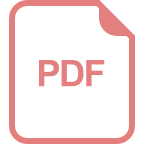
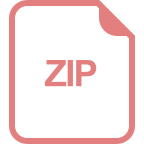














