android调整textview的位置
时间: 2023-04-22 14:01:35 浏览: 785
要调整Android中TextView的位置,可以使用以下方法:
1.使用布局文件:在布局文件中,可以使用layout_margin属性来调整TextView的位置。例如,将TextView向右移动10dp:
```
<TextView
android:id="@+id/myTextView"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="Hello World!"
android:layout_marginLeft="10dp"/>
```
2.使用代码:在代码中,可以使用LayoutParams来设置TextView的位置。例如,将TextView向下移动20dp:
```
TextView myTextView = findViewById(R.id.myTextView);
LayoutParams params = (LayoutParams) myTextView.getLayoutParams();
params.setMargins(, 20, , );
myTextView.setLayoutParams(params);
```
以上是两种常用的方法,可以根据具体情况选择使用。
相关问题
android studio textview位置怎么调整
在Android Studio中,TextView的位置可以通过XML布局文件或者程序代码动态调整。以下是两种常见的方法:
1. XML布局调整:
- 使用`android:layout_x`和`android:layout_y`属性:在<LinearLayout>、<RelativeLayout>等容器中,直接设置TextView的左上角坐标,例如:
```xml
<TextView
android:id="@+id/my_text_view"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_x="50dp" <!-- x轴偏移 -->
android:layout_y="100dp" <!-- y轴偏移 -->
/>
```
2. Java / Kotlin代码调整:
- 获取TextView实例后,可以使用`View`类的方法如`setTranslationX()` 和 `setTranslationY()` 来改变位置:
```java
TextView textView = findViewById(R.id.my_text_view);
textView.setTranslationX(50); // 水平偏移
textView.setTranslationY(100); // 垂直偏移
```
或者使用`LayoutParams`对象调整:
```java
TextView.LayoutParams layoutParams = (TextView.LayoutParams) textView.getLayoutParams();
layoutParams.leftMargin = 50; // 左侧边距
layoutParams.topMargin = 100; // 上方边距
textView.setLayoutParams(layoutParams);
```
Android Studio中如何调整TextView的位置?
在Android Studio中,调整TextView的位置通常涉及XML布局文件或通过代码动态设置。以下是两种常见的方式:
**1. XML布局文件调整:**
在res/layout下的XML布局文件中,你可以直接设置`android:layout_x` 和 `android:layout_y` 属性来指定TextView的水平和垂直偏移量。例如:
```xml
<TextView
android:id="@+id/my_text_view"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_centerHorizontal="true" <!-- 水平居中 -->
android:layout_marginTop="50dp" <!-- 上方距离顶部50dp -->
/>
```
**2. Java/Kotlin代码动态调整:**
在Activity或Fragment的onCreateView或onLayout回调中,你可以获取TextView的View对象并使用`setTranslationX()`和`setTranslationY()`方法来改变位置:
```java
TextView textView = findViewById(R.id.my_text_view);
int xTranslation = 100; // 横向偏移量
int yTranslation = 200; // 纵向偏移量
textView.setTranslationX(xTranslation);
textView.setTranslationY(yTranslation);
```
记得在更新UI时调用`requestLayout()`或`invalidate()`来刷新视图。
阅读全文
相关推荐
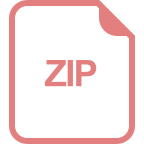
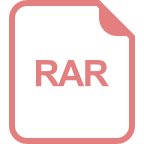
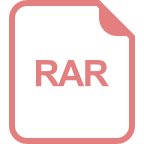
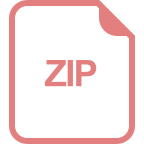
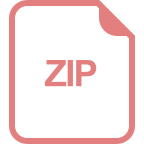
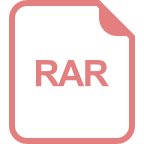
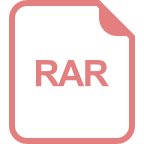
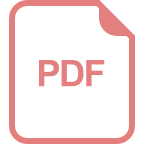
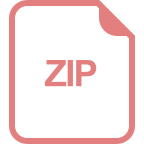
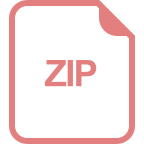




