java调用百度接口识别图片文字
时间: 2023-08-21 22:03:48 浏览: 60
你可以使用百度的OCR接口来实现Java调用百度接口别图片文字的功能。首先,你需要注册百度开发者账号并创建应用,获取到API Key和Secret Key。
接下来,你可以使用Java的网络请求库,例如Apache HttpClient或OkHttp,来发送HTTP POST请求给百度OCR接口。在请求中,你需要包含以下参数:
- 接口地址:https://aip.baidubce.com/rest/2.0/ocr/v1/general_basic
- 请求参数:image(图片的Base64编码)、access_token(通过API Key和Secret Key获取的访问令牌)
- 请求头:Content-Type设置为application/x-www-form-urlencoded
以下是一个示例代码:
```java
import org.apache.http.HttpEntity;
import org.apache.http.HttpResponse;
import org.apache.http.NameValuePair;
import org.apache.http.client.entity.UrlEncodedFormEntity;
import org.apache.http.client.methods.HttpPost;
import org.apache.http.client.utils.URLEncodedUtils;
import org.apache.http.impl.client.CloseableHttpClient;
import org.apache.http.impl.client.HttpClients;
import org.apache.http.message.BasicNameValuePair;
import org.apache.http.util.EntityUtils;
import java.io.IOException;
import java.util.ArrayList;
import java.util.List;
public class BaiduOCR {
public static void main(String[] args) throws IOException {
// 设置API Key和Secret Key
String apiKey = "your_api_key";
String secretKey = "your_secret_key";
// 获取访问令牌
String accessToken = getAccessToken(apiKey, secretKey);
// 图片路径
String imagePath = "path_to_your_image";
// 将图片转为Base64编码
String imageBase64 = getImageBase64(imagePath);
// 调用百度OCR接口
String result = callBaiduOCR(imageBase64, accessToken);
System.out.println(result);
}
// 获取访问令牌
private static String getAccessToken(String apiKey, String secretKey) throws IOException {
CloseableHttpClient httpClient = HttpClients.createDefault();
HttpPost httpPost = new HttpPost("https://aip.baidubce.com/oauth/2.0/token");
List<NameValuePair> params = new ArrayList<>();
params.add(new BasicNameValuePair("grant_type", "client_credentials"));
params.add(new BasicNameValuePair("client_id", apiKey));
params.add(new BasicNameValuePair("client_secret", secretKey));
httpPost.setEntity(new UrlEncodedFormEntity(params));
HttpResponse response = httpClient.execute(httpPost);
HttpEntity entity = response.getEntity();
String result = EntityUtils.toString(entity);
httpClient.close();
return result.split("\"")[3];
}
// 将图片转为Base64编码
private static String getImageBase64(String imagePath) {
// TODO: 实现将图片转为Base64编码的逻辑
return "";
}
// 调用百度OCR接口
private static String callBaiduOCR(String imageBase64, String accessToken) throws IOException {
CloseableHttpClient httpClient = HttpClients.createDefault();
HttpPost httpPost = new HttpPost("https://aip.baidubce.com/rest/2.0/ocr/v1/general_basic");
List<NameValuePair> params = new ArrayList<>();
params.add(new BasicNameValuePair("image", imageBase64));
params.add(new BasicNameValuePair("access_token", accessToken));
httpPost.setEntity(new UrlEncodedFormEntity(params));
HttpResponse response = httpClient.execute(httpPost);
HttpEntity entity = response.getEntity();
String result = EntityUtils.toString(entity);
httpClient.close();
return result;
}
}
```
请注意,在上述代码中,你需要实现将图片转为Base64编码的逻辑,你可以使用第三方库,如Apache Commons Codec,来实现该功能。
希望这能帮到你!如果有任何其他问题,请随时提问。
相关推荐
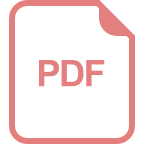
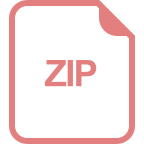














