kotlin Serializable
时间: 2023-10-08 17:12:46 浏览: 53
在Kotlin中,可以使用Serializable接口来实现对象的序列化。Serializable接口是Java平台提供的,允许将一个对象转换成字节流,以便在网络上传输或者保存到本地文件中。要在Kotlin中使用Serializable接口,只需要在类的定义前添加"implements Serializable"即可。
例如:
data class User(val id: Int, val name: String) : Serializable
这样,User类就可以被序列化了。当你需要将User对象转换成字节流时,可以直接使用Java的ObjectOutputStream类来实现。同样,将字节流转换成User对象时,可以使用ObjectInputStream类。
另外,在Kotlin中,除了Serializable接口,还可以使用Parcelable接口进行对象的序列化。Parcelable接口是Android平台提供的,相比Serializable接口,Parcelable接口更加高效,适用于在Android应用中传递大量数据对象。
如果你选择使用Parcelable接口,在Kotlin中需要进行一些额外的配置工作。你需要在类定义前添加@Parcelize注解,并添加一个主构造函数。然后,编译器会自动为你生成writeToParcel和createFromParcel方法。
综上所述,可以根据你的需求选择使用Serializable接口或Parcelable接口来实现对象的序列化。
相关问题
serialization kotlin
在Kotlin中,序列化是指将对象转换为字节流的过程,以便可以在网络上传输或者保存到文件中。Kotlin提供了一个内置的序列化框架,即Kotlin Serialization。下面是关于Kotlin Serialization的介绍:
1. Kotlin Serialization是什么?
Kotlin Serialization是Kotlin官方提供的一种轻量级的序列化框架,用于将Kotlin对象转换为字节流,以便进行传输或持久化存储。
2. 如何使用Kotlin Serialization?
使用Kotlin Serialization,你需要在你的Kotlin类上添加`@Serializable`注解,并且在属性上添加`@SerialName`注解来指定序列化后的字段名。然后,你可以使用`Json.encodeToString()`方法将对象序列化为JSON字符串,或使用`Json.decodeFromString()`方法将JSON字符串反序列化为对象。
3. Kotlin Serialization的特性有哪些?
Kotlin Serialization支持以下特性:
- 支持枚举类的序列化和反序列化
- 支持泛型类型的序列化和反序列化
kotlin getSerializable
To make a class serializable in Kotlin, you need to implement the `Serializable` interface. Here's an example:
```kotlin
import java.io.Serializable
data class Person(val name: String, val age: Int): Serializable
fun main() {
val person = Person("John", 30)
// Serialization
val outputStream = FileOutputStream("person.ser")
val objectOutputStream = ObjectOutputStream(outputStream)
objectOutputStream.writeObject(person)
objectOutputStream.close()
outputStream.close()
// Deserialization
val inputStream = FileInputStream("person.ser")
val objectInputStream = ObjectInputStream(inputStream)
val deserializedPerson = objectInputStream.readObject() as Person
objectInputStream.close()
inputStream.close()
println(deserializedPerson)
}
```
In this example, the `Person` class implements the `Serializable` interface. We then serialize an instance of the `Person` class into a file called "person.ser". Afterward, we deserialize the object from the file and print it out. Note that the serialization and deserialization process requires handling exceptions, but they are omitted here for brevity.
相关推荐
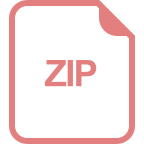
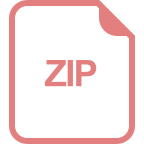
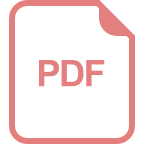
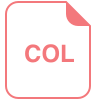











