springsecurity整合jwt 代码实现
时间: 2023-08-23 09:08:09 浏览: 129
Spring Security是Spring框架中的一个用于安全认证和授权的模块,而JWT(JSON Web Token)是一种基于JSON的安全令牌,常用于身份验证和授权。
下面是整合Spring Security和JWT的代码实现:
1. 引入依赖
在pom.xml文件中添加以下依赖:
``` xml
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-security</artifactId>
</dependency>
<dependency>
<groupId>io.jsonwebtoken</groupId>
<artifactId>jjwt</artifactId>
<version>0.9.1</version>
</dependency>
```
2. 创建JwtTokenUtil类
创建一个JwtTokenUtil类,用于生成和解析JWT令牌。
``` java
@Component
public class JwtTokenUtil {
private static final String SECRET_KEY = "secret";
private static final String ISSUER = "jwt";
public String generateToken(String username) {
Date now = new Date();
Date expiryDate = new Date(now.getTime() + 3600000);
return Jwts.builder()
.setSubject(username)
.setIssuer(ISSUER)
.setIssuedAt(now)
.setExpiration(expiryDate)
.signWith(SignatureAlgorithm.HS512, SECRET_KEY)
.compact();
}
public String getUsernameFromToken(String token) {
Claims claims = Jwts.parser()
.setSigningKey(SECRET_KEY)
.parseClaimsJws(token)
.getBody();
return claims.getSubject();
}
public boolean validateToken(String token) {
try {
Jwts.parser().setSigningKey(SECRET_KEY).parseClaimsJws(token);
return true;
} catch (SignatureException ex) {
System.out.println("Invalid JWT signature");
} catch (MalformedJwtException ex) {
System.out.println("Invalid JWT token");
} catch (ExpiredJwtException ex) {
System.out.println("Expired JWT token");
} catch (UnsupportedJwtException ex) {
System.out.println("Unsupported JWT token");
} catch (IllegalArgumentException ex) {
System.out.println("JWT claims string is empty");
}
return false;
}
}
```
3. 创建JwtAuthenticationFilter类
创建一个JwtAuthenticationFilter类,用于过滤并验证JWT令牌。
``` java
public class JwtAuthenticationFilter extends OncePerRequestFilter {
private JwtTokenUtil jwtTokenUtil;
public JwtAuthenticationFilter(JwtTokenUtil jwtTokenUtil) {
this.jwtTokenUtil = jwtTokenUtil;
}
@Override
protected void doFilterInternal(HttpServletRequest request, HttpServletResponse response, FilterChain chain) throws ServletException, IOException {
String header = request.getHeader("Authorization");
if (header != null && header.startsWith("Bearer ")) {
String token = header.substring(7);
if (jwtTokenUtil.validateToken(token)) {
String username = jwtTokenUtil.getUsernameFromToken(token);
UserDetails userDetails = new User(username, "", Collections.emptyList());
UsernamePasswordAuthenticationToken authenticationToken = new UsernamePasswordAuthenticationToken(userDetails, null, userDetails.getAuthorities());
authenticationToken.setDetails(new WebAuthenticationDetailsSource().buildDetails(request));
SecurityContextHolder.getContext().setAuthentication(authenticationToken);
}
}
chain.doFilter(request, response);
}
}
```
4. 配置Spring Security
在Spring Security的配置类中,配置JWT认证过滤器。
``` java
@Configuration
@EnableWebSecurity
public class SecurityConfig extends WebSecurityConfigurerAdapter {
@Autowired
private JwtTokenUtil jwtTokenUtil;
@Override
protected void configure(HttpSecurity http) throws Exception {
http.csrf().disable()
.authorizeRequests()
.antMatchers("/api/authenticate").permitAll()
.anyRequest().authenticated()
.and()
.sessionManagement().sessionCreationPolicy(SessionCreationPolicy.STATELESS);
JwtAuthenticationFilter jwtAuthenticationFilter = new JwtAuthenticationFilter(jwtTokenUtil);
http.addFilterBefore(jwtAuthenticationFilter, UsernamePasswordAuthenticationFilter.class);
}
@Override
protected void configure(AuthenticationManagerBuilder auth) throws Exception {
auth.inMemoryAuthentication()
.withUser("admin")
.password("{noop}admin")
.roles("ADMIN");
}
}
```
5. 创建认证控制器
创建一个认证控制器,用于生成JWT令牌。
``` java
@RestController
@RequestMapping("/api")
public class AuthenticationController {
@Autowired
private JwtTokenUtil jwtTokenUtil;
@PostMapping("/authenticate")
public ResponseEntity<?> createAuthenticationToken(@RequestBody AuthenticationRequest authenticationRequest) throws Exception {
String username = authenticationRequest.getUsername();
String token = jwtTokenUtil.generateToken(username);
return ResponseEntity.ok(new AuthenticationResponse(token));
}
}
```
以上就是Spring Security整合JWT的代码实现。
阅读全文
相关推荐
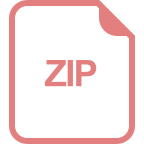
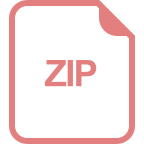
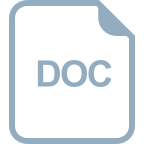





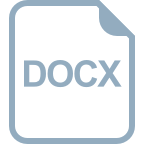
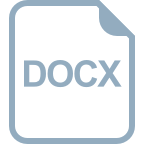
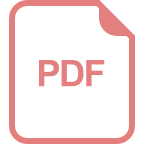
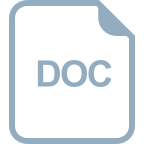
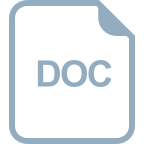



