postgis springboot 路径规划
时间: 2023-10-17 10:34:22 浏览: 113
PostGIS是一个开源的地理信息系统(GIS)扩展,可以与关系型数据库(如PostgreSQL)结合使用。它提供了许多用于空间数据处理和分析的功能。
在Spring Boot中使用PostGIS进行路径规划,你可以按以下步骤进行操作:
1. 首先,在你的Spring Boot项目中添加对PostGIS的依赖。你可以在`pom.xml`文件中添加以下依赖项:
```xml
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-data-jpa</artifactId>
</dependency>
<dependency>
<groupId>org.postgresql</groupId>
<artifactId>postgresql</artifactId>
<scope>runtime</scope>
</dependency>
<dependency>
<groupId>org.hibernate</groupId>
<artifactId>hibernate-spatial</artifactId>
</dependency>
```
2. 确保你的数据库已经安装并配置好了PostGIS扩展。你可以使用以下命令在你的数据库中创建PostGIS扩展:
```sql
CREATE EXTENSION postgis;
```
3. 创建一个实体类来表示你的空间数据。你可以使用`@Entity`注解和相应的字段注解来映射数据库表和字段,例如:
```java
import com.vividsolutions.jts.geom.Point;
@Entity
public class Location {
@Id
@GeneratedValue(strategy = GenerationType.IDENTITY)
private Long id;
private String name;
@Column(columnDefinition = "Geometry")
private Point coordinates;
// Getters and setters
}
```
4. 创建一个Spring Data JPA Repository接口来处理数据库操作。你可以继承`JpaRepository`接口,并且可以使用Spring Data JPA提供的查询方法,例如:
```java
import org.springframework.data.jpa.repository.JpaRepository;
public interface LocationRepository extends JpaRepository<Location, Long> {
List<Location> findByCoordinatesWithin(Point point, Double distance);
}
```
5. 在你的业务逻辑中使用路径规划功能。你可以使用Spring注入`LocationRepository`来访问数据库,并使用该接口提供的方法进行路径规划操作,例如:
```java
import org.springframework.beans.factory.annotation.Autowired;
@Service
public class RoutePlannerService {
@Autowired
private LocationRepository locationRepository;
public List<Location> findLocationsWithin(Point point, Double distance) {
return locationRepository.findByCoordinatesWithin(point, distance);
}
}
```
这样,你就可以在你的Spring Boot应用程序中使用PostGIS进行路径规划了。根据你的具体需求,你可能需要进一步调整和扩展这些代码。希望对你有所帮助!
阅读全文
相关推荐
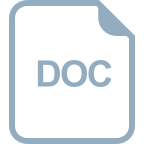
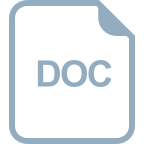
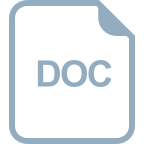
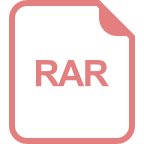
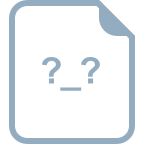
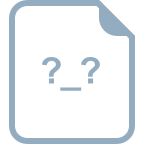





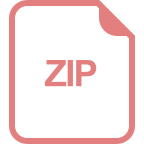






