已知字符串如何从文件中读入 c语言
时间: 2024-09-27 09:15:22 浏览: 43
在C语言中,从文件中读取字符串通常需要使用`fopen`、`fgets`函数以及适当的文件操作。以下是一个基本的步骤:
1. 首先,打开文件。你可以使用`fopen()`函数,它接受两个参数:文件名和模式(如"r"代表读取模式)。例如:
```c
FILE *file = fopen("filename.txt", "r");
```
如果文件无法打开,这会返回NULL。
2. 确保文件成功打开后,可以使用`fgets()`函数逐行读取数据。该函数每次最多读取一行(包括换行符),并存储到指定的字符数组中。例如:
```c
char str[100]; // 假设最大长度为99(包括终止符)
if (file != NULL) {
if (fgets(str, sizeof(str), file) == NULL) {
printf("Error reading from file.\n");
// 关闭文件
fclose(file);
} else {
// 字符串已读取,去掉可能存在的换行符
str[strlen(str)-1] = '\0'; // 如果字符串最后是换行符,移除它
}
}
```
3. 最后别忘了关闭文件,以释放资源:
```c
fclose(file);
```
相关问题
已知一段程序或字符串,例如:There are some books on the desk 1.该程序或字符串从文件读入; 2. 统计非空格字符个数和字符串个数(不包含子串) 统计结果存入另一个文件; 要求界面友好,程序风格规范。 c语言
在C语言中,你可以按照以下步骤编写一个小程序来完成这个任务:
1. **打开文件**:
首先,需要使用`fopen`函数打开输入文件(假设名为"input.txt")以及输出文件(假设名为"output.txt"),如果成功则返回文件指针,失败则处理错误。
```c
#include <stdio.h>
...
FILE *input_file = fopen("input.txt", "r");
FILE *output_file = fopen("output.txt", "w");
if (!input_file || !output_file) {
// 处理文件打开失败的情况
}
```
2. **读取并统计字符**:
使用`fscanf`读取文件内容,并用`getchar`逐个检查每个字符,非空格字符累加。同时,每遇到换行符就增加字符串计数。
```c
int non_space_count = 0;
int string_count = 1; // 初始化为1,因为第一个非空格字符会立即被记录
while (EOF != fgetc(input_file)) {
if (isspace(getc(input_file))) {
non_space_count++;
} else {
non_space_count++;
}
if (!isspace(getc(input_file))) { // 检查是否到下一个非空格字符
string_count++;
}
}
```
3. **关闭文件并写入结果**:
完成读取后,记得关闭文件,并将统计结果写入到输出文件。
```c
fclose(input_file);
fprintf(output_file, "Non-space characters: %d\nStrings: %d\n", non_space_count, string_count);
fclose(output_file);
```
4. **用户界面与规范**:
为了使程序更具可读性,可以添加适当的注释,使用标准的命名约定,并在主函数中提供命令行参数解析,让用户通过参数指定输入和输出文件名。
5. **整体结构**:
程序应该包括一个`main`函数,其中包含上述步骤,以及错误处理部分。
完整示例:
```c
#include <stdio.h>
int main(int argc, char *argv[]) {
// 检查参数数量
if (argc != 3) {
printf("Usage: %s input_file output_file\n", argv[0]);
return 1;
}
FILE *input_file = fopen(argv[1], "r");
FILE *output_file = fopen(argv[2], "w");
if (!input_file || !output_file) {
perror("Error opening file");
return 1;
}
int non_space_count = 0;
int string_count = 1;
while (EOF != fgetc(input_file)) {
if (isspace(getc(input_file))) {
non_space_count++;
} else {
non_space_count++;
}
if (!isspace(getc(input_file))) {
string_count++;
}
}
fclose(input_file);
fprintf(output_file, "Non-space characters: %d\nStrings: %d\n", non_space_count, string_count);
fclose(output_file);
return 0;
}
```
已知字符串str。字符串str包含字符’x’,’y’。 如果相邻的两个字符不同,消除两个字符,优先从左边进行消除。 xyyx - > yx ->,C语言
以下是使用C语言实现字符串消除的代码:
```
#include <stdio.h>
#include <string.h>
void eliminate(char* str) {
int len = strlen(str);
int i, j;
// 从左到右扫描字符串
for (i = 0; i < len - 1; i++) {
// 如果相邻两个字符不同,消除这两个字符
if (str[i] != str[i + 1]) {
// 从左边开始消除
for (j = i; j > 0; j--) {
str[j] = str[j - 1];
}
// 消除完成后将指针向右移动一位
i++;
len--;
}
}
}
int main() {
char str[100];
printf("请输入字符串:\n");
scanf("%s", str);
eliminate(str);
printf("消除后的字符串为:%s\n", str);
return 0;
}
```
该程序首先读入字符串,然后调用 `eliminate()` 函数对字符串进行消除。`eliminate()` 函数扫描字符串,如果相邻两个字符不同,就从左边开始消除这两个字符,直到消除完所有字符为止。最后输出消除后的字符串。
阅读全文
相关推荐
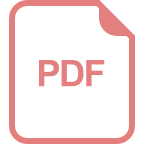
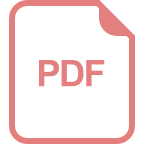
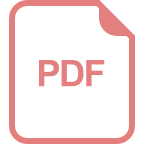
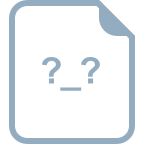
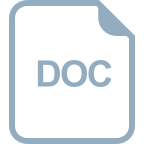
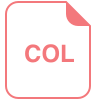











