在python中怎么找出列表中出现次数最多的数
时间: 2023-10-16 07:10:43 浏览: 42
在Python中,可以使用collections模块中的Counter函数来统计列表中每个元素出现的次数,并使用most_common()函数返回出现次数最多的元素和它的出现次数。具体实现如下:
```python
from collections import Counter
my_list = [1, 2, 3, 4, 5, 1, 2, 3, 1, 2, 1]
counter = Counter(my_list)
most_common = counter.most_common(1)
print(most_common[0][0])
```
输出结果为:
```
1
```
表示列表中出现次数最多的数是1。
相关问题
python怎么找出列表中出现次数最多的数
在 Python 中,可以使用 collections 模块中的 Counter 类来统计列表中每个元素出现的次数,然后使用 most_common() 方法找出出现次数最多的元素和它的出现次数。
下面是一个示例代码:
```python
from collections import Counter
my_list = [1, 2, 3, 4, 5, 1, 2, 3, 1, 2, 1]
counter = Counter(my_list)
most_common = counter.most_common(1)
print(most_common[0][0])
```
运行结果:
```
1
```
这个示例中,my_list 是一个包含了一些整数的列表,我们使用 Counter 类来统计每个元素出现的次数,然后使用 most_common() 方法找出出现次数最多的元素和它的出现次数。由于我们只需要找出出现次数最多的元素,因此将 most_common() 方法的参数设置为 1。最后,使用 print() 函数打印出出现次数最多的元素。
求整数序列中出现次数最多的数python
### 回答1:
可以使用Python中的Counter模块来实现统计整数序列中出现次数最多的数。
具体实现步骤如下:
1. 导入Counter模块
```python
from collections import Counter
```
2. 定义整数序列
```python
nums = [1, 2, 3, 4, 5, 1, 2, 3, 1, 2, 1]
```
3. 使用Counter模块统计整数序列中每个数出现的次数
```python
count = Counter(nums)
```
4. 找出出现次数最多的数
```python
most_common_num = count.most_common(1)[][]
```
完整代码如下:
```python
from collections import Counter
nums = [1, 2, 3, 4, 5, 1, 2, 3, 1, 2, 1]
count = Counter(nums)
most_common_num = count.most_common(1)[][]
print("出现次数最多的数是:", most_common_num)
```
输出结果为:
```
出现次数最多的数是: 1
```
### 回答2:
对于求一个整数序列中出现次数最多的数,可以使用Python中的Counter()函数来进行统计。Counter()函数是一个计数器对象,它可以帮助我们快速统计一个列表中每个元素出现的次数,并以字典的形式返回统计结果。
我们可以将整数序列作为Counter()函数的输入参数,然后使用most_common()方法获取出现次数最多的元素,它返回一个元素和计数器值的元组。最后,我们可以返回元素的值。
示例代码如下:
```python
from collections import Counter
def most_frequent(arr):
# 使用Counter函数统计序列中每个元素的出现次数
count = Counter(arr)
# 返回出现次数最多的元素
return count.most_common(1)[0][0]
# 测试
arr = [1, 2, 3, 3, 3, 4, 4, 5, 5, 5, 5]
print(most_frequent(arr)) # 输出 5
```
在这个示例中,我们定义了一个名为most_frequent()的函数,它接受一个整数序列作为输入参数。我们首先使用Counter()函数统计序列中每个元素的出现次数,并将结果存储在变量count中。然后,我们使用most_common()方法从计数器中获取出现次数最多的元素的列表,由于我们只需要获取列表中的第一个元素,所以使用[0][0]获取元素的值。
最后,我们使用示例数组进行函数测试,并将输出结果打印到控制台中,输出为5,也就是整数序列中出现次数最多的数。
### 回答3:
要求在整数序列中找到出现次数最多的数,我们可以采用Python中的字典来存储每个数字出现的次数。具体的实现步骤如下:
1. 输入整数序列
首先,我们需要让用户输入一个整数序列,可以使用input()函数获取用户输入的内容,并将字符串转换成整数列表。具体代码如下:
```
num_list = list(map(int, input().split()))
```
2. 统计数字出现次数
我们可以使用一个字典来统计每个数字的出现次数,其中字典的键是数字,值是该数字在序列中出现的次数。我们可以遍历整个序列,对于每个数字,如果字典中已经有该数字的键,则将该键对应的值加1;如果字典中还没有该数字的键,则将该数字作为新的键,并将值设为1。具体代码如下:
```
dict = {}
for num in num_list:
if num in dict:
dict[num] += 1
else:
dict[num] = 1
```
3. 找到出现次数最多的数字
我们可以遍历字典的值,找到出现次数最多的数字对应的键。具体代码如下:
```
max_num = max(dict, key=dict.get)
```
4. 输出结果
最后,我们可以输出出现次数最多的数字以及它出现的次数,具体代码如下:
```
print("出现次数最多的数字是:", max_num)
print("它出现的次数是:", dict[max_num])
```
完整代码如下所示:
```
num_list = list(map(int, input().split()))
dict = {}
for num in num_list:
if num in dict:
dict[num] += 1
else:
dict[num] = 1
max_num = max(dict, key=dict.get)
print("出现次数最多的数字是:", max_num)
print("它出现的次数是:", dict[max_num])
```
以上就是使用Python求整数序列中出现次数最多的数字的方法。
相关推荐
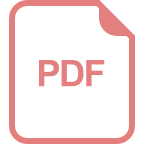
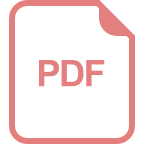
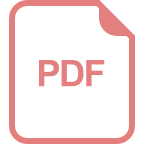












