一个查询页面中包括关键字、所属机构、证照类型、证照状态、颁发日期、成立批准日期、入库时间等字段,要怎么写一个后端代码从controller、service到mapper层查询数据
时间: 2024-09-23 19:05:34 浏览: 42
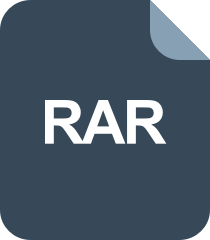
证照自助打印页面代码
在Java的Spring Boot框架下,我们可以按照以下步骤编写从Controller到Mapper的查询数据代码:
1. **Controller层**(`com.example.demo.Controllers.YourController.java`)
```java
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.web.bind.annotation.GetMapping;
import org.springframework.web.bind.annotation.RequestParam;
import com.example.demo.Services.YourService;
public class YourController {
private final YourService yourService;
@Autowired
public YourController(YourService yourService) {
this.yourService = yourService;
}
@GetMapping("/search")
public ResponseEntity<List<YourEntity>> searchData(
@RequestParam(name = "keyword", required = false) String keyword,
@RequestParam(name = "institution", required = false) String institution,
// ...其他参数
) {
try {
List<YourEntity> data = yourService.searchData(keyword, institution, /*...*/);
return new ResponseEntity<>(data, HttpStatus.OK);
} catch (Exception e) {
return new ResponseEntity<>(e.getMessage(), HttpStatus.INTERNAL_SERVER_ERROR);
}
}
}
```
2. **Service层** (`com.example.demo.Services.YourService.java`)
```java
import org.springframework.stereotype.Service;
import java.util.List;
import com.example.demo.Dtos.YourEntity;
import com.example.demo.Mappers.YourMapper;
@Service
public class YourService {
private final YourMapper yourMapper;
@Autowired
public YourService(YourMapper yourMapper) {
this.yourMapper = yourMapper;
}
public List<YourEntity> searchData(String keyword, String institution, /*...*/) {
List<YourEntity> entities = yourMapper.searchData(keyword, institution, /*...*/);
// 这里可能需要进一步处理返回的数据,如转换或过滤
return entities;
}
}
```
3. **Mapper层** (`com.example.demo.Mappers.YourMapper.java`, 使用MyBatis)
```java
import org.apache.ibatis.annotations.Param;
import java.util.List;
public interface YourMapper {
@Select("SELECT * FROM your_table WHERE 1=1")
List<YourEntity> searchData(
@Param("keyword") String keyword,
@Param("institution") String institution,
/* ...其他参数 */
);
}
```
在这个例子中,`your_table`是数据库表名,你需要根据实际数据库结构调整SQL查询。
阅读全文
相关推荐
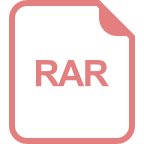
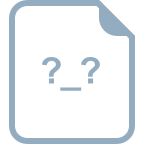















<!DOCTYPE html> <html lang="en"> <head> <meta charset="UTF-8"> <meta http-equiv="X-UA-Compatible" content="IE=edge"> <meta name="viewport" content="width=device-width, initial-scale=1.0"> <title>Document</title> <style> * { padding: 0; margin: 0; } li { list-style: none; } a { text-decoration: none; } .daohang { background-color: #333333; margin: 0 auto; overflow: hidden; position: relative; } .navigation { width: 1226px; height: 40px; margin-left: 208px; float: left; } .navigation>a{ line-height: 40px; font-size: 12px; color: #b0b0b0; } .navigation>span{ color: #424242; } .navigation>a:hover{ color: #fff; } .parent { position: relative; /* 父元素设置为相对定位 / } .child { position: absolute; / 子元素设置为绝对定位 / top: 100%; / 相对于父元素底部定位 / left: 0; display: none; / 初始状态下隐藏 / z-index: 1; / 提高层级,防止被其他元素遮挡 / } .parent:hover .child { display: block; / 鼠标悬停时显示下拉菜单 */ } </style> </head> <body> 小米官方 | 小米商店 | MIUI | loT | 云服务 | 天星科技 | 有品 | 小爱开放平台 | 企业团购 | 资质证照 | 协议规则 | 下载app
小米商城APP | Select Location </body> </html>为什么没有实现鼠标经过图片显现
