图书管理系统C语言数据结构
时间: 2023-12-13 19:05:14 浏览: 79
基于C语言数据结构的图书管理系统,可以采用动态链表来存储图书信息和用户信息。下面是一个简单的实现示例:
1. 定义图书信息结构体和用户信息结构体
```c
// 图书信息结构体
typedef struct book {
char name[50]; // 书名
char author[20]; // 作者
char publisher[30]; // 出版社
int year; // 出版年份
int stock; // 库存
struct book *next; // 指向下一本书的指针
} Book;
// 用户信息结构体
typedef struct user {
char username[20]; // 用户名
char password[20]; // 密码
int isAdmin; // 是否为管理员,0表示普通用户,1表示管理员
struct user *next; // 指向下一个用户的指针
} User;
```
2. 加载图书信息和用户信息
```c
// 从文件中加载图书信息
Book *loadBooks() {
Book *head = NULL, *tail = NULL;
FILE *fp = fopen("books.txt", "r");
if (fp == NULL) {
printf("Failed to open file: books.txt\n");
return NULL;
}
char line[200];
while (fgets(line, sizeof(line), fp)) {
Book *book = (Book *)malloc(sizeof(Book));
sscanf(line, "%s %s %s %d %d", book->name, book->author, book->publisher, &book->year, &book->stock);
book->next = NULL;
if (head == NULL) {
head = book;
} else {
tail->next = book;
}
tail = book;
}
fclose(fp);
return head;
}
// 从文件中加载用户信息
User *loadUsers() {
User *head = NULL, *tail = NULL;
FILE *fp = fopen("users.txt", "r");
if (fp == NULL) {
printf("Failed to open file: users.txt\n");
return NULL;
}
char line[200];
while (fgets(line, sizeof(line), fp)) {
User *user = (User *)malloc(sizeof(User));
sscanf(line, "%s %s %d", user->username, user->password, &user->isAdmin);
user->next = NULL;
if (head == NULL) {
head = user;
} else {
tail->next = user;
}
tail = user;
}
fclose(fp);
return head;
}
```
3. 登录功能
```c
// 登录
User *login(User *users) {
char username[20], password[20];
printf("Please enter your username: ");
scanf("%s", username);
printf("Please enter your password: ");
scanf("%s", password);
User *user = users;
while (user != NULL) {
if (strcmp(user->username, username) == 0 && strcmp(user->password, password) == 0) {
printf("Welcome, %s!\n", username);
return user;
}
user = user->next;
}
printf("Invalid username or password.\n");
return NULL;
}
```
4. 管理员功能
```c
// 添加图书
void addBook(Book **books) {
Book *book = (Book *)malloc(sizeof(Book));
printf("Please enter the book name: ");
scanf("%s", book->name);
printf("Please enter the author: ");
scanf("%s", book->author);
printf("Please enter the publisher: ");
scanf("%s", book->publisher);
printf("Please enter the year of publication: ");
scanf("%d", &book->year);
printf("Please enter the stock: ");
scanf("%d", &book->stock);
book->next = NULL;
if (*books == NULL) {
*books = book;
} else {
Book *tail = *books;
while (tail->next != NULL) {
tail = tail->next;
}
tail->next = book;
}
printf("Book added successfully.\n");
}
// 删除图书
void removeBook(Book **books) {
char name[50];
printf("Please enter the name of the book you want to remove: ");
scanf("%s", name);
Book *prev = NULL, *curr = *books;
while (curr != NULL) {
if (strcmp(curr->name, name) == 0) {
if (prev == NULL) {
*books = curr->next;
} else {
prev->next = curr->next;
}
free(curr);
printf("Book removed successfully.\n");
return;
}
prev = curr;
curr = curr->next;
}
printf("Book not found.\n");
}
// 修改图书信息
void modifyBook(Book *books) {
char name[50];
printf("Please enter the name of the book you want to modify: ");
scanf("%s", name);
Book *book = books;
while (book != NULL) {
if (strcmp(book->name, name) == 0) {
printf("Please enter the new author: ");
scanf("%s", book->author);
printf("Please enter the new publisher: ");
scanf("%s", book->publisher);
printf("Please enter the new year of publication: ");
scanf("%d", &book->year);
printf("Please enter the new stock: ");
scanf("%d", &book->stock);
printf("Book modified successfully.\n");
return;
}
book = book->next;
}
printf("Book not found.\n");
}
```
5. 普通用户功能
```c
// 查询图书
void searchBook(Book *books) {
char name[50];
printf("Please enter the name of the book you want to search: ");
scanf("%s", name);
Book *book = books;
while (book != NULL) {
if (strcmp(book->name, name) == 0) {
printf("Name: %s\n", book->name);
printf("Author: %s\n", book->author);
printf("Publisher: %s\n", book->publisher);
printf("Year of publication: %d\n", book->year);
printf("Stock: %d\n", book->stock);
return;
}
book = book->next;
}
printf("Book not found.\n");
}
```
6. 主函数
```c
int main() {
Book *books = loadBooks();
User *users = loadUsers();
printf("Welcome to the library!\n");
printf(" __ __ _ _ _ _ \n");
printf(" | \\/ | __ _(_) |_| | ___| |_ \n");
printf(" | |\\/| |/ _` | | __| |/ _ \\ __|\n");
printf(" | | | | (_| | | |_| | __/ |_ \n");
printf(" |_| |_|\\__,_|_|\\__|_|\\___|\\__|\n");
printf("\n");
User *user = login(users);
while (user == NULL) {
user = login(users);
}
if (user->isAdmin) {
printf("Welcome, admin!\n");
int choice;
do {
printf("Please select an option:\n");
printf("1. Add book\n");
printf("2. Remove book\n");
printf("3. Modify book information\n");
printf("4. Exit\n");
scanf("%d", &choice);
switch (choice) {
case 1:
addBook(&books);
break;
case 2:
removeBook(&books);
break;
case 3:
modifyBook(books);
break;
case 4:
break;
default:
printf("Invalid choice.\n");
break;
}
} while (choice != 4);
} else {
printf("Welcome, user!\n");
int choice;
do {
printf("Please select an option:\n");
printf("1. Search book\n");
printf("2. Exit\n");
scanf("%d", &choice);
switch (choice) {
case 1:
searchBook(books);
break;
case 2:
break;
default:
printf("Invalid choice.\n");
break;
}
} while (choice != 2);
}
return 0;
}
```
阅读全文
相关推荐
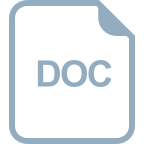
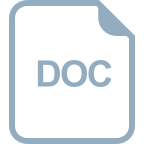
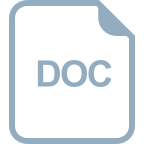

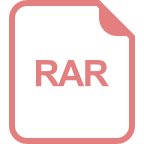





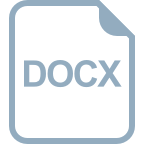
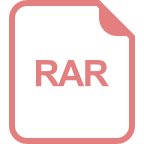
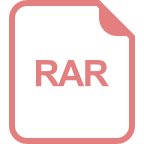
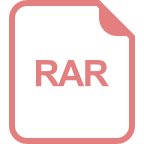
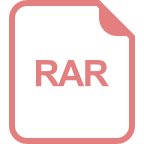
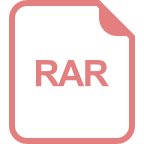

